Dart for Desktop Applications: Creating Powerful GUIs
Graphical User Interfaces (GUIs) play a crucial role in modern software development, providing users with an interactive and intuitive way to interact with applications. While Dart is renowned for its usage in web and mobile development, its capabilities for desktop applications are often overlooked. In this blog post, we will explore how Dart can be leveraged to build powerful GUIs for desktop applications, unveiling its potential and versatility in this domain.
Why Choose Dart for Desktop Applications?
Before delving into the nitty-gritty of creating GUIs with Dart, let’s understand why it is a compelling choice for desktop application development.
1. Familiarity and Ease of Learning
Dart’s syntax is approachable and familiar to developers with experience in C-style languages, making it easy to pick up for those already versed in languages like Java, C#, or JavaScript. This familiarity significantly reduces the learning curve, allowing developers to focus on building stunning desktop applications.
2. Cross-platform Compatibility
Dart is designed to be platform-independent. With Flutter, Google’s UI toolkit for building natively compiled applications, you can create cross-platform desktop apps that run seamlessly on Windows, macOS, and Linux without significant modifications to the codebase.
3. Hot Reload for Rapid Development
Dart’s Hot Reload feature, borrowed from Flutter, facilitates rapid development by instantly reflecting code changes in the running application. This dramatically speeds up the development cycle, enabling developers to iterate and fine-tune the GUI in real-time.
4. Robust Ecosystem
Dart benefits from a thriving ecosystem with a wide range of packages and plugins available on pub.dev, its package repository. Whether you need advanced UI components, data handling libraries, or platform-specific integrations, the Dart ecosystem has you covered.
Setting up the Development Environment
To begin building desktop applications with Dart, you need to set up your development environment. Follow these steps to get started:
Step 1: Install Dart SDK
Visit the official Dart website (dart.dev) and download the Dart SDK suitable for your operating system. Once downloaded, follow the installation instructions provided for your OS.
Step 2: Install Flutter
As we’ll be using Flutter for building our desktop GUI, you’ll need to install Flutter alongside Dart. Head to the Flutter website (flutter.dev) and download the appropriate package. After installation, make sure to set the necessary environment variables.
Step 3: Verify Installation
Open your terminal or command prompt and run the following commands to verify that Dart and Flutter are correctly installed:
bash dart --version flutter --version
If the commands display the version numbers, congratulations! You’re all set up and ready to embark on your Dart desktop application development journey.
Building Your First Dart Desktop Application
Let’s start with a simple “Hello, World!” desktop application to ensure everything is working correctly. We’ll use Flutter to create the GUI.
Step 1: Create a New Project
Open your terminal/command prompt and execute the following Flutter command to create a new project:
bash flutter create hello_world_app cd hello_world_app
Step 2: Modify the Main Code
Navigate to lib/main.dart in your project directory. Replace the existing code with the following Dart code:
dart import 'package:flutter/material.dart'; void main() { runApp(HelloWorldApp()); } class HelloWorldApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Hello, World!'), ), body: Center( child: Text( 'Hello, World!', style: TextStyle(fontSize: 24), ), ), ), ); } }
Step 3: Run the Application
Run the following command in the terminal to launch your desktop application:
bash flutter run -d windows
If you’re using macOS or Linux, replace windows with macos or linux, respectively.
Congratulations! You’ve just built and launched your first Dart desktop application. It’s a simple one, but it serves as a foundation for creating more complex and feature-rich GUIs.
Creating a Feature-Rich Desktop Application
Now that you’ve mastered the basics, let’s explore some key components and features you can leverage to create powerful GUIs for your desktop applications.
1. Material Design
Flutter follows Google’s Material Design guidelines, which provide a consistent and visually appealing user experience across platforms. By using Flutter’s Material widgets, you can create beautiful and responsive desktop applications with ease.
dart import 'package:flutter/material.dart'; class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('My Awesome App'), ), body: Center( child: ElevatedButton( onPressed: () { // Add your action here }, child: Text('Click Me'), ), ), ), ); } }
2. Responsive Layouts
Dart and Flutter provide excellent support for building responsive GUIs that automatically adapt to different screen sizes and orientations. The LayoutBuilder and MediaQuery widgets are essential tools for creating responsive user interfaces.
dart import 'package:flutter/material.dart'; class ResponsiveApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Responsive App'), ), body: LayoutBuilder( builder: (context, constraints) { if (constraints.maxWidth > 600) { return DesktopView(); } else { return MobileView(); } }, ), ), ); } }
3. State Management
State management is crucial for interactive desktop applications. Flutter offers various state management solutions like Provider, Bloc, Redux, and MobX. Choose the one that best fits your project’s needs.
dart import 'package:flutter/material.dart'; import 'package:provider/provider.dart'; class CounterApp extends StatelessWidget { @override Widget build(BuildContext context) { return ChangeNotifierProvider<CounterModel>( create: (context) => CounterModel(), child: MaterialApp( home: CounterScreen(), ), ); } } class CounterModel with ChangeNotifier { int _count = 0; int get count => _count; void increment() { _count++; notifyListeners(); } } class CounterScreen extends StatelessWidget { @override Widget build(BuildContext context) { final counter = Provider.of<CounterModel>(context); return Scaffold( appBar: AppBar( title: Text('Counter App'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text( 'Count: ${counter.count}', style: TextStyle(fontSize: 24), ), ElevatedButton( onPressed: () => counter.increment(), child: Text('Increment'), ), ], ), ), ); } }
Conclusion
Dart offers a powerful and versatile platform for creating desktop applications with stunning GUIs. Its cross-platform capabilities, ease of learning, rapid development features, and robust ecosystem make it an excellent choice for modern desktop app development. By harnessing the power of Flutter alongside Dart, you can build feature-rich applications that run seamlessly on Windows, macOS, and Linux. So, don’t hesitate to dive into Dart for your next desktop project and craft impressive, user-friendly applications!
Table of Contents
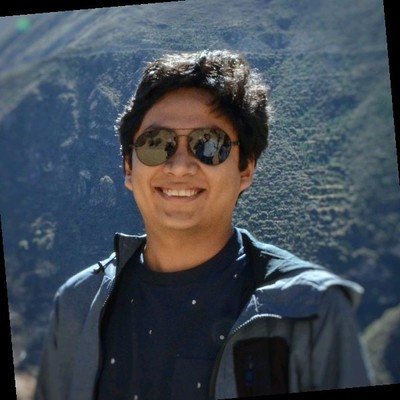
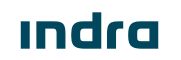