Debugging and Testing Dart Applications: Best Practices
In the world of software development, debugging and testing are essential processes that ensure the reliability and quality of your applications. Dart, a versatile programming language, is no exception. Whether you’re building web applications, mobile apps, or command-line tools with Dart, implementing effective debugging and testing practices is crucial to identifying and resolving issues early in the development lifecycle. In this guide, we’ll delve into the best practices for debugging and testing Dart applications, covering essential techniques, tools, and strategies.
Table of Contents
1. Understanding Debugging and Testing
1.1. The Importance of Debugging and Testing
Debugging and testing are crucial steps in software development that help identify and fix issues in your codebase. Debugging involves locating and correcting errors (or “bugs”) in your code, ensuring that it behaves as expected. Testing, on the other hand, involves systematically assessing various aspects of your application to validate its functionality and performance.
Effective debugging and testing lead to the following benefits:
- Improved Software Quality: Catching and addressing issues early in development results in a more stable and reliable application.
- Time and Cost Savings: Fixing bugs and issues before deployment reduces the time and resources spent on post-deployment problem-solving.
- Enhanced User Experience: Thorough testing minimizes the likelihood of encountering unexpected behavior, resulting in a smoother user experience.
1.2. Types of Bugs and Errors
Bugs and errors can manifest in various forms, making it crucial to understand their types to effectively address them.
- Syntax Errors: These are caused by violating the rules of the programming language. Dart’s strong typing system often catches syntax errors during compilation.
dart var x = 10; print(x // Missing closing parenthesis
- Logic Errors: These occur when the program runs without errors but produces incorrect results due to flawed logic.
dart int calculateSum(int a, int b) { return a - b; // Should be a + b }
- Runtime Errors: These errors occur during program execution, often due to unexpected conditions like division by zero or accessing null values.
dart void main() { int result = 10 ~/ 0; // Division by zero print(result); }
2. Debugging Dart Applications
Debugging Dart applications involves identifying and rectifying bugs to ensure the proper functioning of your code. Here are some effective debugging techniques and tools:
2.1. Utilizing print() Statements
One of the simplest yet effective ways to debug your Dart code is by using print() statements. By strategically placing print() statements at key points in your code, you can output variable values and messages to the console, helping you understand the flow and behavior of your application.
dart void main() { int x = 5; int y = 3; print('Before calculation'); int sum = x + y; print('After calculation: $sum'); }
2.2. Leveraging Logging Libraries
Logging libraries provide more advanced debugging capabilities compared to print() statements. Libraries like logging enable you to define different logging levels, categorize messages, and direct logs to various outputs (e.g., console, files).
dart import 'package:logging/logging.dart'; void main() { Logger.root.level = Level.ALL; Logger.root.onRecord.listen((record) { print('${record.level.name}: ${record.time}: ${record.message}'); }); final logger = Logger('myApp'); logger.info('Initializing application...'); }
2.3. Using DevTools for Web Applications
For Dart web applications, the Dart DevTools suite offers powerful debugging and profiling capabilities. It provides insights into the behavior of your application, performance bottlenecks, and network activity.
To launch DevTools, run the following command:
bash dartdevc --enable-vm-service web/main.dart
Then, access DevTools by navigating to http://127.0.0.1:9100 in your web browser.
2.4. Integrated Development Environments (IDEs)
IDEs such as Visual Studio Code and IntelliJ IDEA offer integrated debugging tools for Dart applications. You can set breakpoints, step through code, inspect variables, and analyze call stacks to pinpoint and resolve issues effectively.
3. Testing Dart Applications
Testing is a systematic approach to validate that your code behaves as expected under different conditions. Dart provides various testing frameworks and tools to ensure the reliability of your applications.
3.1. Writing Unit Tests
Unit tests focus on testing individual units of code in isolation, such as functions and methods. The test package is widely used for writing unit tests in Dart.
dart import 'package:test/test.dart'; int add(int a, int b) { return a + b; } void main() { test('Addition', () { expect(add(2, 3), equals(5)); }); }
3.2. Creating Integration Tests
Integration tests evaluate the interactions between different components or modules of your application. The test package also supports writing integration tests.
dart import 'package:test/test.dart'; import 'package:myapp/calculator.dart'; void main() { test('Calculator Addition', () { Calculator calc = Calculator(); expect(calc.add(2, 3), equals(5)); }); }
3.3. Implementing Widget Tests for Flutter Apps
For Flutter applications, widget tests verify the behavior of individual widgets. The flutter_test package provides tools for writing widget tests.
dart import 'package:flutter_test/flutter_test.dart'; import 'package:myapp/widgets/my_button.dart'; void main() { testWidgets('Button Tap', (WidgetTester tester) async { await tester.pumpWidget(MyButton(text: 'Click me')); await tester.tap(find.text('Click me')); await tester.pump(); expect(find.text('Button tapped!'), findsOneWidget); }); }
3.4. Test-Driven Development (TDD) Approach
Test-Driven Development (TDD) is a methodology where you write tests before writing the actual code. This approach helps you define clear requirements and ensures that your code meets those requirements.
The TDD cycle generally involves the following steps:
- Write a failing test for the desired functionality.
- Write the minimum code required to make the test pass.
- Refactor the code while keeping the test passing.
4. Essential Best Practices
4.1. Keeping Tests Independent and Isolated
Each test should be independent of others, and the order of test execution should not affect the results. Isolated tests prevent false positives and make troubleshooting easier.
4.2. Providing Meaningful Test Cases and Descriptions
Use descriptive test names and comments to clearly communicate the purpose of each test. This helps in understanding the intent of the test and its expected outcomes.
4.3. Regularly Running Tests
Integrate tests into your development workflow by running them frequently. Automate test execution to catch issues early and ensure that your codebase remains stable.
4.4. Continuous Integration and Automated Testing
Leverage Continuous Integration (CI) tools like Jenkins, Travis CI, or GitHub Actions to automatically build and test your code whenever changes are pushed. This ensures consistent testing across different environments and reduces the risk of deployment failures.
Conclusion
In conclusion, effective debugging and testing are essential for maintaining the quality and reliability of your Dart applications. By understanding the types of bugs, employing debugging techniques, and following testing best practices, you can build robust applications that provide a seamless experience for users. Incorporating these practices early in your development process can save time, reduce costs, and lead to a more successful software release. So, whether you’re developing web applications, mobile apps, or other Dart-powered solutions, prioritize debugging and testing to ensure your code is solid and dependable.
Table of Contents
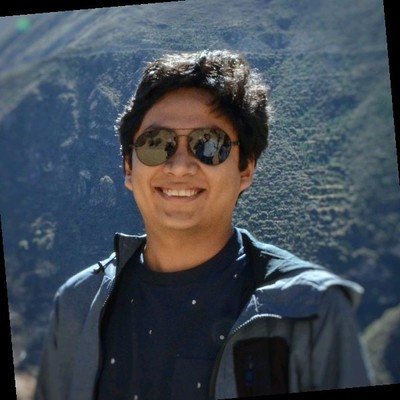
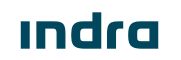