Functional Reactive Programming with Dart: Streamlining Event Handling
In the realm of modern software development, event handling plays a pivotal role in creating responsive and interactive applications. However, managing and orchestrating various events can often lead to complex and convoluted code. This is where Functional Reactive Programming (FRP) comes to the rescue, offering a paradigm that simplifies event-driven code by treating it as data streams. In this article, we will delve into the world of Functional Reactive Programming using Dart, a versatile programming language developed by Google, and learn how to effectively streamline event handling using streams.
Table of Contents
1. Understanding Functional Reactive Programming (FRP)
Before we dive into Dart and its integration with FRP, let’s grasp the essence of Functional Reactive Programming. FRP is a programming paradigm that emphasizes the declarative composition of event-driven code by using functional programming techniques. It treats events as first-class citizens, allowing developers to transform, combine, and manipulate them effortlessly.
At the core of FRP are streams. Streams represent sequences of asynchronous events that can be observed and acted upon. These events can be user interactions, data updates, or any other form of asynchronous occurrences. Instead of dealing with callbacks and imperatively handling events, FRP provides a more elegant approach by providing a unified interface to process and react to these events.
2. Leveraging Streams in Dart
Dart, a modern and versatile programming language, provides native support for working with streams, making it an ideal choice for implementing FRP principles. Dart’s dart:async library offers a rich set of classes and utilities for creating, manipulating, and consuming streams. Let’s explore some key concepts and components of working with streams in Dart.
2.1. Creating Streams
In Dart, you can create a stream using the Stream class and its constructor. Let’s say we want to create a stream of integers:
dart import 'dart:async'; void main() { var stream = Stream<int>.fromIterable([1, 2, 3, 4, 5]); stream.listen((value) { print('Received: $value'); }); }
In this example, we create a stream using Stream<int>.fromIterable, passing in a list of integers. The listen method is used to subscribe to the stream and receive values as they are emitted.
2.2. Transforming Streams
One of the powerful features of streams is the ability to transform them using various operators. Dart’s stream library offers a wide range of operators for transformation, combination, and manipulation. Let’s see an example of using the map operator to transform values in a stream:
dart void main() { var originalStream = Stream<int>.fromIterable([1, 2, 3, 4, 5]); var transformedStream = originalStream.map((value) => value * 2); transformedStream.listen((value) { print('Transformed: $value'); }); }
In this snippet, the map operator is used to double each value in the stream. This showcases how easily you can apply transformations to the data emitted by the stream.
2.3. Combining Streams
Often, you might need to combine multiple streams to react to events from both sources simultaneously. Dart provides the StreamZip class to achieve this. Consider combining two streams of integers:
dart void main() { var stream1 = Stream<int>.fromIterable([1, 2, 3]); var stream2 = Stream<int>.fromIterable([4, 5, 6]); var combinedStream = StreamZip([stream1, stream2]); combinedStream.listen((values) { print('Combined: $values'); }); }
In this example, the StreamZip class takes a list of streams and emits a list of values from each stream as they occur in parallel.
3. Implementing FRP for Event Handling
Now that we have a foundational understanding of streams in Dart, let’s explore how we can leverage FRP principles to streamline event handling within applications. Imagine building a user interface that responds to button clicks. Traditional imperative approaches would involve setting up event listeners and managing state. With FRP, we can simplify this process.
3.1. Creating an Event Stream
Let’s start by creating an event stream for button clicks using Dart’s stream capabilities:
dart import 'dart:async'; void main() { var button = Button(); var clickStream = button.onClickStream; clickStream.listen((event) { print('Button clicked'); }); } class Button { final StreamController<void> _controller = StreamController<void>.broadcast(); Stream<void> get onClickStream => _controller.stream; void click() { _controller.add(null); } }
In this example, we define a Button class with an associated stream controller _controller. The stream controller is set to broadcast mode to allow multiple subscribers. The onClickStream getter exposes the stream to the outside world. When the button is clicked, the click method adds an event to the stream.
3.2. Reacting to Event Streams
With our event stream in place, we can now react to button clicks using FRP techniques:
dart void main() { var button = Button(); var clickStream = button.onClickStream; clickStream .map((event) => 1) // Transform event to a meaningful value .scan<int>((count, event, index) => count + event, 0) // Accumulate event values .listen((count) { print('Button clicked $count times'); }); } class Button { // ... (same as before) // ... (same as before) }
In this snippet, the map operator transforms each button click event into a value of 1. The scan operator then accumulates these values, effectively counting the number of button clicks. The final result is printed to the console whenever the button is clicked.
4. Benefits of FRP in Event Handling
By adopting Functional Reactive Programming, developers can unlock a multitude of benefits when it comes to event handling and application design:
4.1. Declarative and Composable
FRP encourages a declarative approach, where event transformations and reactions are defined in a clear and concise manner. This leads to more readable and maintainable code. The composability of streams allows for easy composition of complex event-handling logic from smaller, reusable components.
4.2. Asynchronous Handling Made Simple
Asynchronous programming can be challenging to manage, especially in applications with numerous events. FRP simplifies asynchronous event handling by abstracting away complex callback chains and allowing developers to focus on the sequence of events.
4.3. Reactivity and Responsiveness
FRP promotes a highly responsive and interactive user experience. By immediately reacting to events and updating the user interface, applications built with FRP feel more intuitive and engaging.
Conclusion
Functional Reactive Programming with Dart provides a modern and elegant way to streamline event handling in applications. By treating events as data streams and leveraging Dart’s native support for streams, developers can simplify complex event-driven code, resulting in more maintainable and efficient applications. The power of FRP lies in its declarative nature, composability, and ability to manage asynchronous events effectively.
Table of Contents
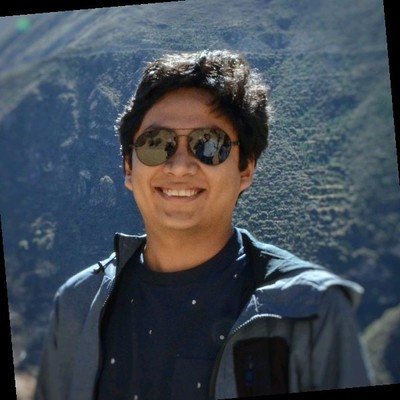
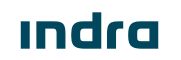