Dependency Management with Dart: Managing Packages and Dependencies
In modern software development, the ability to efficiently manage external code libraries and dependencies is crucial. This becomes even more evident in languages like Dart, which is gaining popularity for building web, mobile, and desktop applications. Dart’s package management system simplifies the process of incorporating third-party libraries into your projects, streamlining development and enhancing code reusability. In this blog post, we’ll dive into the world of dependency management with Dart, exploring how to manage packages effectively and ensure a smooth development workflow.
Table of Contents
1. Understanding Dependency Management
1.1. What are Packages and Dependencies?
In Dart, a package is a collection of Dart code that provides a set of features or utilities. Packages can include anything from UI components and networking libraries to utility functions and more. Dependencies, on the other hand, refer to other packages that your project relies on to function correctly.
Imagine you’re building a web application that requires HTTP requests. Instead of writing the HTTP request handling code from scratch, you can use an existing HTTP package. This is where dependencies come into play. By specifying the dependencies your project needs, you can easily incorporate existing functionality without reinventing the wheel.
1.2. Why is Dependency Management Important?
Dependency management brings several benefits to your development process:
- Code Reusability: Utilizing existing packages saves time and effort, as you can leverage well-tested solutions rather than starting from scratch.
- Efficiency: When well-managed, dependencies ensure that your codebase remains lean and focused on your application’s unique features.
- Collaboration: Teams can collaborate more effectively when they share a common understanding of the packages being used, leading to smoother integration and development.
- Maintenance: Properly managed dependencies simplify updates and bug fixes, as you can easily update a package to its latest version.
2. Getting Started with Dart Packages
2.1. Pub: Dart’s Package Manager
Dart uses a package manager called “pub.” Pub is a command-line tool that simplifies the process of managing packages and dependencies in your Dart projects. It helps you find, install, and manage packages effortlessly.
2.2. Creating a pubspec.yaml File
To start managing dependencies, you’ll need to create a pubspec.yaml file in the root directory of your Dart project. This file is where you specify your project’s metadata, dependencies, and other configuration settings.
Here’s a basic example of a pubspec.yaml file:
yaml name: my_dart_app description: A sample Dart application dependencies: http: ^0.13.3
In this example, the name and description fields provide information about your project. The dependencies section lists the packages your project relies on. The http package is specified with a version constraint using the caret (^) symbol and a version number. This constraint indicates that the project can use any version of the http package that is compatible with version 0.13.3.
3. Managing Dependencies
3.1. Specifying Package Dependencies
When specifying dependencies in your pubspec.yaml file, you can define them with various version constraints. These constraints ensure that your project uses compatible versions of packages.
- Use ^ for compatible versions. For example, ^1.2.0 means your project can use any version greater than or equal to 1.2.0 but less than 2.0.0.
- Use >= for a minimum version requirement. For example, >=2.0.0 means your project requires version 2.0.0 or higher.
- Use any to allow any version, which can be risky due to potential compatibility issues.
3.2. Semantic Versioning
Packages in Dart typically follow semantic versioning (SemVer). SemVer consists of three parts: MAJOR.MINOR.PATCH. Increment the:
- MAJOR version when you make incompatible API changes.
- MINOR version when you add new features in a backward-compatible manner.
- PATCH version when you make backward-compatible bug fixes.
Following SemVer helps manage compatibility between packages and makes it easier to understand the potential impacts of upgrading.
3.3. Resolving Dependency Conflicts
As your project grows and accumulates dependencies, it’s possible to encounter conflicts between different packages’ requirements. Pub uses a resolution algorithm to find a compatible set of package versions that satisfy all dependencies. However, in some cases, conflicts might arise.
To resolve conflicts, you can explicitly specify the version of a package or use the dependency_overrides section in your pubspec.yaml file. This section allows you to force specific packages to use particular versions, helping to avoid version clashes.
4. Working with Packages
4.1. Installing Packages
After you’ve defined your project’s dependencies in the pubspec.yaml file, you need to install them. Run the following command in your project’s root directory:
bash dart pub get
This command fetches the required packages and their dependencies, downloading them to your project’s ./pub-cache directory.
4.2. Importing and Using Packages
Once a package is installed, you can import its functionality into your Dart code. For example, if you’ve added the http package to your project, you can use it like this:
dart import 'package:http/http.dart' as http; void main() { final response = await http.get(Uri.parse('https://example.com')); print('Response status: ${response.statusCode}'); }
By using the import statement, you’re making the http package’s functions, classes, and other elements accessible in your code.
5. Best Practices for Dependency Management
5.1. Regularly Update Packages
Keeping your project’s packages up to date is essential for security patches, bug fixes, and new features. Periodically run the following command to update your packages:
bash dart pub upgrade
However, be cautious when updating major versions, as they might introduce breaking changes that require adjustments to your code.
5.2. Use Isolation for Different Projects
If you’re working on multiple projects, it’s a good practice to maintain separate virtual environments for each. Dart’s pub supports creating isolated environments using the dart pub global activate command. This prevents conflicts between packages used in different projects.
5.3. Keep Dependencies Minimal
While it’s tempting to include various packages for every potential use case, it’s wise to keep your dependencies minimal. Unused packages bloat your project’s size and potentially introduce unnecessary complexity.
6. Advanced Dependency Management
6.1. Private Packages
Sometimes, you might want to use a package that’s not publicly available. You can host private packages on your own servers or use platforms like Pub.dev’s private package hosting. To use a private package, add it to your pubspec.yaml file like any other package, but specify the package location using the hosted field.
6.2. Conditional Dependencies
Dart’s pubspec.yaml allows you to specify dependencies conditionally. For example, you might want to use a package only for specific platforms, such as web or mobile. You can achieve this using environment-specific sections:
yaml dependencies: http: ^0.13.3 dev_dependencies: flutter_test: sdk: flutter
In this example, http is a regular dependency, while flutter_test is a development dependency that’s only used when the project is targeting the Flutter platform.
Conclusion
Dependency management is a cornerstone of efficient software development, and Dart’s package management system makes it seamless. By understanding how to define, install, and manage dependencies in Dart projects, you can streamline your development process, collaborate more effectively, and focus on building the unique features that set your application apart. Remember to follow best practices, keep dependencies minimal, and regularly update packages to ensure your projects stay secure and up to date. With these tools and insights, you’re well-equipped to navigate the world of Dart dependency management with confidence. Happy coding!
Table of Contents
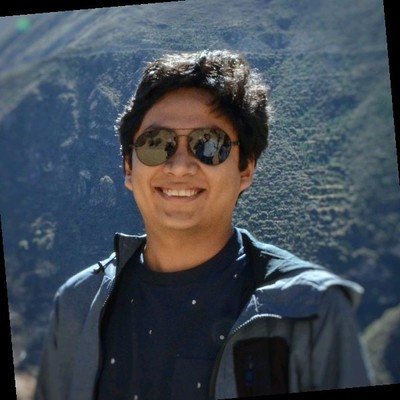
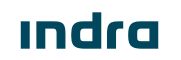