Functional Programming with Dart: Embrace the Paradigm
In recent years, functional programming has gained popularity among developers due to its ability to create more maintainable and robust code. Dart, a powerful and flexible programming language, embraces functional programming paradigms, making it an excellent choice for building scalable and performant applications. In this blog post, we will explore the world of functional programming in Dart, uncover its benefits, and demonstrate how to leverage its features to write clean, concise, and efficient code.
1. What is Functional Programming?
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. It emphasizes immutability, pure functions, and higher-order functions as its core principles. By focusing on expressions rather than statements, functional programming promotes code that is easier to reason about, test, and maintain.
2. Why Use Functional Programming in Dart?
While Dart is primarily known as an object-oriented language, it provides robust support for functional programming. By adopting functional programming principles in Dart, developers can achieve several advantages:
- Simplicity and Conciseness: Functional programming encourages the use of small, composable functions, resulting in concise and readable code. The focus on immutability helps reduce complexity and enables better code comprehension.
- Predictability and Testability: Pure functions, a fundamental concept in functional programming, produce the same output for the same input, regardless of external state. This predictability simplifies testing and debugging, leading to more reliable and bug-free code.
- Modularity and Reusability: Functional programming encourages modular code design, where functions are reusable building blocks. This promotes code reusability and makes it easier to reason about and refactor codebases.
- Concurrency and Parallelism: Functional programming, with its emphasis on immutability and avoiding side effects, facilitates concurrent and parallel programming. Dart’s Isolate API and libraries like RxDart provide excellent support for asynchronous and reactive programming patterns.
3. Key Concepts of Functional Programming in Dart
Let’s explore some of the key concepts that make functional programming in Dart powerful.
3.1 Immutability
In functional programming, immutability is a crucial concept. Immutable objects cannot be modified after they are created, which ensures predictable behavior and prevents accidental changes. Dart provides the ‘final’ keyword to declare variables as immutable, making it easy to create immutable data structures.
dart final int x = 5; final List<int> numbers = [1, 2, 3];
3.2 Higher-Order Functions
Higher-order functions take one or more functions as arguments or return functions as results. They enable code reuse, composition, and encapsulation of behavior. Dart supports higher-order functions, allowing developers to pass functions as parameters or store them in variables.
dart void higherOrderFunction(Function callback) { // Function body callback(); }
3.3 Pure Functions
Pure functions are functions that always produce the same output for the same input and do not cause side effects. They rely only on their arguments and do not modify any external state. Pure functions in Dart are easy to test, reason about, and can be used confidently in concurrent and parallel programming.
dart int add(int a, int b) { return a + b; }
3.4 Recursion
Recursion is a technique where a function calls itself. It is a powerful tool in functional programming to solve complex problems by breaking them down into smaller, self-contained subproblems. Dart supports recursion, allowing developers to write elegant and concise code for tasks that involve repeated operations.
dart int factorial(int n) { if (n <= 1) { return 1; } else { return n * factorial(n - 1); } }
4. Functional Programming Techniques in Dart
Now that we understand the core concepts of functional programming in Dart, let’s explore some techniques and patterns that can enhance our code.
4.1 Using Anonymous Functions
Anonymous functions, also known as lambda functions or closures, are functions without a name. They can be defined inline and are useful when a function is only needed in a specific context. Dart supports anonymous functions, allowing developers to write concise and expressive code.
dart void main() { final numbers = [1, 2, 3, 4, 5]; final evenNumbers = numbers.where((number) => number % 2 == 0); print(evenNumbers); // Output: (2, 4) }
4.2 Function Composition
Function composition is a technique where multiple functions are combined to create a new function. Dart provides the Function.compose method, allowing developers to easily compose functions and create pipelines of transformations.
dart int addOne(int number) => number + 1; int multiplyByTwo(int number) => number * 2; void main() { final addOneAndMultiplyByTwo = multiplyByTwo.compose(addOne); print(addOneAndMultiplyByTwo(3)); // Output: 8 }
4.3 Higher-Order List Operations
Dart provides a rich set of higher-order list operations like map, filter, and reduce, which simplify working with collections. These operations enable developers to express complex transformations and computations succinctly.
