Dart for Game Development: Building Engaging Experiences
In recent years, Dart has gained significant popularity among developers for its versatility and performance. While it is commonly associated with Flutter for building mobile apps, Dart can also be a powerful tool for game development. By leveraging the capabilities of the Flutter framework and its rich ecosystem, developers can create engaging and visually stunning games. In this blog post, we will explore the potential of Dart for game development and guide you through the process of building captivating gaming experiences.
Why Choose Dart for Game Development?
Dart offers several advantages for game development, such as its simplicity, productivity, and the vast ecosystem provided by Flutter. Its single-threaded, event-driven architecture lends itself well to game development, enabling developers to create responsive and interactive experiences. Dart also boasts excellent performance, making it suitable for both 2D and 3D games. With Flutter’s cross-platform capabilities, you can target multiple platforms, including Android, iOS, web, and desktop, with minimal effort.
Setting Up a Game Development Environment
To get started with Dart game development, you need to set up a development environment. Install Dart SDK and Flutter, and configure your IDE for Dart development. This section will guide you through the necessary steps to ensure a smooth setup process.
Core Concepts of Game Development with Dart
Before diving into building games, it’s essential to understand the core concepts of game development. Topics like game loops, rendering, input handling, and state management play a crucial role. We will explore these concepts and learn how to apply them using Dart and Flutter.
Creating Game Mechanics with Dart
Game mechanics define the rules and interactions within a game. In this section, we’ll cover topics such as player input, collision detection, scoring systems, and game states. Through code samples and explanations, you’ll gain a solid understanding of how to implement these mechanics using Dart.
dart // Example code snippet for implementing player input import 'package:flutter/material.dart'; class GameScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( body: GestureDetector( onTap: () { // Handle tap event }, onPanUpdate: (details) { // Handle drag event }, child: // Your game widget here, ), ); } }
Building Game Graphics with Flutter
One of the strengths of Dart for game development is the integration with the Flutter framework, which provides powerful tools for creating visually appealing graphics. We’ll explore how to leverage Flutter’s widgets, animations, and canvas rendering to design captivating game graphics.
dart // Example code snippet for animating game graphics import 'package:flutter/material.dart'; class AnimatedGameWidget extends StatefulWidget { @override _AnimatedGameWidgetState createState() => _AnimatedGameWidgetState(); } class _AnimatedGameWidgetState extends State<AnimatedGameWidget> with SingleTickerProviderStateMixin { AnimationController _animationController; @override void initState() { super.initState(); _animationController = AnimationController( duration: const Duration(seconds: 1), vsync: this, )..repeat(); } @override void dispose() { _animationController.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return AnimatedBuilder( animation: _animationController, builder: (context, child) { return Transform.rotate( angle: _animationController.value * 2 * 3.14, child: // Your game graphics here, ); }, ); } }
Implementing Game Physics and Interactions
Physics and interactions are essential aspects of game development. We’ll delve into concepts like collision detection, physics simulation, and object interactions. Using Dart libraries or creating your own physics engine, you can create immersive and interactive game experiences.
Optimizing Game Performance
To ensure smooth gameplay and a responsive user experience, optimizing game performance is crucial. In this section, we’ll explore techniques such as rendering optimizations, memory management, and efficient game logic implementation. These optimizations will help you deliver high-performance games using Dart.
Testing and Debugging Games
Thorough testing and debugging are essential for a successful game launch. Dart provides a range of testing frameworks and tools to assist you. We’ll cover unit testing, integration testing, and debugging techniques specific to game development, ensuring that your game is stable and bug-free.
Publishing and Monetizing Your Dart Games
Once your game is ready, it’s time to share it with the world. We’ll guide you through the process of publishing your game to various platforms, including app stores and the web. Additionally, we’ll explore different monetization strategies, such as in-app purchases and advertisements, to help you generate revenue from your game.
Game Development Resources and Further Learning
In this final section, we’ll provide a curated list of resources, tutorials, and communities to help you continue your journey in Dart game development. These resources will equip you with the knowledge and support needed to further enhance your game development skills.
Conclusion
Dart and Flutter present a compelling combination for game development, allowing developers to create engaging and visually stunning experiences. By leveraging the power of Dart’s simplicity, performance, and the rich ecosystem provided by Flutter, game developers can build captivating games for various platforms. Whether you’re a beginner or an experienced developer, Dart for game development offers an exciting opportunity to unleash your creativity and build immersive gaming experiences.
Table of Contents
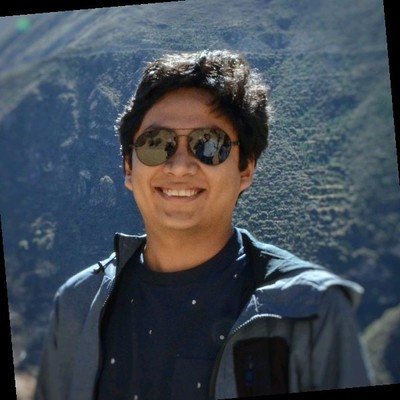
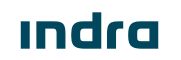