Dart for Web Development: Creating Dynamic and Responsive Websites
In today’s digital era, having a dynamic and responsive website is essential to provide an exceptional user experience. While there are several programming languages available for web development, Dart stands out as a powerful language designed specifically for front-end development. With its modern features and robust ecosystem, Dart offers developers a streamlined and efficient way to create interactive and responsive websites.
In this blog post, we will dive into the world of Dart for web development. We’ll explore the key features of the language, its benefits, and how it enables developers to build dynamic and responsive websites. So, let’s get started!
1. What is Dart?
Dart is a general-purpose, class-based programming language developed by Google. Initially introduced as an alternative to JavaScript, Dart has evolved into a versatile language that excels in web and mobile app development. Dart compiles to efficient JavaScript code, making it compatible with all modern web browsers.
2. Key Features of Dart
Dart comes with a rich set of features that make it an excellent choice for web development. Some of the key features include:
- Strong Typing: Dart is a statically typed language, which helps catch errors at compile time and ensures better code quality.
- Just-In-Time (JIT) Compilation: Dart supports JIT compilation, enabling developers to write, compile, and run code dynamically during development, leading to faster iteration cycles.
- Ahead-of-Time (AOT) Compilation: For production deployment, Dart can be compiled to efficient JavaScript code using AOT compilation, resulting in optimized performance.
- Garbage Collection: Dart has built-in garbage collection, relieving developers from memory management tasks.
- Asynchronous Programming: Dart provides excellent support for asynchronous programming with its async and await keywords, making it easy to handle complex operations without blocking the user interface.
3. Building Dynamic Websites with Dart
3.1. Language Basics
Let’s start by looking at some code examples to understand the basics of Dart:
dart void main() { String message = 'Hello, Dart!'; print(message); }
In the above code, we define a function main() that prints a message to the console. Dart’s syntax is clean and familiar, making it easy for developers to transition from other programming languages.
3.2. DOM Manipulation
Dart enables seamless manipulation of the Document Object Model (DOM), allowing developers to create dynamic web applications. Here’s an example of how Dart can modify an HTML element:
dart import 'dart:html'; void main() { ButtonElement button = querySelector('#myButton'); button.onClick.listen((event) { button.text = 'Clicked!'; }); }
In the above code snippet, we import the dart:html library, which provides access to the DOM APIs. We select a button element with the ID myButton and attach an event listener to change the button’s text when it’s clicked.
3.3. Asynchronous Programming
With Dart’s async/await syntax, handling asynchronous operations becomes straightforward. Here’s an example of fetching data from an API:
dart import 'dart:convert'; import 'package:http/http.dart' as http; void main() async { var response = await http.get(Uri.parse('https://api.example.com/data')); var data = jsonDecode(response.body); print(data); }
In this example, we use the http package to make an HTTP request and jsonDecode to parse the response. The await keyword allows us to pause the execution until the response is received.
4. Creating Responsive Websites with Dart
4.1. Dart UI Libraries
Dart provides several UI libraries that help in creating responsive web applications. The dart:ui library allows direct access to low-level rendering APIs, while packages like flutter_web and flutter_styled bring Flutter’s rich UI capabilities to the web.
4.2. Media Queries
Media queries are an essential part of responsive web design, and Dart offers built-in support for them. Here’s an example of using media queries in Dart:
dart import 'dart:html'; void main() { window.onResize.listen((event) { if (window.matchMedia('(max-width: 600px)').matches) { print('Mobile view'); } else { print('Desktop view'); } }); }
In the above code, we listen to the onResize event and check the window width using a media query. Based on the width, we can apply different styles or perform specific actions.
4.3. Handling Device Orientation
Dart also allows developers to handle device orientation changes easily. Here’s an example:
dart import 'dart:html'; void main() { window.onDeviceOrientation.listen((event) { print('Orientation changed to: ${event.alpha}, ${event.beta}, ${event.gamma}'); }); }
In this code snippet, we listen to the onDeviceOrientation event and log the device’s orientation data whenever it changes.
5. Dart and Web Frameworks
5.1 AngularDart
AngularDart is a powerful web application framework that leverages Dart’s capabilities to build large-scale, maintainable applications. It provides features like dependency injection, declarative templates, and two-way data binding.
5.2 Flutter for Web
Flutter, a popular cross-platform UI toolkit, can also be used for web development using the Flutter for Web project. With Flutter, developers can build highly responsive and visually stunning web applications using a single codebase.
6. Dart Tools and Ecosystem
6.1 Package Manager
Dart comes with its package manager called Pub, which allows developers to manage dependencies efficiently. Pub hosts a vast collection of libraries and packages that cover a wide range of functionality, making it easy to extend the capabilities of your Dart projects.
6.2 Testing Frameworks
Dart provides testing frameworks like test and flutter_test for unit testing and widget testing, respectively. These frameworks offer a comprehensive set of tools to ensure the quality and reliability of your web applications.
6.3 Code Editors and IDEs
Dart is well-supported by popular code editors and IDEs such as Visual Studio Code, IntelliJ IDEA, and Android Studio. These tools offer features like code completion, debugging, and refactoring, enhancing the developer experience.
Conclusion
Dart has emerged as a powerful language for building dynamic and responsive websites. With its clean syntax, strong typing, and extensive ecosystem, Dart enables developers to create modern web applications with ease. Whether you’re working on a small project or a large-scale application, Dart provides the tools and libraries you need to deliver exceptional user experiences. Start exploring Dart for web development, and unlock a whole new world of possibilities!
Table of Contents
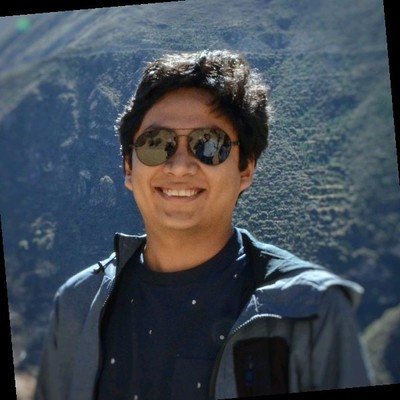
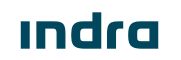