Dart for Mobile App Development: Building Cross-Platform Apps
In today’s digital landscape, mobile app development has become an integral part of businesses and organizations. The demand for apps that run seamlessly on multiple platforms is higher than ever. Enter Dart, a versatile and efficient programming language that empowers developers to build cross-platform mobile apps with ease. In this blog, we will delve into the world of Dart, explore its capabilities, and provide practical code samples to help you build robust and high-performance cross-platform mobile apps.
Introduction to Dart
Dart is a powerful programming language developed by Google, known for its simplicity, speed, and versatility. It combines familiar syntax with modern features, making it an ideal choice for mobile app development. Dart is used extensively in conjunction with the Flutter framework, which allows developers to create high-quality, native-like user interfaces for iOS and Android using a single codebase.
Benefits of Using Dart for Mobile App Development
Dart offers numerous advantages for building cross-platform mobile apps. Some key benefits include:
- Productivity: Dart’s concise syntax and powerful tooling enable developers to write clean and maintainable code, boosting productivity.
- Hot Reload: The Flutter framework provides a hot reload feature, allowing for quick code changes and instant app updates during development.
- Fast Performance: Dart’s Just-in-Time (JIT) compilation enables fast development cycles, while its Ahead-of-Time (AOT) compilation ensures highly optimized performance in production.
- Native-like UI: With Flutter, developers can build beautiful and responsive user interfaces that look and feel like native apps on both iOS and Android platforms.
- Access to Native APIs: Dart allows seamless integration with native code and access to device-specific APIs, enabling developers to leverage platform-specific features.
Setting Up the Dart Development Environment
Before diving into mobile app development with Dart, it’s essential to set up your development environment. This involves installing Dart SDK, Flutter SDK, and configuring your IDE (e.g., Visual Studio Code or Android Studio) to work with Dart and Flutter.
Building Cross-Platform Apps with Flutter
Flutter is a UI toolkit built on top of Dart, designed specifically for building natively compiled applications for mobile, web, and desktop from a single codebase. Let’s explore the process of building cross-platform apps with Flutter through the following steps:
1. Installing Flutter SDK
To begin, we need to install the Flutter SDK and set up the necessary environment variables. Flutter provides comprehensive documentation on its official website, guiding you through the installation process for various operating systems.
Code Sample: Flutter installation command for macOS via Homebrew
css brew install --cask flutter
2. Creating a New Flutter Project
Once Flutter is installed, you can create a new Flutter project using the flutter create command. This will set up a basic project structure with the necessary files and directories.
Code Sample: Creating a new Flutter project
lua flutter create my_app
3. Understanding the Flutter Project Structure
A Flutter project consists of various directories and files. Understanding the project structure is crucial for efficient development.
Code Sample: Flutter project structure
css my_app/ |- lib/ | |- main.dart |- android/ |- ios/ |- pubspec.yaml
4. Building User Interfaces with Flutter Widgets
Flutter provides a wide range of customizable widgets for creating stunning user interfaces. Widgets are the building blocks of Flutter apps, and they can be composed to create complex UI elements.
Code Sample: Creating a simple Flutter widget
dart import 'package:flutter/material.dart'; class MyWidget extends StatelessWidget { @override Widget build(BuildContext context) { return Container( child: Text('Hello, World!'), ); } }
5. Managing State in Flutter
State management is a crucial aspect of mobile app development. Flutter offers several options for managing state, including setState, Provider, Riverpod, and more. Understanding and implementing proper state management techniques is essential for building scalable and maintainable apps.
Code Sample: Using the Provider package for state management
dart import 'package:flutter/material.dart'; import 'package:provider/provider.dart'; class CounterModel extends ChangeNotifier { int _count = 0; int get count => _count; void increment() { _count++; notifyListeners(); } } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return ChangeNotifierProvider( create: (_) => CounterModel(), child: MaterialApp( home: MyHomePage(), ), ); } } class MyHomePage extends StatelessWidget { @override Widget build(BuildContext context) { final counter = Provider.of<CounterModel>(context); return Scaffold( appBar: AppBar( title: Text('Counter App'), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text( 'Count: ${counter.count}', style: TextStyle(fontSize: 24), ), RaisedButton( child: Text('Increment'), onPressed: () => counter.increment(), ), ], ), ), ); }6.
6. Interacting with APIs and Data Persistence
Mobile apps often require interaction with APIs to fetch data or perform various operations. Dart provides excellent support for making HTTP requests using packages like http or dio. Additionally, Dart offers libraries like sqflite and shared_preferences for local data persistence.
Code Sample: Fetching data from an API using the http package
dart import 'package:http/http.dart' as http; Future<void> fetchData() async { final response = await http.get(Uri.parse('https://api.example.com/data')); if (response.statusCode == 200) { final data = response.body; // Process the data } else { throw Exception('Failed to fetch data'); } }
7. Testing and Debugging Flutter Apps
Flutter provides a comprehensive testing framework for writing unit tests, widget tests, and integration tests. Additionally, tools like the Dart DevTools and Flutter Inspector assist in debugging and profiling Flutter apps for performance optimization.
8. Deploying Flutter Apps
Once your Flutter app is ready, you can deploy it to the Google Play Store or Apple App Store. Flutter provides detailed documentation on the necessary steps for app signing, releasing, and publishing.
Dart Best Practices for Mobile App Development
To ensure efficient and maintainable code, it’s essential to follow Dart best practices. Here are some key practices to consider:
- Writing Clean and Maintainable Code: Follow the Dart style guide, use proper naming conventions, and ensure code readability.
- Leveraging Dart Packages and Libraries: Utilize existing packages from the Dart ecosystem to save development time and enhance functionality.
- Optimizing Performance: Apply performance optimization techniques, such as minimizing unnecessary re-renders, reducing widget rebuilds, and using asynchronous operations.
- Ensuring App Security: Implement secure coding practices, including data encryption, proper authentication mechanisms, and secure network communication.
- Handling Platform-Specific Features: Use platform-specific code and packages to handle device-specific features and ensure a native-like experience.
Conclusion
Dart has emerged as a powerful language for building cross-platform mobile apps, thanks to its simplicity, speed, and versatility. With Flutter as the UI toolkit, developers can create visually stunning and high-performance apps that run seamlessly on multiple platforms. By following Dart best practices and leveraging the extensive ecosystem of Dart packages, you can unlock the full potential of mobile app development with Dart. Start exploring the possibilities and build your next cross-platform mobile app with Dart and Flutter!
Table of Contents
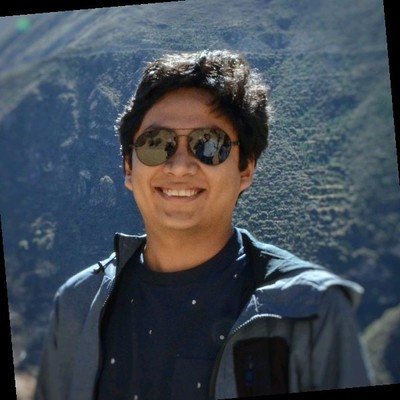
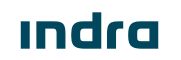