dart void main() { final numbers = [1, 2, 3, 4, 5]; final squaredNumbers = numbers.map((number) => number * number); print(squaredNumbers); // Output: (1, 4, 9, 16, 25) final evenNumbers = numbers.where((number) => number % 2 == 0); print(evenNumbers); // Output: (2, 4) final sum = numbers.reduce((value, element) => value + element); print(sum); // Output: 15 }
4.4 Memoization
Memoization is a technique used to cache the results of expensive function calls and return the cached value when the same inputs occur again. Dart provides memoization libraries like memoize that help improve performance by reducing redundant computations.
dart import 'package:memoize/memoize.dart'; int fibonacci(int n) { if (n == 0 || n == 1) { return n; } else { return fibonacci(n - 1) + fibonacci(n - 2); } } void main() { final memoizedFibonacci = memoize(fibonacci); print(memoizedFibonacci(10)); // Output: 55 }
5. Dart Libraries for Functional Programming
Dart offers several libraries that enhance the functional programming experience and provide additional tools and utilities.
5.1 Dartx
Dartx is an extension library that adds useful extension methods to core Dart classes. It provides a wide range of functional programming utilities, such as map operations, filtering, chaining, and more.
dart import 'package:dartx/dartx.dart'; void main() { final numbers = [1, 2, 3, 4, 5]; final squaredNumbers = numbers.map((number) => number * number); print(squaredNumbers); // Output: (1, 4, 9, 16, 25) final evenNumbers = numbers.where((number) => number.isEven); print(evenNumbers); // Output: (2, 4) final sum = numbers.sum(); print(sum); // Output: 15 }
5.2 RxDart
RxDart is an extension of Dart that brings reactive programming concepts and utilities to the language. It enables developers to work with reactive streams and provides powerful operators to handle asynchronous and event-driven programming.
dart import 'package:rxdart/rxdart.dart'; void main() { final subject = PublishSubject<int>(); subject.stream .map((number) => number * 2) .where((number) => number.isEven) .listen(print); subject.add(1); subject.add(2); subject.add(3); subject.add(4); subject.add(5); subject.close(); }
6. Best Practices for Functional Programming in Dart
To make the most of functional programming in Dart, follow these best practices:
6.1 Avoiding Side Effects
Avoid modifying external state or mutable data structures inside functions. Side effects can introduce complexity and make code harder to reason about. Embrace immutability and pure functions whenever possible.
6.2 Using Immutable Data Structures
Prefer immutable data structures to mutable ones, as they make code more predictable and thread-safe. Dart provides immutable collections like UnmodifiableListView and libraries like built_value and freezed for creating immutable data models.
6.3 Leveraging Lazy Evaluation
Lazy evaluation is a technique that delays the evaluation of an expression until its value is actually needed. Dart provides lazy evaluation through iterators and generators, allowing developers to optimize performance by computing values on-demand.
6.4 Unit Testing Functional Code
Functional code is highly testable due to its predictable nature. Leverage Dart’s testing framework to write comprehensive unit tests for your functional code. Focus on testing the input/output relationships of pure functions and verifying the behavior of higher-order functions.
Conclusion
Functional programming brings numerous benefits to Dart developers, including code simplicity, testability, modularity, and support for concurrency. By embracing functional programming concepts and techniques in Dart, developers can write cleaner, more maintainable code that is easier to reason about and test. Dart’s support for immutability, higher-order functions, pure functions, recursion, and libraries like Dartx and RxDart make it an excellent choice for functional programming enthusiasts. So why not explore the power of functional programming in Dart and take your coding skills to the next level?
Table of Contents
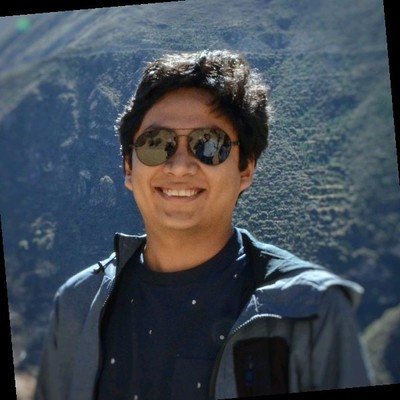
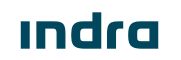