Reactive Streams in Dart: Handling Streams of Data
In the world of programming, data streams are a common occurrence. Whether it’s user input in a graphical user interface, network responses, or any other continuous flow of data, efficiently managing these streams is crucial for maintaining a responsive and robust application. This is where Reactive Streams come into play, providing a powerful way to handle and process streams of data in a reactive and efficient manner. In this article, we will dive into the concept of Reactive Streams in Dart, exploring its benefits and providing code samples to demonstrate its implementation.
Table of Contents
1. Understanding Reactive Streams
Reactive Programming: Before we delve into Reactive Streams, let’s briefly understand the concept of reactive programming. Reactive programming is an approach where you build your application by modeling it as a network of asynchronous data flows, also known as streams. It promotes the propagation of changes and the handling of asynchronous events in a declarative and more predictable way.
- Streams: In Dart, a stream is a sequence of asynchronous events. It’s a way to represent and process a continuous flow of data over time. Streams can emit a variety of events, such as data values, errors, and the completion signal. Streams can be unicast (one-to-one) or multicast (one-to-many).
- Reactive Streams: Reactive Streams take the concept of streams further by providing a standard for asynchronous stream processing with non-blocking backpressure. Backpressure is a mechanism to handle situations when a producer is producing data faster than the consumer can consume it. Reactive Streams enable smooth communication between producers and consumers, ensuring that the system remains responsive and doesn’t run out of memory due to excessive buffering.
2. Benefits of Using Reactive Streams
Reactive Streams offer several advantages when it comes to handling streams of data in Dart applications:
- Efficient Resource Management: Backpressure management ensures that the data flow is controlled, preventing the consumer from being overwhelmed by a rapid influx of data. This leads to efficient resource management and improved application stability.
- Responsive Applications: By preventing data overload, Reactive Streams help maintain application responsiveness. This is crucial in scenarios where the producer and consumer operate at different speeds, such as network requests.
- Memory Efficiency: Reactive Streams handle data in a way that minimizes memory usage. Instead of buffering large amounts of data, the system only keeps a manageable amount at a time.
- Simplified Error Handling: Reactive Streams provide standardized error handling mechanisms, making it easier to manage errors across different parts of your application.
- Modular and Composable: Reactive Streams allow you to compose complex data flows from simpler streams. This promotes modularity and reusability of code.
3. Reactive Streams in Action
To understand how Reactive Streams work in Dart, let’s consider a practical example involving the processing of user search queries in a search bar. We will use the dart:async library to work with streams and the rxdart package for reactive extensions.
3.1. Setting Up the Environment
Before we begin, make sure you have the necessary dependencies installed. In your pubspec.yaml file, add the following:
yaml dependencies: flutter: sdk: flutter rxdart: ^0.27.0
Now run flutter pub get to fetch the dependencies.
3.2. Creating a Search Stream
We’ll create a stream that represents the user’s search queries. This stream will emit events whenever the user types something in the search bar. Here’s how you can set up the stream:
dart import 'package:rxdart/rxdart.dart'; void main() { final searchController = PublishSubject<String>(); // Create a stream from the search controller final searchStream = searchController.stream; // Subscribe to the search stream searchStream.listen((query) { print('Received search query: $query'); // Perform search operation here }); // Simulate user input searchController.add('Dart programming'); searchController.add('Reactive Streams'); // Don't forget to close the stream when done searchController.close(); }
In this example, we used the PublishSubject class from the rxdart package to create a stream controller. The stream emits events whenever the user types a search query. We added some sample queries to demonstrate how the stream works.
3.3. Applying Backpressure
Now, let’s consider a scenario where the search operation takes some time, and we want to ensure that the stream doesn’t overwhelm the system with too many queries at once. We can apply backpressure using the throttleTime operator from rxdart:
dart import 'package:rxdart/rxdart.dart'; import 'dart:async'; void main() { final searchController = PublishSubject<String>(); final searchStream = searchController.stream .throttleTime(Duration(milliseconds: 500)); // Apply backpressure searchStream.listen((query) { print('Received search query: $query'); // Simulate search operation delay Future.delayed(Duration(seconds: 2), () { print('Search completed for: $query'); }); }); // Simulate user input searchController.add('Dart programming'); searchController.add('Reactive Streams'); searchController.close(); }
In this example, the throttleTime operator ensures that only one event is emitted within a specified time window (500 milliseconds in this case). This helps prevent rapid successive events from overwhelming the search operation.
Conclusion
Reactive Streams provide an elegant solution for handling streams of data in Dart applications. They enable efficient resource management, responsive applications, and simplified error handling. By using libraries like rxdart, you can implement reactive programming patterns and handle backpressure effectively. Understanding and implementing Reactive Streams can lead to more maintainable and performant Dart applications. So, the next time you find yourself dealing with data streams, consider harnessing the power of Reactive Streams to elevate your application’s efficiency and responsiveness.
In this article, we explored the concept of Reactive Streams in Dart, discussed their benefits, and provided practical code samples to demonstrate their usage. As you continue your journey in Dart development, remember that mastering Reactive Streams can greatly enhance your ability to build robust and reactive applications.
Table of Contents
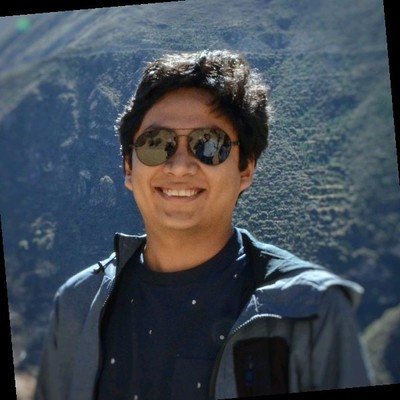
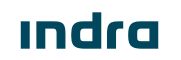