Concurrency in Dart: Harnessing the Power of Isolates
Concurrency is a crucial aspect of modern software development, allowing programs to perform multiple tasks simultaneously, thereby improving performance and responsiveness. Dart, a versatile and popular programming language, offers powerful concurrency support through isolates. Isolates are lightweight, independent threads that enable parallel execution without sharing memory, making them an excellent choice for concurrent programming in Dart.
In this blog, we will explore the fundamentals of isolates, their benefits, and how to utilize them effectively to boost the performance of your Dart applications.
What are Isolates?
Dart runs on a single-threaded event loop, making it challenging to execute multiple tasks concurrently without impacting performance. Isolates address this limitation by providing a mechanism for running code in separate threads, each with its own memory space, allowing true parallel execution.
Unlike threads, isolates do not share memory, ensuring that one isolate’s actions do not interfere with another’s. Communication between isolates is achieved through messages, enabling safe and efficient data exchange.
The Advantages of Isolates
- Improved Performance: By utilizing multiple isolates, applications can execute tasks in parallel, reducing overall processing time and increasing performance. This is especially beneficial for computationally intensive operations.
- Enhanced Scalability: Isolates enable horizontal scaling, allowing applications to leverage multi-core processors effectively. As your hardware evolves, the application can easily take advantage of the additional computing power without significant code changes.
- Robustness: Isolates are isolated from each other, meaning that errors or crashes in one isolate do not affect others. This isolation ensures that critical application components can continue running, enhancing overall application robustness.
- Responsive User Interface: Isolates can be used to offload resource-intensive computations from the main thread, keeping the user interface responsive and smooth even during complex tasks.
- Shared-State Protection: With isolates, the risk of race conditions and shared-state bugs is minimized since each isolate has its own memory space.
Creating Isolates
In Dart, creating an isolate is a straightforward process using the Isolate.spawn function. Let’s take a look at a simple example where we create an isolate to perform a time-consuming task, factorial computation:
dart import 'dart:isolate'; void isolateEntry(SendPort sendPort) { int result = 1; final int number = 10; for (int i = 1; i <= number; i++) { result *= i; } sendPort.send(result); } void main() { ReceivePort receivePort = ReceivePort(); Isolate.spawn(isolateEntry, receivePort.sendPort); receivePort.listen((message) { print("Factorial result: ${message}"); receivePort.close(); }); }
In this example, we define the isolateEntry function, which calculates the factorial of a given number. The Isolate.spawn function takes two arguments: the entry point function (isolateEntry in our case) and a SendPort to allow communication between the main isolate and the newly created isolate. When the computation is complete, the result is sent back to the main isolate through the SendPort.
Passing Messages between Isolates
Isolates communicate by passing messages through ports. Dart provides two types of ports for communication: SendPort and ReceivePort. The SendPort is used to send messages from one isolate to another, while the ReceivePort is used to receive messages.
Let’s extend our previous example to calculate the factorial of multiple numbers concurrently using isolates:
dart import 'dart:isolate'; void isolateEntry(SendPort sendPort) { ReceivePort receivePort = ReceivePort(); sendPort.send(receivePort.sendPort); receivePort.listen((message) { int number = message; int result = 1; for (int i = 1; i <= number; i++) { result *= i; } sendPort.send(result); receivePort.close(); }); } void main() { int number1 = 10; int number2 = 5; Isolate.spawn(isolateEntry, ReceivePort().sendPort).then((isolate1) { Isolate.spawn(isolateEntry, ReceivePort().sendPort).then((isolate2) { ReceivePort receivePort = ReceivePort(); isolate1.sendPort.send(number1); isolate2.sendPort.send(number2); int factorial1, factorial2; int responsesReceived = 0; receivePort.listen((message) { if (responsesReceived == 0) { factorial1 = message; } else { factorial2 = message; print("Factorial of ${number1}: ${factorial1}"); print("Factorial of ${number2}: ${factorial2}"); receivePort.close(); } responsesReceived++; }); }); }); }
In this example, we spawn two isolates to calculate the factorials of number1 and number2 concurrently. The isolateEntry function now takes the number to calculate the factorial as the message and sends the result back using the SendPort. The main isolate sends the numbers to the spawned isolates and listens for the results using a ReceivePort.
Efficiently Managing Isolates
While isolates provide significant advantages, managing them efficiently is crucial for optimal performance and resource utilization. Here are some essential tips for managing isolates effectively:
- Reuse Isolates: Creating isolates is a resource-intensive task. Whenever possible, reuse isolates for similar computations rather than creating new ones. Implement a pool of isolates to manage their lifecycle efficiently.
- Use Isolate Groups: Dart provides the concept of isolate groups, allowing you to spawn multiple isolates within the same group. This feature is helpful when you want to terminate a group of isolates together or share resources within a group.
- Isolate Supervision: Monitor the health of isolates and restart them if they crash unexpectedly. Isolate supervision ensures that your application remains robust and resilient to isolate failures.
- Message Size: Be mindful of the size of messages being passed between isolates. Large messages can cause performance bottlenecks and increase memory consumption. If you need to send large amounts of data, consider serializing and deserializing it.
Conclusion
Concurrency is a critical aspect of modern application development, and Dart’s isolates provide a powerful solution for harnessing concurrent capabilities. By leveraging isolates, you can improve your Dart applications’ performance, responsiveness, and scalability.
In this blog, we explored the fundamentals of isolates, their advantages, and how to create and manage them efficiently. Now, armed with this knowledge, you can confidently incorporate isolates into your Dart projects and unlock the true potential of concurrency.
Remember to carefully manage your isolates, reuse them whenever possible, and supervise them for a robust and high-performance concurrent application. Happy coding!
Table of Contents
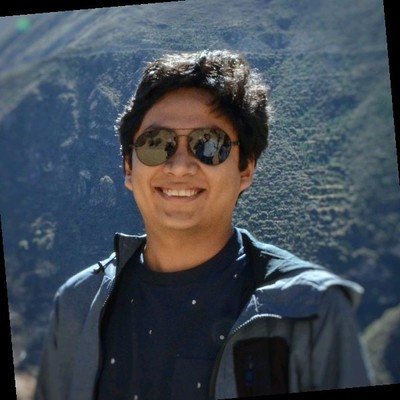
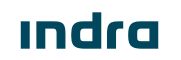