Web Assembly with Dart: Unlocking High-Performance Web Apps
In the world of web development, delivering high-performance applications is crucial to provide users with a seamless and enjoyable experience. Traditional web technologies like JavaScript have come a long way, but sometimes they may not be sufficient to meet the demands of complex applications or computationally intensive tasks. This is where WebAssembly (Wasm) comes into play. In this blog, we’ll explore the synergistic relationship between WebAssembly and Dart, a modern programming language, and see how this duo unlocks the potential for building high-performance web apps.
1. Understanding WebAssembly
1.1 What is WebAssembly?
WebAssembly is a binary instruction format that allows running code written in various programming languages on web browsers at near-native speed. It acts as a low-level virtual machine that enables efficient and secure execution of code on different platforms. Unlike JavaScript, WebAssembly is not tied to any specific language, making it versatile and widely applicable.
1.2 Benefits of WebAssembly
- High Performance: WebAssembly executes at near-native speed, providing significant performance improvements over traditional JavaScript. This makes it suitable for resource-intensive tasks, such as rendering complex graphics or running data-intensive algorithms.
- Language Agnostic: WebAssembly allows developers to write code in their preferred programming languages. Whether it’s C, C++, Rust, or Dart, they can compile their code into WebAssembly modules and run them on the web.
- Improved Security: WebAssembly runs in a sandboxed environment, ensuring that it cannot access the host system directly. This mitigates potential security risks associated with executing untrusted code.
- Small File Size: WebAssembly binaries are compact, resulting in faster download times compared to the equivalent JavaScript files. This is crucial for optimizing web app performance, particularly in low-bandwidth scenarios.
- Easy Integration: WebAssembly modules can be seamlessly integrated with existing web projects. This enables developers to enhance specific parts of their applications without rewriting the entire codebase.
2. Introducing Dart
2.1 What is Dart?
Dart is a modern, open-source programming language developed by Google. It is designed for building fast, scalable, and cross-platform applications. Dart offers a productive development experience with features like a strong type system, Just-In-Time (JIT) and Ahead-Of-Time (AOT) compilation, and a comprehensive standard library.
2.2 Why Choose Dart for WebAssembly?
The combination of Dart and WebAssembly brings numerous advantages to web developers:
- Performance: Dart’s optimized JIT and AOT compilation techniques ensure that the code runs efficiently on both the server and the client-side.
- Productivity: Dart’s clean and concise syntax, along with its powerful tooling, enhances developer productivity. This makes it easier to build and maintain complex web applications.
- Cross-Platform: Dart allows developers to target multiple platforms with the same codebase, including the web, mobile (using Flutter), and server applications.
- Type Safety: Dart’s strong type system catches errors at compile-time, reducing the likelihood of runtime errors and enhancing code reliability.
- Building High-Performance Web Apps with Dart and WebAssembly
- Now, let’s dive into some practical examples to demonstrate how Dart and WebAssembly can be combined to create high-performance web applications.
3. Setting Up the Development Environment
Before we begin, make sure you have the following installed:
- Dart SDK: Download and install the latest Dart SDK from the official Dart website.
- WebAssembly SDK: Install the WebAssembly SDK, which includes tools for compiling code to WebAssembly.
Once the setup is complete, let’s move on to our first example.
Example 1: Image Processing
For our first example, let’s build an image processing application that applies a grayscale filter to an image. We’ll implement the image processing algorithm in Dart and compile it to WebAssembly for improved performance.
dart // image_processing.dart import 'dart:ffi'; // Import the Dart FFI library for working with WebAssembly import 'dart:html'; // Import the Dart HTML library for DOM manipulation // Define the image processing algorithm in Dart int grayscale(int pixel) { int r = (pixel >> 16) & 0xFF; int g = (pixel >> 8) & 0xFF; int b = pixel & 0xFF; int gray = (0.3 * r + 0.59 * g + 0.11 * b).toInt(); return (0xFF << 24) | (gray << 16) | (gray << 8) | gray; } // Implement the image processing in WebAssembly final ffi.DynamicLibrary wasmImageProcessing = ffi.DynamicLibrary.open('image_processing.wasm'); typedef GrayscaleFunc = ffi.Int32 Function(ffi.Int32); typedef Grayscale = int Function(int); void main() { final canvas = querySelector('#canvas') as CanvasElement; final context = canvas.context2D; final img = ImageElement(src: 'example.jpg'); img.onLoad.listen((_) { canvas.width = img.width; canvas.height = img.height; context.drawImage(img, 0, 0); final imgData = context.getImageData(0, 0, img.width, img.height); final data = imgData.data; final grayScaleFunc = wasmImageProcessing .lookup<ffi.NativeFunction<GrayscaleFunc>>('grayscale') .asFunction<Grayscale>(); for (var i = 0; i < data.length; i += 4) { final grayPixel = grayScaleFunc(data[i] << 16 | data[i + 1] << 8 | data[i + 2]); data[i] = (grayPixel >> 16) & 0xFF; data[i + 1] = (grayPixel >> 8) & 0xFF; data[i + 2] = grayPixel & 0xFF; } context.putImageData(imgData, 0, 0); }); }
In this example, we define the grayscale function in Dart to apply the grayscale filter to a given pixel. We then load an image using Dart’s ImageElement and apply the grayscale filter to it using our WebAssembly function grayScaleFunc.
