Machine Learning in Dart: Training Models and Making Predictions
Machine Learning (ML) has revolutionized various industries by enabling computers to learn from data and make predictions or decisions without being explicitly programmed. While languages like Python have been dominant in the ML space, Dart, a language primarily associated with front-end web development and Flutter app development, has also started gaining traction in the ML community. In this blog post, we’ll delve into the exciting realm of Machine Learning in Dart, focusing on training models and making predictions within Dart applications.
Table of Contents
1. Why Machine Learning in Dart?
Dart is known for its performance, versatility, and its use in creating responsive and visually appealing applications using Flutter. With the integration of TensorFlow, a popular open-source ML framework, Dart can now empower developers to build and deploy ML models directly within their Dart codebase. This opens up new possibilities for creating ML-powered mobile and web applications, offering seamless user experiences and efficient model execution.
2. Setting Up the Environment
Before we dive into training ML models in Dart, let’s ensure our environment is properly set up. You’ll need to have Dart and the necessary packages installed. You can set up a Dart environment using the Dart SDK and a code editor like Visual Studio Code.
Next, you’ll need to install the ‘tflite’ package, which allows us to work with TensorFlow Lite models in Dart. To install it, add the following dependency to your pubspec.yaml file:
yaml dependencies: tflite: ^1.0.0
After adding the dependency, run flutter packages get to fetch and install the package.
3. Loading a Pretrained Model
Most often, in real-world scenarios, we don’t start from scratch when training ML models. We often use pretrained models and fine-tune them for specific tasks. TensorFlow Lite models are a great choice due to their compact size and efficiency.
Let’s load a pretrained image classification model using the tflite package. First, download a TensorFlow Lite model (with a .tflite extension) that performs image classification. Then, place the model file in your project directory.
In your Dart code, you can load the model as follows:
dart import 'package:flutter/material.dart'; import 'package:tflite/tflite.dart'; void main() async { WidgetsFlutterBinding.ensureInitialized(); await Tflite.loadModel( model: 'assets/image_classification_model.tflite', labels: 'assets/labels.txt', ); runApp(MyApp()); } class MyApp extends StatelessWidget { // Your app code here }
Replace ‘assets/image_classification_model.tflite’ with the actual path to your pretrained model and ‘assets/labels.txt’ with the path to the text file containing class labels for the model.
4. Making Predictions
With the model loaded, it’s time to make predictions on new data. For example, let’s say we want to classify an image from the user’s camera or gallery.
First, we need to preprocess the input image to match the input format expected by the model. TensorFlow Lite models often require images to be resized, normalized, and converted to a specific format. Here’s how you can preprocess an image:
dart import 'dart:typed_data'; import 'package:image/image.dart' as img; import 'package:tflite/tflite.dart'; Uint8List preprocessImage(Uint8List imageBytes) { img.Image image = img.decodeImage(imageBytes)!; img.Image resizedImage = img.copyResize(image, width: 224, height: 224); Float32List input = Float32List(224 * 224 * 3); for (int y = 0; y < 224; y++) { for (int x = 0; x < 224; x++) { var pixel = resizedImage.getPixel(x, y); input[y * 224 * 3 + x * 3] = (pixel >> 16 & 0xFF) / 255.0; input[y * 224 * 3 + x * 3 + 1] = (pixel >> 8 & 0xFF) / 255.0; input[y * 224 * 3 + x * 3 + 2] = (pixel & 0xFF) / 255.0; } } return input.buffer.asUint8List(); }
The preprocessImage function resizes the image to 224×224 pixels and normalizes the pixel values.
Next, let’s use the preprocessed image to make predictions using the loaded model:
dart Future<List<Map<String, dynamic>>> classifyImage(Uint8List imageBytes) async { Uint8List input = preprocessImage(imageBytes); List<dynamic>? results = await Tflite.runModelOnBinary(input: input); return results!.map<Map<String, dynamic>>((dynamic result) { return {'label': result['label'], 'confidence': result['confidence']}; }).toList(); }
The classifyImage function preprocesses the image and uses the Tflite.runModelOnBinary method to obtain classification results.
5. Integrating with Flutter UI
To complete the ML-powered app experience, integrate the prediction process with a Flutter UI. You can use the camera plugin to capture images from the user’s camera. Once the image is captured, display the results of the classification on the screen.
Here’s a simplified example of how you can integrate the camera plugin with the ML model:
dart import 'package:flutter/material.dart'; import 'package:camera/camera.dart'; import 'dart:typed_data'; List<CameraDescription> cameras = []; void main() async { WidgetsFlutterBinding.ensureInitialized(); cameras = await availableCameras(); await Tflite.loadModel( model: 'assets/image_classification_model.tflite', labels: 'assets/labels.txt', ); runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'ML in Dart', home: CameraScreen(), ); } } class CameraScreen extends StatefulWidget { @override _CameraScreenState createState() => _CameraScreenState(); } class _CameraScreenState extends State<CameraScreen> { late CameraController _controller; late Future<void> _initializeControllerFuture; @override void initState() { super.initState(); _controller = CameraController( cameras[0], ResolutionPreset.medium, ); _initializeControllerFuture = _controller.initialize(); } @override void dispose() { _controller.dispose(); super.dispose(); } Future<void> _takePictureAndClassify() async { try { await _initializeControllerFuture; XFile image = await _controller.takePicture(); Uint8List imageBytes = await image.readAsBytes(); List<Map<String, dynamic>> results = await classifyImage(imageBytes); // Display results to the user } catch (e) { print(e); } } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Camera')), body: FutureBuilder<void>( future: _initializeControllerFuture, builder: (context, snapshot) { if (snapshot.connectionState == ConnectionState.done) { return CameraPreview(_controller); } else { return Center(child: CircularProgressIndicator()); } }, ), floatingActionButton: FloatingActionButton( onPressed: _takePictureAndClassify, child: Icon(Icons.camera), ), ); } }
In this example, the _CameraScreenState class manages the camera controller and the image classification process. The _takePictureAndClassify function captures an image and passes it to the classifyImage function for prediction. The results can then be displayed to the user.
Conclusion
Machine Learning in Dart brings a new dimension to Flutter app development. With the integration of TensorFlow and the ‘tflite’ package, you can harness the power of ML to create sophisticated applications that make intelligent predictions. Whether it’s image classification, object detection, or natural language processing, Dart’s potential in the ML field is expanding rapidly. As you embark on your journey of exploring Machine Learning in Dart, remember that continuous learning and experimentation are key to mastering this exciting technology.
In this blog post, we’ve covered the basics of training models and making predictions using TensorFlow Lite models in Dart. From setting up your environment to integrating ML with a Flutter UI, you now have the tools to start creating ML-powered applications using Dart. So, dive in, experiment, and unlock the potential of Machine Learning in Dart!
Table of Contents
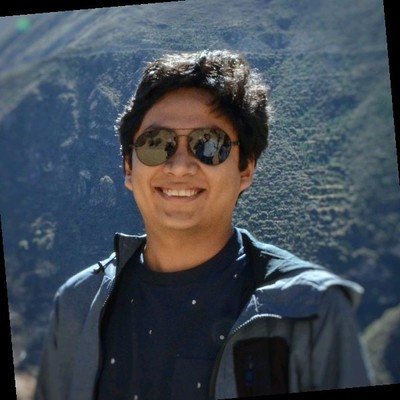
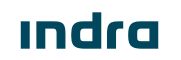