Implementing Design Patterns in Dart: Reusable Solutions for Common Problems
In the world of software development, creating maintainable, scalable, and efficient code is of utmost importance. Design patterns offer a proven way to achieve these goals by providing reusable solutions to common programming problems. Dart, a versatile programming language often used for building web and mobile applications, can greatly benefit from the implementation of these design patterns. In this article, we’ll dive into the world of design patterns and explore how to effectively implement them in Dart to create more organized and robust codebases.
Table of Contents
1. Understanding Design Patterns
Design patterns are general solutions to recurring problems that developers encounter during software development. They provide a structured approach to solving these problems by offering a set of best practices that have been refined over time. Using design patterns not only improves code quality but also makes it more readable, maintainable, and adaptable to change.
There are three main categories of design patterns:
- Creational Patterns: These patterns focus on the process of object creation, providing ways to create objects in a manner that suits the situation. Examples include the Singleton, Factory, and Builder patterns.
- Structural Patterns: Structural patterns deal with the composition of classes and objects to form larger structures. They help define relationships between different components. Examples include the Adapter, Decorator, and Facade patterns.
- Behavioral Patterns: Behavioral patterns address how objects interact and communicate with each other. They provide solutions for creating efficient communication channels between objects. Examples include the Observer, Strategy, and Command patterns.
Now, let’s delve into some of these design patterns and see how they can be effectively implemented in Dart.
2. Singleton Pattern
The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. This can be useful for scenarios where you want to have a single point of control, such as managing configuration settings or creating a logging service.
dart class Logger { static Logger _instance; factory Logger() { _instance ??= Logger._internal(); return _instance; } Logger._internal(); void log(String message) { print(message); } }
In this example, the Logger class can only be instantiated once due to the private constructor. The factory constructor is responsible for controlling the instantiation process and ensuring that only one instance of the Logger class exists throughout the application.
3. Factory Pattern
The Factory pattern is used to create objects without specifying the exact class of object that will be created. It provides an interface for creating objects in a super-class, but allows subclasses to alter the type of objects that will be created.
dart abstract class Shape { void draw(); } class Circle implements Shape { @override void draw() { print("Drawing a circle."); } } class Square implements Shape { @override void draw() { print("Drawing a square."); } } class ShapeFactory { Shape createShape(String type) { if (type == "circle") { return Circle(); } else if (type == "square") { return Square(); } return null; } }
Here, the ShapeFactory class encapsulates the object creation process. Depending on the input, it creates instances of different shapes without exposing the instantiation logic to the client code.
4. Observer Pattern
The Observer pattern defines a one-to-many relationship between objects, ensuring that when one object changes state, all its dependents are notified and updated automatically.
dart class Subject { final List<Observer> _observers = []; String _state; String get state => _state; set state(String newState) { _state = newState; notifyObservers(); } void attach(Observer observer) { _observers.add(observer); } void detach(Observer observer) { _observers.remove(observer); } void notifyObservers() { for (var observer in _observers) { observer.update(); } } } abstract class Observer { void update(); } class ConcreteObserver implements Observer { final String _name; final Subject _subject; ConcreteObserver(this._name, this._subject); @override void update() { print("Observer $_name: Subject state changed to ${_subject.state}"); } }
In this example, the Subject maintains a list of observers that are notified whenever its state changes. The ConcreteObserver class subscribes to a subject and gets notified when the subject’s state is updated.
5. Applying Design Patterns to Real-World Scenarios
Design patterns shine when applied to real-world programming scenarios. Consider building a shopping cart for an e-commerce app. The Strategy pattern could be used to handle different payment methods, allowing you to easily add new methods without modifying existing code. The Decorator pattern could help add additional features or discounts to products in the cart. The Facade pattern could simplify interactions between the shopping cart and various backend services.
Conclusion
Implementing design patterns in Dart can greatly enhance the quality and maintainability of your codebase. By leveraging the power of creational, structural, and behavioral patterns, you can effectively tackle common programming problems and create solutions that are not only efficient but also adaptable to change. Whether you’re building web applications, mobile apps, or any other software, understanding and applying design patterns will undoubtedly elevate your development skills and the overall quality of your projects. So go ahead, start integrating these patterns into your Dart projects, and experience the difference firsthand. Happy coding!
In this article, we explored the significance of design patterns in software development and how they can be implemented in Dart. We discussed key patterns such as Singleton, Factory, and Observer, along with their corresponding code samples. By embracing design patterns, you can ensure your Dart projects are more organized, efficient, and maintainable. Whether you’re a beginner or an experienced developer, incorporating these patterns into your coding arsenal can lead to more elegant and robust solutions to common programming challenges.
Table of Contents
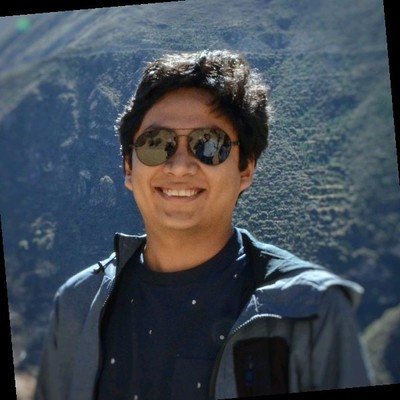
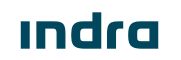