Example 2: Physics Simulation
Another exciting use case for WebAssembly with Dart is creating physics simulations. Let’s implement a simple physics simulation of bouncing balls.
dart // physics_simulation.dart import 'dart:ffi'; // Import the Dart FFI library for working with WebAssembly final ffi.DynamicLibrary wasmPhysicsSim = ffi.DynamicLibrary.open('physics_simulation.wasm'); typedef SimulateFunc = ffi.Void Function(ffi.Pointer<ffi.Float>, ffi.Int32); typedef Simulate = void Function(ffi.Pointer<ffi.Float>, int); void main() { final numBalls = 5; final ballSize = 20; final ballSpeed = 2; final canvas = querySelector('#canvas') as CanvasElement; final context = canvas.context2D; canvas.width = window.innerWidth; canvas.height = window.innerHeight; final ballsPtr = ffi.allocate<ffi.Float>(count: numBalls * 4); for (var i = 0; i < numBalls * 4; i += 4) { ffi.Pointer<ffi.Float> ballPtr = ballsPtr.elementAt(i); ballPtr.store(i.isEven ? canvas.width / 2 : canvas.height / 2); ballPtr.offsetBy(1).store(ballSize); ballPtr.offsetBy(2).store((i.isEven ? ballSpeed : -ballSpeed) * (i % 3 == 0 ? -1 : 1)); ballPtr.offsetBy(3).store((i.isEven ? -ballSpeed : ballSpeed) * (i % 3 == 0 ? -1 : 1)); } final simulateFunc = wasmPhysicsSim .lookup<ffi.NativeFunction<SimulateFunc>>('simulate') .asFunction<Simulate>(); void animate() { context.clearRect(0, 0, canvas.width, canvas.height); simulateFunc(ballsPtr, numBalls); for (var i = 0; i < numBalls * 4; i += 4) { final ballX = ballsPtr.elementAt(i).load(); final ballY = ballsPtr.elementAt(i + 1).load(); context.beginPath(); context.arc(ballX, ballY, ballSize, 0, 2 * pi); context.fillStyle = '#0095DD'; context.fill(); context.closePath(); } window.requestAnimationFrame((_) => animate()); } animate(); }
In this example, we simulate the physics of multiple bouncing balls using WebAssembly. The simulate function in WebAssembly calculates the new positions of the balls based on their current positions and velocities.
Conclusion
The combination of WebAssembly and Dart opens up a new world of possibilities for web developers to build high-performance web applications. The ability to leverage Dart’s language features and WebAssembly’s speed empowers developers to create complex and computationally intensive web applications with ease. Whether it’s image processing, physics simulations, or any other performance-critical task, WebAssembly with Dart is a powerful duo that can unlock the full potential of the modern web.
So, next time you find yourself facing performance bottlenecks in your web app, consider harnessing the power of WebAssembly with Dart to take your web development to new heights! Happy coding!
Table of Contents
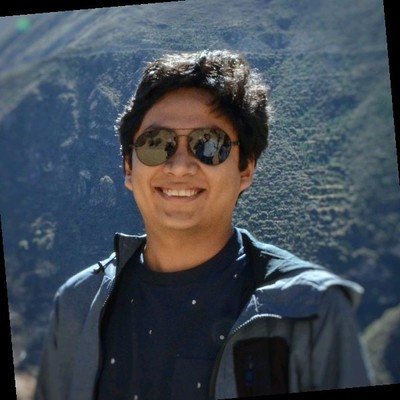
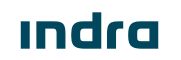