Exploring Dart’s Performance Optimization Techniques
In the ever-evolving landscape of software development, performance optimization plays a pivotal role in ensuring that applications deliver a smooth and responsive user experience. Dart, a versatile programming language primarily known for its use in building web and mobile applications, offers several techniques that developers can employ to enhance the performance of their Dart-based projects. In this blog post, we’ll delve into various performance optimization techniques and best practices, accompanied by code samples and actionable insights.
Table of Contents
1. Understanding the Importance of Performance Optimization
Before diving into the intricacies of performance optimization in Dart, it’s crucial to grasp why it matters. Users have grown accustomed to applications that respond quickly and smoothly, regardless of the platform. Slow-loading pages, unresponsive interfaces, and high resource consumption can lead to frustrated users and negatively impact your application’s reputation.
Efficient performance optimization not only improves user experience but can also lead to increased user engagement, longer session times, and better conversion rates. Moreover, optimized applications tend to consume fewer system resources, resulting in cost savings, especially in cloud-based deployments.
2. Profiling Your Code
Performance optimization begins with identifying bottlenecks in your code. Dart provides tools and libraries for profiling, which helps you pinpoint areas that need improvement. The built-in Observatory tool, for instance, provides insights into memory usage, CPU utilization, and more.
2.1. Using the Observatory Tool
To start using the Observatory tool, import the dart:developer library and add the following line at the beginning of your Dart file:
dart import 'dart:developer';
You can then use the log() function to log messages with various levels of severity. These messages will appear in the Observatory tool’s logging view, giving you insights into the flow of your application.
dart void main() { log('Starting the application', name: 'app_start'); // Your application code here log('Application finished', name: 'app_end'); }
By strategically placing log() statements, you can analyze the sequence of events and identify performance bottlenecks.
3. Efficient Data Structures
Choosing the right data structures can significantly impact your application’s performance. Dart offers various collections, each with its own advantages and trade-offs. For example, when dealing with a large number of items and frequent insertions and deletions, LinkedHashMap might be more efficient than Map.
Example: Using a LinkedHashMap for Performance
dart void main() { var linkedMap = LinkedHashMap<int, String>(); linkedMap[1] = 'apple'; linkedMap[2] = 'banana'; linkedMap[3] = 'cherry'; print(linkedMap); }
In scenarios where you require fast random access, List and Set might be more appropriate choices.
4. Asynchronous Programming
Dart’s asynchronous programming capabilities can greatly enhance the responsiveness of your applications, especially when dealing with tasks that involve I/O operations or network requests.
4.1. Utilizing async and await
The async and await keywords in Dart allow you to write asynchronous code that appears synchronous, making it more readable and maintainable. For example, when making an HTTP request:
dart import 'package:http/http.dart' as http; Future<void> fetchUserData() async { final response = await http.get(Uri.parse('https://api.example.com/user')); if (response.statusCode == 200) { // Process the response } else { // Handle error } }
By utilizing asynchronous programming, your application can continue to perform other tasks while waiting for potentially time-consuming operations to complete.
5. Code Splitting and Lazy Loading
For web applications built using Dart and Flutter, code splitting and lazy loading can significantly reduce initial load times. Code splitting involves breaking your application code into smaller chunks and loading them only when needed.
5.1. Implementing Code Splitting in Flutter
Flutter, a UI toolkit built with Dart, supports code splitting through its import mechanism. You can split your code into separate files and load them on demand. Here’s a simplified example:
dart import 'package:flutter/material.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // Normal app initialization } class MyLazyLoadedComponent extends StatelessWidget { // This component's code is in a separate file }
By employing code splitting and lazy loading, you ensure that your application only loads the necessary components when users interact with them, leading to faster initial load times.
6. Memory Management
Efficient memory management is a cornerstone of performance optimization. Dart’s garbage collector automatically frees up memory occupied by objects that are no longer in use. However, understanding memory usage patterns and optimizing object creation can further improve performance.
6.1. Minimizing Object Creation
Excessive object creation can lead to increased memory consumption and longer garbage collection cycles. Reusing objects or utilizing object pools can mitigate this issue. For instance, when working with frequently changing data, consider using object pools to recycle objects instead of creating new ones.
Conclusion
Optimizing the performance of Dart applications is a multi-faceted endeavor that requires careful consideration of data structures, asynchronous programming, memory management, and more. By profiling your code, making informed choices about data structures, leveraging asynchronous capabilities, implementing code splitting, and managing memory efficiently, you can create Dart applications that deliver exceptional user experiences.
In this blog post, we’ve merely scratched the surface of Dart’s performance optimization techniques. As you continue your journey with Dart, exploring more advanced concepts and experimenting with different strategies will empower you to create high-performance applications that stand out in today’s competitive digital landscape. Remember, the key is to continually monitor, measure, and adapt your optimization strategies as your application evolves, ensuring it remains responsive, efficient, and user-friendly.
Table of Contents
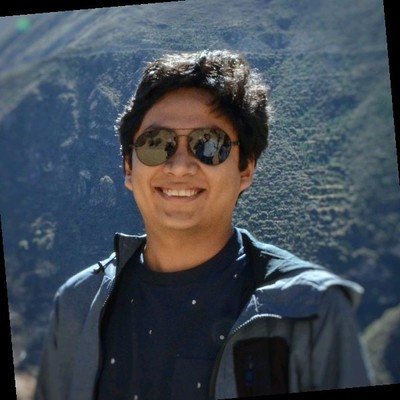
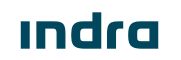