Code Organization in Dart: Structuring Your Projects
When embarking on a new Dart project, whether it’s a small app or a complex software solution, proper code organization is crucial. A well-structured project not only enhances code readability and maintainability but also facilitates collaboration among team members. In this article, we will delve into the best practices for organizing your Dart projects, covering key principles, directory structures, and providing code samples along the way.
Table of Contents
1. Why Code Organization Matters
Before we dive into the specifics, let’s understand why code organization is essential. Imagine a large project with hundreds of files, each containing numerous lines of code. Without a logical structure, navigating through this codebase becomes a nightmare. Code organization:
- Improves Readability: Well-organized code is easier to understand, reducing the time developers spend deciphering its logic and purpose.
- Enhances Maintenance: When changes or updates are needed, a clear organization minimizes the chances of introducing bugs and makes debugging more efficient.
- Enables Collaboration: A structured project facilitates collaboration by allowing team members to work on different parts of the codebase without stepping on each other’s toes.
2. Key Principles of Code Organization
Before we delve into directory structures, let’s establish some key principles that guide effective code organization in Dart projects.
2.1. Single Responsibility Principle (SRP)
Each file or module should have a single responsibility. This means that a file should address a specific concern or functionality. This not only makes your codebase more modular but also helps in isolating bugs and easing future changes.
2.2. Separation of Concerns (SoC)
Related to SRP, SoC dictates that different concerns (like UI, business logic, and data handling) should be separated. This allows for better maintainability and enables changes in one part of the application without affecting others.
2.3. Modularity and Reusability
Create reusable modules or components that can be easily integrated into different parts of your application. This reduces redundancy and promotes consistency across your project.
2.4. Consistent Naming Conventions
Establish a consistent naming convention for files, classes, functions, and variables. This makes it easier to locate and understand code elements.
2.5. Layered Architecture
Divide your application into distinct layers, such as presentation, business logic, and data access. This architectural approach improves code organization and scalability.
3. Directory Structure
Now that we’ve covered the foundational principles, let’s explore a recommended directory structure for organizing your Dart projects.
plaintext my_dart_project/ ?? lib/ ? ?? models/ ? ?? services/ ? ?? ui/ ? ? ?? screens/ ? ? ?? widgets/ ? ?? main.dart ?? test/ ?? pubspec.yaml
3.1. lib Directory
The lib directory is where the bulk of your Dart code resides. Here’s a breakdown of the subdirectories within it:
- models: Store your data models here. These are classes that define the structure of your data entities.
- services: Place service classes here. Services encapsulate specific functionalities (e.g., API calls, data storage) that can be reused across your application.
- ui: This directory contains user interface-related code. Inside ui, you can further categorize your code:
- screens: Each screen of your application gets its own folder. This folder contains the screen’s UI components and associated logic.
- widgets: Reusable UI components that are shared across different screens or sections of your app belong here.
3.2. test Directory
The test directory is where you write unit tests for your Dart code. Structuring your tests similarly to your codebase can help maintain consistency and ease testing.
3.3. pubspec.yaml
The pubspec.yaml file is where you manage your project’s dependencies. This file ensures that the necessary packages are installed to support your project’s functionality.
4. Sample Code Organization
Let’s put theory into practice with a simple Dart project to illustrate how code organization works in real life.
Suppose we’re building a To-Do List application. Here’s a sample directory structure:
plaintext todo_app/ ?? lib/ ? ?? models/ ? ? ?? task.dart ? ? ?? category.dart ? ?? services/ ? ? ?? database.dart ? ? ?? task_service.dart ? ?? ui/ ? ? ?? screens/ ? ? ? ?? home/ ? ? ? ? ?? home_screen.dart ? ? ? ? ?? home_screen_logic.dart ? ? ? ?? add_task/ ? ? ? ?? add_task_screen.dart ? ? ? ?? add_task_screen_logic.dart ? ? ?? widgets/ ? ? ?? task_item.dart ? ? ?? category_selector.dart ? ?? main.dart ?? test/ ? ?? models/ ? ? ?? task_test.dart ? ? ?? category_test.dart ? ?? services/ ? ?? task_service_test.dart ?? pubspec.yaml
In this sample project structure:
- The models directory holds the Task and Category classes, defining the structure of our data entities.
- The services directory contains the database-related code and the TaskService class responsible for managing tasks.
- The ui/screens directory houses the main screens of the app, such as the HomeScreen and AddTaskScreen.
- The ui/widgets directory contains reusable UI components like TaskItem and CategorySelector.
Conclusion
Code organization is a vital aspect of any Dart project, impacting readability, maintainability, and collaboration. By following key principles like SRP, SoC, modularity, and consistent naming conventions, along with a well-defined directory structure, you can create a project that’s easier to understand, maintain, and scale. Remember, a little effort in organizing your code at the beginning can save a significant amount of time and frustration in the long run. Happy coding!
Table of Contents
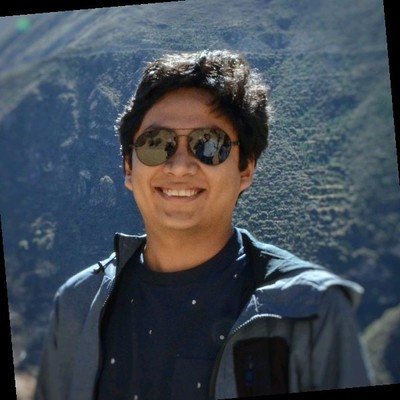
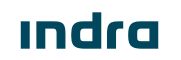