Top 10 Dart Frameworks for Web Development
Dart, developed by Google, is a powerful and versatile programming language that allows developers to build fast, scalable, and efficient web applications. With its clean syntax, strong typing, and asynchronous programming support, Dart has gained popularity among web developers. To make the most out of Dart’s capabilities, developers often turn to frameworks that provide additional tools, libraries, and utilities. In this blog post, we will explore the top 10 Dart frameworks for web development, discussing their features, advantages, and code samples. Whether you’re a seasoned Dart developer or just getting started, these frameworks will help you streamline your development process and build high-quality web applications.
1. AngularDart
AngularDart, developed by Google, is a popular framework for building complex, single-page web applications. It is a Dart port of the widely-used Angular framework, which offers a comprehensive set of tools for building dynamic web applications. AngularDart leverages Dart’s static typing and strong performance to deliver high-quality applications with excellent performance. Let’s take a look at a simple code sample to get started with AngularDart:
dart import 'package:angular/angular.dart'; @Component( selector: 'my-app', template: '<h1>Hello, AngularDart!</h1>', ) class AppComponent {} void main() { runApp(ng.AppComponentNgFactory); }
In the above code, we define an AngularDart component named AppComponent that displays a simple “Hello, AngularDart!” message. The main() function bootstraps the AngularDart application by calling runApp() and passing in the factory generated by Angular’s build system.
2. Aqueduct
Aqueduct is a powerful server-side framework for Dart that focuses on building scalable and high-performance web applications. It follows the Model-View-Controller (MVC) architectural pattern and provides a range of features, including routing, authentication, and database integration. Here’s an example of how to create a basic Aqueduct application:
dart import 'package:aqueduct/aqueduct.dart'; class HelloController extends Controller { @override Future<RequestOrResponse> handle(Request request) async { return Response.ok('Hello, Aqueduct!'); } } class HelloChannel extends ApplicationChannel { @override Future prepare() async { logger.onRecord.listen((rec) => print("$rec")); } @override Controller get entryPoint { final router = Router(); router.route('/hello').link(() => HelloController()); return router; } } Future main() async { final app = Application<HelloChannel>() ..options.configurationFilePath = "config.yaml" ..options.port = 8888; await app.start(); }
In the code above, we define an Aqueduct controller named HelloController that handles requests to the /hello route and returns a “Hello, Aqueduct!” response. The HelloChannel class sets up the application channel and defines the routing configuration. The main() function creates an instance of the Application class, sets the configuration file path, and starts the Aqueduct server on port 8888.
3. Angel
Angel is a full-featured server-side framework for Dart that aims to provide an enjoyable and productive development experience. It offers a wide range of features, including routing, ORM integration, authentication, and much more. Angel follows the MVC architectural pattern and provides a flexible and extensible structure for building web applications. Let’s see a simple example of how to create a basic Angel application:
dart import 'package:angel_framework/angel_framework.dart'; main() async { var app = Angel(); app.get('/', (req, res) => res.write('Hello, Angel!')); await app.startServer('localhost', 3000); }
In the above code, we create an instance of the Angel class and define a route using the get() method. The route / responds with a “Hello, Angel!” message. Finally, we start the Angel server on localhost at port 3000.
4. Flutter
Flutter, although primarily known as a UI framework for building mobile applications, can also be used for web development. Flutter’s web support allows you to build responsive, beautiful, and fast web applications using Dart. Flutter leverages a widget-based approach, enabling developers to create complex UIs with ease. Here’s an example of a basic Flutter web application:
dart import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Hello, Flutter!'), ), body: Center( child: Text('Welcome to Flutter web!'), ), ), ); } }
In the code above, we define a Flutter web application using the MaterialApp widget. The application has a simple app bar and a centered text widget that displays “Welcome to Flutter web!”.
5. Shelf
Shelf is a minimalistic web server framework for Dart that focuses on simplicity and extensibility. It provides a foundation for building custom web applications or middleware. Shelf leverages Dart’s asynchronous programming support, making it suitable for handling high-concurrency scenarios. Here’s an example of a basic Shelf application:
dart import 'package:shelf/shelf.dart' as shelf; import 'package:shelf/shelf_io.dart' as io; void main() { var handler = const shelf.Pipeline().addMiddleware(shelf.logRequests()).addHandler(_echoRequest); io.serve(handler, 'localhost', 8080).then((server) { print('Serving at http://${server.address.host}:${server.port}'); }); } shelf.Response _echoRequest(shelf.Request request) { return shelf.Response.ok('Hello, Shelf!'); }
In the above code, we define a Shelf application that listens on localhost at port 8080. The _echoRequest() function handles incoming requests and returns a “Hello, Shelf!” response. The application also includes middleware to log incoming requests.
