Dart for Network Programming: Socket-based Communication
In today’s interconnected world, network communication plays a pivotal role in various applications, from online gaming to real-time messaging and data sharing. Dart, a versatile programming language developed by Google, provides a robust set of tools and libraries for efficient network programming. One of the key techniques in this domain is socket-based communication, which allows applications to exchange data over the network. In this blog post, we’ll explore the fundamentals of socket-based communication using Dart, along with code samples and practical use cases.
Table of Contents
1. Understanding Sockets: The Building Blocks
Sockets serve as the foundation for network communication, enabling the exchange of data between different devices or applications. A socket can be thought of as an endpoint for sending or receiving data over a network. Dart offers the Socket class, which is part of the dart:io library, for working with sockets.
1.1. Creating a Socket
To establish a connection, you first need to create a socket. Here’s a basic example:
dart import 'dart:io'; void main() async { final server = await ServerSocket.bind('127.0.0.1', 8080); print('Server listening on ${server.address}:${server.port}'); await for (var socket in server) { handleConnection(socket); } } void handleConnection(Socket socket) { print('Connection from ${socket.remoteAddress}:${socket.remotePort}'); socket.write('Hello, client!\n'); socket.close(); }
In this example, we create a simple server that listens on the address 127.0.0.1 and port 8080. When a client connects, the handleConnection function is called to handle the interaction. The server writes a greeting message to the client and then closes the connection.
2. Client-Server Interaction
Socket-based communication involves both client and server components. The client initiates a connection to the server, and the server responds. Let’s explore how this interaction works in Dart.
2.1. Creating a Client Socket
To establish a connection as a client, you can use the Socket.connect method:
dart import 'dart:io'; void main() async { final socket = await Socket.connect('127.0.0.1', 8080); socket.listen((data) { print('Received: $data'); socket.close(); }); socket.write('Hello, server!'); }
In this code snippet, the client connects to the server at address 127.0.0.1 and port 8080. The socket.listen method listens for incoming data from the server. The client sends a greeting message to the server using the socket.write method.
3. Handling Data
Socket communication is all about sending and receiving data. Dart provides mechanisms to handle data efficiently.
Sending and Receiving Text
dart import 'dart:io'; void main() async { final server = await ServerSocket.bind('127.0.0.1', 8080); await for (var socket in server) { socket.listen((data) { print('Received: $data'); socket.write('Message received!'); }); } }
In this example, the server listens for incoming connections and responds with a message when data is received. The client, as shown earlier, can use the write method to send text to the server.
3.1. Sending and Receiving Binary Data
Socket communication isn’t limited to text; you can also send and receive binary data, such as images or files. Here’s a simplified example:
dart import 'dart:io'; void main() async { final server = await ServerSocket.bind('127.0.0.1', 8080); await for (var socket in server) { socket.add([0x48, 0x65, 0x6C, 0x6C, 0x6F]); // Sending "Hello" final data = await socket.first; print('Received: $data'); } }
In this snippet, the server sends the bytes [0x48, 0x65, 0x6C, 0x6C, 0x6F] (which spell “Hello” in ASCII) to the client. The client waits for the first piece of data using socket.first.
4. Use Cases for Socket Communication
Socket-based communication has a wide range of applications. Let’s explore a couple of scenarios where Dart’s networking capabilities shine.
4.1. Real-Time Chat Application
Imagine building a real-time chat application. Sockets enable instant message delivery between users, creating a seamless chatting experience. Dart’s socket libraries provide the tools necessary to establish connections, exchange messages, and handle multiple clients simultaneously.
4.2. Multiplayer Game Networking
For multiplayer games, low-latency communication is crucial. Dart’s socket-based networking can facilitate real-time player interactions, such as moving characters and sharing game state. This ensures that players experience minimal delay and a smooth gaming experience.
Conclusion
Dart’s support for socket-based communication empowers developers to create networked applications that perform efficiently and provide seamless user experiences. In this blog post, we’ve covered the basics of socket communication, including creating sockets, handling data, and exploring practical use cases. Whether you’re building a real-time chat app or a multiplayer game, Dart’s networking capabilities have you covered. So, harness the power of Dart and start building your own networked applications today!
Table of Contents
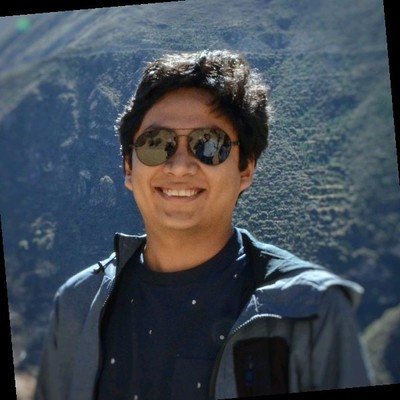
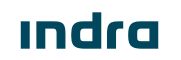