Error Handling and Exceptions in Dart: Writing Robust Code
As developers, we strive to create applications that not only deliver on functionality but also provide a seamless and error-free user experience. To achieve this, error handling and exception management are crucial aspects of coding that can’t be overlooked. In the world of Dart programming, mastering error handling is essential for writing robust and resilient applications. In this blog post, we’ll delve into the importance of error handling, explore the mechanisms Dart provides for managing exceptions, and showcase best practices through code samples.
Table of Contents
1. Understanding the Importance of Error Handling
Imagine you’re building a finance application that involves complex calculations and interactions with external APIs. Even a small oversight or a network hiccup can result in unexpected errors that disrupt the user experience or compromise the integrity of the application’s data. Error handling is the art of anticipating potential issues and having a strategy in place to gracefully handle them.
By implementing proper error handling, you can:
- Improve User Experience: Instead of encountering cryptic error messages or crashes, users can be presented with friendly and informative feedback that guides them towards resolving the issue.
- Maintain Application Stability: Properly handled errors prevent your application from crashing and give you the opportunity to gracefully recover from unexpected situations.
- Debugging and Maintenance: Effective error handling aids in identifying the root causes of issues, making debugging and maintenance more manageable.
2. The Role of Exceptions
In Dart, exceptions are the primary mechanism for handling runtime errors and abnormal conditions. An exception is an instance of a class derived from the Exception class, indicating that something unexpected occurred during the program’s execution. Dart provides a wide range of built-in exception classes, such as FormatException, RangeError, and NoSuchMethodError, to cover various scenarios.
2.1. Throwing Exceptions
In Dart, you can throw an exception explicitly using the throw keyword, followed by an instance of an exception class. For instance:
dart void calculateDivision(int dividend, int divisor) { if (divisor == 0) { throw Exception("Cannot divide by zero"); } // Perform division }
In this example, if the divisor is zero, an exception will be thrown with the specified error message.
2.2. Handling Exceptions
To catch and handle exceptions, Dart provides the try, catch, and optionally, finally blocks. The try block contains the code that might raise an exception, while the catch block handles the exception if one is thrown. The finally block contains code that will be executed regardless of whether an exception was thrown or not.
dart void main() { try { int result = 10 ~/ 0; // Attempting to divide by zero print("Result: $result"); } catch (e) { print("An error occurred: $e"); } finally { print("Execution completed."); } }
In this example, when the division by zero occurs, the catch block is executed, and the error message is printed. The finally block is then executed, ensuring the completion of the execution flow.
2.3. Custom Exception Classes
While Dart provides built-in exception classes, you can also create your own custom exception classes by extending the Exception class or its subclasses. This can be particularly useful when you need to represent specific errors within your application domain.
dart class NetworkException implements Exception { final String message; NetworkException(this.message); } void fetchData() { try { // Simulate a network error throw NetworkException("Unable to connect to the server"); } catch (e) { print("Error: $e"); } }
Creating custom exception classes allows you to encapsulate error details and provide more context when handling exceptions.
3. Best Practices for Effective Error Handling
Writing effective error handling code requires following certain best practices to ensure the robustness of your Dart applications.
3.1. Be Specific with Exception Types
When catching exceptions, try to catch specific exception types whenever possible. This allows you to handle different error scenarios differently and provide more accurate error messages to users.
dart try { // ... } on NetworkException catch (e) { print("Network error: ${e.message}"); } on FileSystemException catch (e) { print("File system error: ${e.message}"); } catch (e) { print("An error occurred: $e"); }
3.2. Graceful Degradation
Implement graceful degradation by anticipating potential errors and providing fallback mechanisms. For instance, if an API call fails, you could offer cached data as an alternative.
dart try { // Make API call } catch (e) { // Use cached data }
3.3. Logging and Monitoring
Implement a logging mechanism to record exceptions and errors. This can aid in diagnosing issues and monitoring the health of your application.
dart try { // ... } catch (e) { logger.error("An error occurred: $e"); }
3.4. Avoid Catching All Exceptions
Avoid catching general exceptions without proper analysis, as it might hide bugs or lead to unexpected behavior. Only catch exceptions that you can handle appropriately.
dart try { // ... } catch (e) { // Handle specific exceptions } finally { // Cleanup }
3.5. Maintain User-Friendly Messages
When presenting error messages to users, strive for clarity and helpfulness. Avoid exposing technical details that users might not understand.
dart try { // ... } catch (e) { showErrorDialog("Oops! Something went wrong. Please try again later."); }
Conclusion
Error handling and exception management are essential skills for writing robust and resilient Dart applications. By anticipating potential issues, understanding the mechanisms of exceptions, and adhering to best practices, you can create a more pleasant user experience and ensure the stability of your codebase. Remember, the goal of error handling is not just to avoid crashes, but also to provide meaningful feedback and maintain the trust of your users. So, the next time you’re coding in Dart, don’t forget to give error handling the attention it deserves. Your users will thank you for it!
Table of Contents
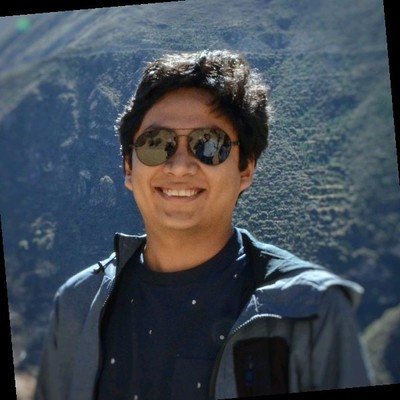
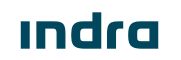