Exploring the Power of Dart: A Deep Dive into Its Features
In the world of modern programming languages, Dart has been gaining significant attention. Developed by Google, Dart is a versatile language that is designed to build high-performance web, mobile, and desktop applications. It combines the best of both worlds, offering the simplicity of JavaScript with the performance of languages like Java and C++. In this blog, we’ll take a deep dive into the power of Dart, exploring its key features and showcasing code samples to illustrate its capabilities.
Strong Typing and Type Inference
One of the key strengths of Dart is its strong typing system, which promotes code reliability and early error detection. Dart supports both static and dynamic typing, giving developers flexibility in choosing the appropriate approach for their projects. With static typing, variables are explicitly declared with their types, allowing for better code readability and easier refactoring. Dart also supports type inference, which automatically infers the types based on context, reducing the need for explicit type declarations.
Code Sample:
dart void main() { String message = "Hello, Dart!"; int count = 42; print(message); print(count); }
In the above code sample, the variables message and count are explicitly declared with their respective types. The print function is used to display the values of these variables. This demonstrates the strong typing feature of Dart, ensuring that variables are used in a type-safe manner.
Asynchronous Programming with Futures and Streams
Asynchronous programming is crucial for building responsive and efficient applications. Dart provides built-in support for asynchronous programming through Futures and Streams. Futures represent a single asynchronous computation that may complete with a value or an error. They allow developers to handle operations that take time, such as reading from a file or making an API request, without blocking the execution of the program.
Code Sample:
dart import 'dart:async'; Future<String> fetchData() { return Future.delayed(Duration(seconds: 2), () => "Data Fetched!"); } void main() { print("Fetching data..."); fetchData().then((data) { print(data); }); print("Program continues..."); }
In the above code sample, the fetchData function simulates a delay of 2 seconds before returning the fetched data. The then method is used to handle the completion of the Future and print the fetched data. This allows the program to continue executing other tasks without waiting for the asynchronous operation to complete.
Streams, on the other hand, represent a sequence of asynchronous events. They are useful for handling continuous data streams, such as user input or real-time data updates. Dart’s stream API provides powerful features for manipulating and transforming data streams, enabling developers to build reactive and event-driven applications.
Object-Oriented Programming in Dart
Dart is an object-oriented language, and it fully embraces the principles of object-oriented programming (OOP). It provides classes, objects, inheritance, and other OOP concepts to help structure and organize code. Dart’s class-based approach promotes code reusability and modularity, making it easier to build and maintain complex applications.
Code Sample:
dart class Animal { String name; int age; Animal(this.name, this.age); void eat() { print("$name is eating."); } } class Cat extends Animal { String color; Cat(String name, int age, this.color) : super(name, age); void meow() { print("$name is meowing."); } } void main() { var cat = Cat("Whiskers", 3, "Gray"); cat.eat(); cat.meow(); }
In the above code sample, the Animal class represents a generic animal with a name and age. The Cat class extends the Animal class and adds an additional property color. The Cat class also defines a custom method meow. In the main function, an instance of the Cat class is created, and its inherited and custom methods are called.
AOT and JIT Compilation
Dart offers two compilation modes: Ahead-of-Time (AOT) and Just-in-Time (JIT). The AOT compilation produces highly optimized native code that can be executed directly on the target platform, resulting in faster startup times and improved performance. This makes Dart suitable for building standalone applications, such as mobile and desktop apps.
On the other hand, the JIT compilation allows for a faster development cycle by providing hot-reload capabilities. It compiles the Dart code to a highly optimized intermediate representation (IR), which is then executed by the Dart Virtual Machine (VM). This enables developers to make changes to their code and see the results immediately without restarting the application.
Cross-platform Development with Flutter
One of the most significant advantages of Dart is its close integration with the Flutter framework. Flutter is a popular open-source UI toolkit developed by Google for building beautiful and natively compiled applications for mobile, web, and desktop platforms. Dart serves as the primary programming language for Flutter, enabling developers to build cross-platform apps with a single codebase.
Code Sample:
dart import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter App', home: Scaffold( appBar: AppBar( title: Text('My First Flutter App'), ), body: Center( child: Text('Hello, Flutter!'), ), ), ); } }
The above code sample showcases a basic Flutter app written in Dart. It creates a simple “Hello, Flutter!” application with a centered text widget. Flutter’s declarative UI programming model, combined with Dart’s expressiveness, makes building rich and responsive user interfaces a breeze.
Tools and Libraries
Dart ecosystem provides a rich set of tools and libraries that enhance development productivity. The Dart SDK includes a comprehensive set of command-line tools for building, testing, and analyzing Dart code. It also offers a package manager called Pub, which allows developers to easily manage and share their Dart libraries.
Furthermore, the Dart community has developed numerous third-party libraries and packages that cover a wide range of functionalities, including network requests, database access, state management, and more. These libraries, along with the robust Flutter ecosystem, provide developers with a vast array of resources to accelerate their development process.
Conclusion
In this blog, we have explored the power of Dart programming language and its key features. Dart’s strong typing, support for asynchronous programming, object-oriented capabilities, compilation modes, and integration with Flutter make it a versatile language for developing high-performance applications. Whether you’re building web, mobile, or desktop apps, Dart empowers developers to write clean, efficient, and maintainable code. With its growing ecosystem and vibrant community, Dart continues to evolve and establish itself as a language of choice for modern application development.
Table of Contents
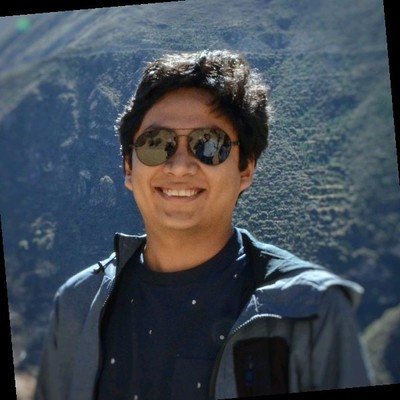
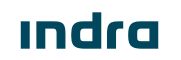