Exploring Dart’s Metaprogramming Capabilities: Code Generation and Reflection
Metaprogramming is a fascinating concept in the world of programming, allowing developers to write code that manipulates or generates other code. Dart, a modern programming language developed by Google, provides robust metaprogramming capabilities through code generation and reflection. These features empower developers to create more dynamic and efficient applications, enabling tasks like generating repetitive code, optimizing performance, and enhancing the development process. In this blog post, we’ll delve into Dart’s metaprogramming features, focusing on code generation and reflection, their use cases, and providing illustrative code samples.
Table of Contents
1. Understanding Metaprogramming
Metaprogramming involves writing code that manipulates or generates other code during compilation or runtime. Dart, being a statically-typed language, offers two primary metaprogramming techniques: code generation and reflection. Code generation is the process of creating code dynamically, typically during build time, while reflection involves inspecting and interacting with the structure of objects and classes during runtime.
2. Code Generation in Dart
2.1. Generating Boilerplate Code
One common use case for code generation is reducing boilerplate code. Dart’s source_gen library is a powerful tool that facilitates the generation of repetitive code, such as getters, setters, and serialization methods for data classes. Let’s consider a scenario where you’re building a data-driven application and need to create numerous model classes with similar properties and methods. Instead of manually writing repetitive code, you can use code generation to automate the process.
dart import 'package:source_gen/source_gen.dart'; @immutable class DataModel { final String name; final int age; const DataModel(this.name, this.age); } @DataModelGenerator() @DataModel('John', 30) class User {} void main() {}
In this example, the @DataModelGenerator annotation triggers the code generation process. The code generator inspects the annotated class and generates necessary boilerplate code, such as constructor, getter, and setter methods. This approach not only saves time but also reduces the chances of introducing errors due to manual code duplication.
2.2. Building Serialization Libraries
Serialization is the process of converting objects into a format suitable for storage or transmission, often in JSON or XML. Dart’s code generation capabilities are particularly valuable when building serialization libraries. You can define the structure of your data classes and generate serialization and deserialization methods automatically.
dart import 'package:json_annotation/json_annotation.dart'; part 'user.g.dart'; @JsonSerializable() class User { final String username; final String email; User(this.username, this.email); factory User.fromJson(Map<String, dynamic> json) => _$UserFromJson(json); Map<String, dynamic> toJson() => _$UserToJson(this); }
By using the json_serializable package, you can annotate your data class and generate serialization methods with a single command. This eliminates the need to write repetitive serialization code for each data class, ensuring consistency and reducing the chance of errors.
3. Reflection in Dart
3.1. Inspecting and Modifying Code Structure
Reflection allows developers to examine and manipulate the structure of code during runtime. While Dart’s reflection capabilities are more limited compared to languages like Python or Java, they can still be valuable in certain scenarios. For example, reflection can be used to implement dynamic plugins or create runtime debugging tools.
dart class MyClass { void printInfo() { print('This is an instance of MyClass'); } } void main() { var instance = MyClass(); // Using reflection to call a method by its name instance.invoke(#printInfo, []); }
In this code snippet, the invoke method uses reflection to call the printInfo method of the MyClass instance. This demonstrates how reflection can provide a level of dynamism to your applications.
3.2. Implementing Generic Algorithms
Reflection can also be useful when implementing generic algorithms that need to work with a variety of object types. Instead of writing multiple algorithms for each type, you can use reflection to adapt the algorithm based on the object’s characteristics.
dart void printProperties(Object obj) { final instanceMirror = reflect(obj); instanceMirror.type.declarations.forEach((symbol, declaration) { if (declaration is VariableMirror && !declaration.isStatic) { print('${MirrorSystem.getName(symbol)}: ${instanceMirror.getField(symbol).reflectee}'); } }); } class Person { String name = 'John'; int age = 30; } void main() { final person = Person(); printProperties(person); }
In this example, the printProperties function uses reflection to inspect and print the properties of an object dynamically. This allows you to write a single function that can work with various object types, promoting code reusability.
4. Use Cases for Metaprogramming
4.1. Dependency Injection
Metaprogramming can simplify dependency injection by automatically generating the necessary code for wiring up dependencies. This is especially valuable in larger projects with complex dependency graphs.
4.2. Serialization and Deserialization
Code generation is a natural fit for serialization and deserialization tasks. By defining your data structures with annotations, you can generate the corresponding serialization and deserialization logic.
4.3. User Interface Building
Metaprogramming can assist in building user interfaces by automatically generating repetitive UI components based on data models.
5. Exploring Code Generation
5.1. Source Gen Library
The source_gen library provides a foundation for creating code generators in Dart. It allows you to define custom annotations, which trigger code generation when applied to classes or methods.
5.2. Writing Code Generators
Creating a code generator involves writing a Dart library that utilizes the source_gen library. You define an annotation class, a generator class, and methods to generate code based on annotated elements.
6. Harnessing Reflection
6.1. Mirrors Library
Dart’s dart:mirrors library provides reflection capabilities, enabling you to inspect and modify code structures at runtime. However, note that this library is not available in Flutter due to its impact on app size.
6.2. Introspecting Code
With the mirrors library, you can access class metadata, inspect fields and methods, and even invoke methods by name.
7. Best Practices and Caveats
- Use metaprogramming judiciously, as it can introduce complexity and make code harder to understand.
- Be cautious with reflection, as it can impact performance and may not be available in all environments.
- Document your code thoroughly, especially when using metaprogramming techniques, to make the codebase more accessible to other developers.
Conclusion
Dart’s metaprogramming capabilities, including code generation and reflection, offer powerful tools for enhancing productivity and code quality. Code generation streamlines the creation of repetitive code and simplifies tasks like serialization, while reflection allows for dynamic interactions with code structures. By understanding and utilizing these features judiciously, developers can create more efficient and dynamic Dart applications, improving the development process and user experience.
Incorporating metaprogramming into your development toolkit can unlock new possibilities and solutions to complex problems. As you explore Dart’s metaprogramming features, you’ll discover innovative ways to streamline your codebase, enhance performance, and create more maintainable applications.
Table of Contents
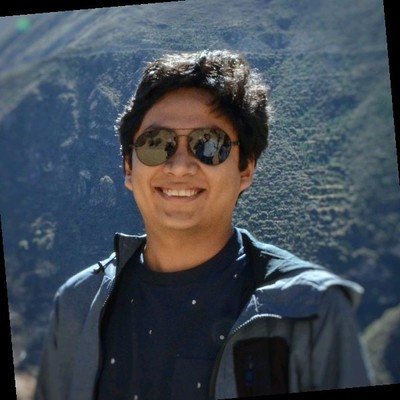
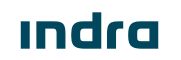