6. Redstone
Redstone is a lightweight server-side framework for Dart inspired by Python’s Flask. It provides a simple and intuitive way to define routes and build RESTful APIs. Redstone allows you to create controllers, define routes, and handle requests using annotations. Here’s an example of a basic Redstone application:
dart import 'package:redstone/server.dart' as app; @app.Route('/') helloWorld() => 'Hello, Redstone!'; void main() { app.setupConsoleLog(); app.start(); }
In the code above, we define a route using the @app.Route annotation. The / route responds with a “Hello, Redstone!” message. The main() function sets up the console log and starts the Redstone server.
7. Jaguar
Jaguar is a feature-rich, batteries-included web framework for Dart that focuses on performance and developer productivity. It provides a set of high-level APIs and utilities to build scalable and high-performance web applications. Jaguar offers routing, dependency injection, serialization, and much more. Here’s an example of a basic Jaguar application:
dart import 'package:jaguar/jaguar.dart'; void main() async { final server = Jaguar(); server.get('/', (ctx) => 'Hello, Jaguar!'); await server.serve(); }
In the code above, we create an instance of the Jaguar class and define a route using the get() method. The route / responds with a “Hello, Jaguar!” message. Finally, we start the Jaguar server.
8. OverReact
OverReact is a comprehensive Dart library for building reusable and efficient user interfaces using React.js. It provides a higher-level abstraction over the React.js library, allowing developers to write UI components in Dart instead of JavaScript. OverReact simplifies UI development and provides type safety and code completion benefits. Here’s an example of how to define a simple OverReact component:
dart import 'package:over_react/over_react.dart'; @Factory() UiFactory<HelloProps> Hello = _$Hello; @Props() class _$HelloProps extends UiProps { String name; } @Component() class HelloComponent extends UiComponent<HelloProps> { @override render() { return Dom.h1()('Hello, ${props.name}!'); } } void main() { final hello = Hello()..name = 'OverReact'; react_dom.render(hello, querySelector('#app')); }
In the above code, we define an OverReact component named HelloComponent that accepts a name prop and displays a “Hello, {name}!” message. The main() function creates an instance of the Hello factory and renders it using react_dom.render().
9. Drift
Drift is an ORM (Object-Relational Mapping) framework for Dart that simplifies database integration and management in web applications. It provides a clean and intuitive API for defining models and working with databases. Drift supports a variety of database backends and allows developers to write type-safe queries using Dart’s strong typing. Here’s an example of how to define a simple model using Drift:
dart import 'package:drift/drift.dart'; class Users extends Table { IntColumn get id => integer().autoIncrement()(); TextColumn get name => text()(); } @DriftDatabase() class MyAppDatabase extends _$MyAppDatabase { MyAppDatabase() : super('my_app_database.db'); @override int get schemaVersion => 1; Users get users => Users(); }
In the code above, we define a Drift table named Users with an id column of type int and a name column of type text. The MyAppDatabase class extends _$MyAppDatabase generated by Drift, representing the database instance. The users getter provides access to the Users table.
10. Polymer
Polymer is a Dart library for building web components using the Web Components standard. It allows developers to create reusable and encapsulated UI components that can be easily integrated into web applications. Polymer simplifies component creation, data binding, and event handling. Here’s an example of a simple Polymer component:
dart import 'package:polymer/polymer.dart'; @PolymerRegister('hello-world') class HelloWorld extends PolymerElement { HelloWorld.created() : super.created(); @property String message = 'Hello, Polymer!'; @reflectable void sayHello() { print(message); } }
In the above code, we define a Polymer component named HelloWorld that displays a message and provides a sayHello() method to print the message. The @PolymerRegister annotation registers the component with Polymer.
Conclusion
Dart frameworks offer a wide range of options for web development, catering to various needs and preferences. From server-side frameworks like Aqueduct and Angel to client-side frameworks like AngularDart and Polymer, there’s a Dart framework for every use case. These frameworks provide essential tools, libraries, and abstractions, empowering developers to build high-quality web applications efficiently. Whether you’re looking for a full-featured MVC framework or a minimalistic server for custom applications, Dart has you covered. So go ahead, pick a framework that suits your requirements, and unlock the full potential of Dart in web development. Happy coding!
Table of Contents
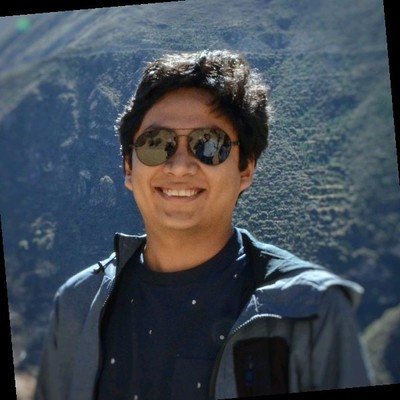
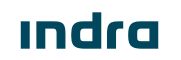