Test-Driven Development with Dart: Ensuring Code Quality
In today’s fast-paced software development world, ensuring code quality is of paramount importance. The quality of code directly impacts the reliability, maintainability, and scalability of applications. One approach that has gained popularity among developers for its ability to boost code quality is Test-Driven Development (TDD). TDD is a software development practice that involves writing tests before writing the actual code. This blog will explore Test-Driven Development with Dart, a versatile and efficient programming language, and demonstrate how it can lead to more robust, cleaner, and maintainable code.
What is Test-Driven Development (TDD)?
- Test-Driven Development is a software development methodology that emphasizes writing tests before implementing the code. It follows a simple and iterative process:
- Write a Test: The developer writes a test that describes the expected behavior of the code. Initially, this test will fail since the implementation is not yet written.
- Implement the Code: The developer then writes the minimal amount of code required to make the test pass. This step ensures that the implementation meets the test criteria.
- Refactor the Code: Once the test passes, the developer can refactor the code to improve its design without changing the functionality. Since there are tests in place, any regressions can be easily detected.
- Repeat: This process is repeated for each new feature or piece of functionality.
Advantages of Test-Driven Development
Adopting Test-Driven Development with Dart can yield several advantages for developers and projects:
- Improved Code Quality: TDD helps catch bugs early in the development process, leading to higher code quality. The tests act as safety nets, alerting developers if any subsequent code changes introduce issues.
- Maintainable Codebase: Writing tests first encourages developers to think about the design and structure of their code thoroughly. This often results in a more maintainable and modular codebase.
- Faster Debugging and Refactoring: With TDD, developers can quickly identify the root cause of a bug by running the related test cases. It also provides the confidence to refactor code knowing that tests will detect any potential regressions.
- Collaboration and Documentation: Tests serve as documentation for the expected behavior of the code. They also facilitate collaboration among team members, as new developers can understand the code’s intent by looking at the tests.
- Continuous Integration (CI) and Continuous Deployment (CD): TDD fits well with CI/CD pipelines, allowing automated testing and quicker feedback, which streamlines the development and deployment process.
Getting Started with Test-Driven Development in Dart
Now that we understand the benefits of TDD let’s dive into practical examples of how to implement Test-Driven Development with Dart. For this demonstration, we’ll create a simple function and test its behavior using Dart’s built-in testing framework called test.
Setting up a Dart Project
Before we begin, ensure you have Dart SDK installed on your machine. If not, download and install it from the official Dart website (https://dart.dev/get-dart). Once Dart is installed, you can create a new Dart project using the following command:
bash $ mkdir my_project $ cd my_project $ dart create .
Writing the First Test
Let’s start by writing a simple test for a function that adds two numbers. Create a new file named add_test.dart inside the test directory of your Dart project. In this file, we’ll write the test case for our add function:
dart // test/add_test.dart import 'package:test/test.dart'; import '../lib/my_math.dart'; void main() { group('Add Function Test', () { test('Adds two positive numbers', () { expect(add(2, 3), equals(5)); }); test('Adds a positive and a negative number', () { expect(add(5, -3), equals(2)); }); test('Adds two negative numbers', () { expect(add(-2, -3), equals(-5)); }); }); }
Implementing the Function
Now that we have written the test cases, let’s implement the add function in a new file named my_math.dart inside the lib directory:
dart // lib/my_math.dart int add(int a, int b) { return a + b; }
Running the Tests
To run the tests, use the following command in your project’s root directory:
bash $ dart test
If everything is set up correctly, you should see output indicating that all the test cases passed successfully.
Red-Green-Refactor: The TDD Cycle
The TDD cycle follows a pattern known as “Red-Green-Refactor.” Let’s understand each step in more detail:
- Red: In this step, you write a test for the functionality you want to implement. The test should initially fail, represented by the “Red” color, as the implementation is not yet present.
- Green: In this step, you implement the minimal code required to make the test pass. The test should now pass successfully, indicated by the “Green” color.
- Refactor: In this step, you can improve the code’s design, eliminate duplication, and optimize performance without changing the behavior. The tests act as a safety net, ensuring that your refactoring does not introduce regressions.
Conclusion
Test-Driven Development with Dart is a powerful approach to ensure code quality, increase developer productivity, and build more reliable software. By writing tests before the implementation, developers gain a deeper understanding of the expected behavior and can confidently refactor their code without fear of breaking existing functionality.
In this blog post, we explored the concept of Test-Driven Development and its benefits. We also walked through a practical example of implementing TDD in a Dart project. By integrating TDD into your development workflow, you can create cleaner, more maintainable, and bug-free code, providing a solid foundation for your applications to grow and evolve. Happy coding!
Table of Contents
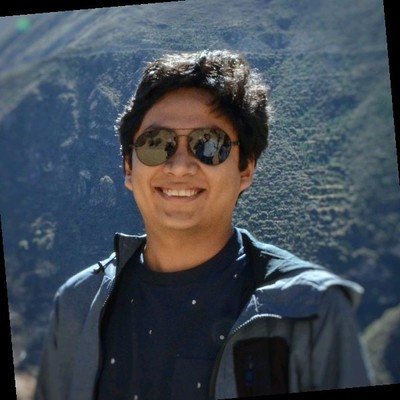
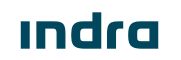