Mastering Dart: A Comprehensive Guide for Programmers
Are you a programmer looking to master Dart? Look no further! In this comprehensive guide, we will take you on a journey through the world of Dart programming. Whether you’re a beginner or an experienced developer, this tutorial will equip you with the knowledge and skills needed to become a Dart expert. So, let’s get started!
What is Dart?
Dart is a powerful, object-oriented programming language developed by Google. It offers a wide range of features, including a concise syntax, strong typing, and excellent support for both frontend and backend development. Dart is widely used for building web and mobile applications, as well as server-side applications using frameworks like Flutter and AngularDart.
Setting Up the Development Environment
To start coding in Dart, you’ll need to set up your development environment. This section will guide you through the installation process and provide step-by-step instructions for configuring Dart SDK and IDEs such as Visual Studio Code or IntelliJ IDEA.
Dart Syntax Fundamentals
Before diving into advanced topics, it’s crucial to understand the basics of Dart syntax. In this section, we’ll cover variables, data types, operators, control structures, and more. We’ll also explore concepts like type inference and optional typing, which make Dart a flexible and developer-friendly language.
Code Sample 1: Hello World in Dart
csharp void main() { print('Hello, Dart!'); }
Variables and Data Types
Variables are essential in any programming language. In Dart, you have various data types at your disposal, such as numbers, strings, booleans, lists, maps, and more. This section will teach you how to declare and use variables effectively in Dart, along with type annotations and type inference.
Code Sample 2: Variable Declaration and Usage
arduino int age = 25; String name = 'John Doe'; bool isStudent = true; print('Name: $name, Age: $age, Is Student: $isStudent');
Control Flow and Looping
Control flow and looping structures allow you to make decisions and iterate over data in your programs. In this section, we’ll cover if-else statements, switch statements, loops like for, while, and do-while, and more. You’ll learn how to control the flow of your Dart code effectively.
Code Sample 3: For Loop in Dart
css for (int i = 1; i <= 5; i++) { print('Iteration $i'); }
Functions and Lambdas
Functions are the building blocks of modular and reusable code. Dart provides various ways to define and use functions, including named functions, anonymous functions, and arrow functions (lambdas). This section will cover function declarations, parameters, return types, and how to work with higher-order functions.
Code Sample 4: Function Declaration and Usage
csharp int add(int a, int b) { return a + b; } print(add(2, 3)); // Output: 5
Object-Oriented Programming with Dart
Dart supports object-oriented programming (OOP) principles like classes, objects, inheritance, and polymorphism. In this section, we’ll explore how to create classes, define properties and methods, implement interfaces, and leverage the power of OOP to build modular and scalable applications.
Code Sample 5: Class and Object in Dart
arduino class Person { String name; int age; void introduce() { print('Hello, my name is $name. I am $age years old.'); } } Person person = Person(); person.name = 'John Doe'; person.age = 25; person.introduce();
Error Handling and Exception Handling
Errors and exceptions are part of every programming language. Dart provides robust mechanisms for handling errors and exceptions, including try-catch blocks, throw statements, and custom exception classes. This section will guide you through error handling techniques to ensure the reliability of your Dart code.
Code Sample 6: Exception Handling in Dart
php try { // Code that may throw an exception } catch (e) { // Handle the exception print('An error occurred: $e'); }
Dart Libraries and Packages
Dart offers a rich ecosystem of libraries and packages to extend its functionality. In this section, we’ll explore how to import and use libraries, as well as introduce popular packages for common tasks like HTTP requests, JSON parsing, and more. You’ll learn how to leverage the power of existing libraries to enhance your Dart applications.
Asynchronous Programming with Dart
Asynchronous programming is crucial for building responsive and efficient applications. Dart provides powerful features like async/await and futures to handle asynchronous tasks. In this section, we’ll dive into asynchronous programming, covering topics like async functions, futures, error handling, and working with multiple asynchronous operations.
Code Sample 7: Asynchronous Function in Dart
csharp Future<String> fetchData() async { // Simulate network request delay await Future.delayed(Duration(seconds: 2)); return 'Data fetched successfully!'; } void main() async { String data = await fetchData(); print(data); }
Unit Testing in Dart
Unit testing is an essential part of software development. Dart includes a powerful testing framework called “test” for writing unit tests. This section will introduce you to unit testing in Dart, covering test setup, writing test cases, assertions, and running tests using popular testing tools.
Dart Best Practices and Tips
To become a proficient Dart programmer, it’s essential to follow best practices and utilize advanced techniques. In this section, we’ll share some useful tips, coding conventions, and performance optimization techniques that will enhance your Dart code quality and productivity.
Conclusion
Congratulations! You have reached the end of our comprehensive guide to mastering Dart programming. We have covered the fundamentals, advanced topics, and best practices to help you become a Dart expert. Remember, practice is key to mastering any programming language. So, keep coding, experimenting, and building amazing applications with Dart. Happy coding!
Whether you’re a beginner or an experienced programmer, mastering Dart can open up exciting opportunities in web and mobile development. Dive into the world of Dart programming with our comprehensive guide, complete with code samples and step-by-step instructions.
Table of Contents
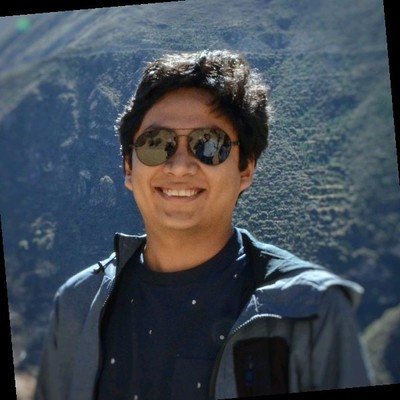
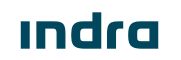