Dart for Server-Side Development: Building Scalable Applications
In the world of modern web and application development, scalability and performance are crucial factors. As your user base grows, your application should gracefully handle the increased traffic and demand. One way to achieve this is by choosing the right technology stack. Dart, a powerful and versatile programming language, is gaining popularity as an excellent choice for server-side development. In this blog, we’ll explore the benefits of using Dart for building scalable applications, its features, and some code samples to get you started.
Why Choose Dart for Server-Side Development?
1. Single Language for Frontend and Backend
Dart is unique as it enables developers to use the same language for both frontend and backend development. This is made possible with the use of the Flutter framework, which allows Dart to build beautiful and performant user interfaces for web, mobile, and desktop applications. The ability to share code between the client and server simplifies development, reduces code duplication, and enhances maintainability.
2. High Performance
Dart is designed with performance in mind. It’s compiled to efficient native code, which leads to faster execution times and better overall performance compared to interpreted languages. With server-side Dart, you can handle a high number of concurrent connections without sacrificing speed.
3. Scalability
Scalability is a critical concern for any application expected to grow over time. Dart excels at building scalable applications due to its asynchronous programming model. Using the async and await keywords, developers can write non-blocking code, making it easier to handle multiple requests concurrently without creating bottlenecks.
4. Strongly Typed Language
Dart is a statically typed language, meaning that type checking is performed during compile-time rather than runtime. This helps catch errors early in the development process, reducing the chances of unexpected issues in production. Strong type annotations also improve code readability and maintainability, making it easier for teams to collaborate effectively.
5. Rich Standard Library
Dart comes with a comprehensive standard library that includes essential utilities for handling collections, dates, and I/O operations. This library simplifies common tasks and ensures you have the tools you need to build robust server-side applications without relying heavily on external dependencies.
Getting Started with Dart for Server-Side Development
To start building scalable applications with Dart, follow these steps:
Step 1: Install Dart SDK
Begin by installing the Dart SDK, which includes the Dart compiler and various tools necessary for development. You can find installation instructions for your operating system on the official Dart website.
Step 2: Choose a Web Framework
Dart offers several web frameworks to choose from, depending on your project requirements and personal preferences. Some popular options include:
Aqueduct
Aqueduct is a feature-rich and high-performance web framework for Dart. It’s well-suited for building RESTful APIs and takes advantage of Dart’s asynchronous capabilities to handle a large number of concurrent requests efficiently.
dart // An example Aqueduct route import 'package:aqueduct/aqueduct.dart'; class UserController extends ResourceController { @Operation.get() Future<Response> getAllUsers() async { final users = await fetchUsersFromDatabase(); return Response.ok(users); } }
Angel
Angel is a flexible and powerful framework with a focus on simplicity and extensibility. It comes with a wide range of plugins and is suitable for various types of applications, from small prototypes to large-scale projects.
dart // An example Angel route import 'package:angel_framework/angel_framework.dart'; void configureRoutes(Angel app) { app.get('/api/users', (req, res) async { final users = await fetchUsersFromDatabase(); return res.json(users); }); }
Step 3: Set Up Database Integration
Scalable applications often require data persistence, and Dart provides support for various databases through packages like mongo_dart, postgres, and sqljocky for MongoDB, PostgreSQL, and MySQL respectively. Choose the one that best fits your needs and integrate it into your project.
dart // Example: Connecting to a PostgreSQL database import 'package:postgres/postgres.dart'; void main() async { final connection = PostgreSQLConnection('localhost', 5432, 'my_database'); await connection.open(); // Use the connection for database queries // ... await connection.close(); }
Step 4: Implement Asynchronous Operations
Leverage Dart’s async and await keywords to write asynchronous code, allowing your application to handle multiple requests concurrently. This is especially useful for I/O-bound operations like reading from a database or making API calls.
dart Future<void> fetchUsersFromDatabase() async { final results = await database.query('SELECT * FROM users'); // Process the results // ... }
Step 5: Write Unit Tests
Unit testing is essential to ensure the correctness of your application’s logic and maintain its stability during future updates. Dart has excellent support for writing unit tests with packages like test and mockito.
dart // Example: Unit test using the `test` package import 'package:test/test.dart'; void main() { test('Addition test', () { expect(1 + 1, equals(2)); }); }
Best Practices for Building Scalable Dart Applications
1. Optimize Database Queries
Efficient database queries play a crucial role in the performance of your application. Ensure that you index the columns used in frequently executed queries and avoid unnecessary data retrieval.
2. Use Caching
Implement caching mechanisms for frequently accessed data to reduce database load and response times. Dart provides caching libraries like cached_network_image for image caching.
3. Load Balancing
As your application scales, consider using load balancing techniques to distribute incoming requests across multiple server instances. This helps evenly distribute the workload and prevent overloading a single server.
4. Error Handling
Proper error handling is essential for maintaining the reliability of your application. Utilize Dart’s try-catch blocks to handle exceptions gracefully and provide meaningful error messages to users.
Conclusion
Dart is a powerful language for server-side development, offering high performance, scalability, and code sharing capabilities with the frontend. By choosing Dart, you can build robust and scalable applications that can handle increased user demand as your project grows. With its extensive standard library, asynchronous programming model, and various web frameworks to choose from, Dart is an excellent choice for building modern and efficient server-side applications. So why wait? Start your journey with Dart for server-side development today and unlock the potential of scalable applications. Happy coding!
Table of Contents
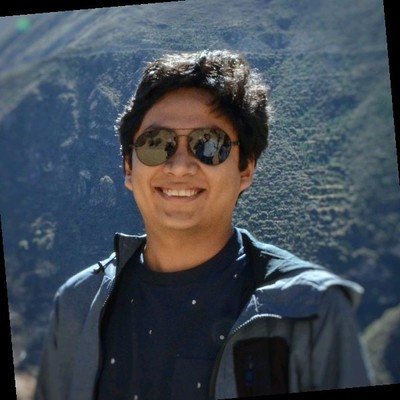
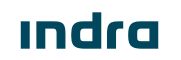