Web Scraping with Dart: Extracting Data from Websites
In the age of data-driven decision-making, accessing and extracting valuable information from websites has become crucial for various applications. Web scraping is the process of automatically gathering data from websites, and it plays a vital role in data analysis, market research, competitive analysis, and more. In this blog, we will explore how to perform web scraping with Dart, a versatile and efficient language for web development, and extract data from websites using various libraries and techniques.
1. Introduction to Web Scraping
1.1 What is Web Scraping?
Web scraping, also known as web harvesting or web data extraction, is the process of extracting data from websites automatically. This data can be in various forms, such as text, images, tables, or links, and can be further processed for analysis or storage. Web scraping is commonly used in various industries for market research, competitor analysis, sentiment analysis, price comparison, and more.
1.2 Legality and Ethics of Web Scraping
Before diving into web scraping, it’s essential to understand the legal and ethical considerations associated with it. While web scraping itself is not illegal, it can infringe on a website’s terms of service or copyright if not done responsibly. Always review a website’s terms of service and robots.txt file to ensure compliance. Additionally, avoid scraping sensitive or personal data, and be mindful of the rate at which you send requests to avoid overloading the server.
2. Introducing Dart for Web Scraping
2.1 Why Choose Dart?
Dart is an open-source, object-oriented programming language developed by Google. It’s known for its high performance, fast development cycles, and excellent tooling support. Dart is particularly well-suited for web development, making it an ideal choice for web scraping projects. Its versatility and ease of use make it a valuable asset when dealing with web scraping challenges.
2.2 Setting Up the Development Environment
To get started with Dart, you’ll need to install the Dart SDK on your system. You can download the SDK from the official Dart website (https://dart.dev/get-dart). Once installed, you can use Dart’s package manager, pub, to manage dependencies for your project.
3. Getting Started with HTTP Requests
3.1 Making HTTP Requests with Dart
HTTP requests are the foundation of web scraping. Dart provides several libraries for making HTTP requests, but one of the most commonly used is the http package. To use it, add the following dependency to your pubspec.yaml file:
yaml dependencies: http: ^0.13.3
Now, you can fetch data from a website using a simple HTTP GET request:
dart import 'package:http/http.dart' as http; void main() async { var url = Uri.parse('https://example.com'); var response = await http.get(url); if (response.statusCode == 200) { print('Response: ${response.body}'); } else { print('Failed to load data, status code: ${response.statusCode}'); } }
3.2 Handling Responses
Once you make an HTTP request, the server will respond with data. The response might contain HTML, JSON, or other data formats. To extract specific data from the response, you’ll need to parse it.
4. Parsing HTML with Dart
4.1 Overview of HTML Parsing
HTML is the most common format for presenting data on websites. To extract information from HTML, we can use the html package in Dart. This package provides convenient methods to parse and manipulate HTML documents.
4.2 Using html Package for Parsing
To use the html package, add it to your pubspec.yaml:
yaml dependencies: html: ^0.15.0 # Check for the latest version
Now, let’s see an example of how to extract specific data from HTML:
dart import 'package:http/http.dart' as http; import 'package:html/parser.dart' show parse; void main() async { var url = Uri.parse('https://example.com'); var response = await http.get(url); if (response.statusCode == 200) { var document = parse(response.body); var titleElement = document.querySelector('title'); print('Title: ${titleElement.text}'); } else { print('Failed to load data, status code: ${response.statusCode}'); } }
In this example, we fetch a web page and parse it using the html package to extract the title of the page.
4.3 Extracting Data from HTML Elements
HTML documents are structured using tags and elements. To extract data from specific elements, you can use CSS selectors or XPath expressions with the html package.
dart import 'package:http/http.dart' as http; import 'package:html/parser.dart' show parse; void main() async { var url = Uri.parse('https://example.com'); var response = await http.get(url); if (response.statusCode == 200) { var document = parse(response.body); var headlines = document.querySelectorAll('h2.headline'); headlines.forEach((element) { print('Headline: ${element.text}'); }); } else { print('Failed to load data, status code: ${response.statusCode}'); } }
In this example, we extract all headlines with the CSS class ‘headline’ from the page.
5. Advanced Web Scraping Techniques
5.1 Handling Pagination
Some websites paginate their content, spreading it across multiple pages. To scrape all pages efficiently, you’ll need to implement pagination logic in your Dart web scraper.
dart import 'package:http/http.dart' as http; import 'package:html/parser.dart' show parse; void main() async { for (var page = 1; page <= 5; page++) { var url = Uri.parse('https://example.com/page/$page'); var response = await http.get(url); if (response.statusCode == 200) { var document = parse(response.body); // Process data from the current page } else { print('Failed to load data, status code: ${response.statusCode}'); } } }
In this example, we scrape data from multiple pages by iterating through the page numbers in the URL.
5.2 Dealing with Dynamic Content (JavaScript-heavy Websites)
Some websites load data dynamically using JavaScript. The http package in Dart doesn’t handle JavaScript execution. For such cases, you can use packages like puppeteer.dart or webdriver to control a headless browser and scrape the dynamically loaded content.
5.3 Using Headless Browsers for Scraping
A headless browser is a browser without a user interface. It allows you to run browser operations programmatically. Dart provides packages like puppeteer.dart or webdriver to control headless browsers like Chromium, Firefox, or Chrome. You can use these packages to scrape websites with JavaScript-rendered content.
6. Storing Scraped Data
6.1 Choosing the Right Data Storage Format
Once you’ve scraped data from websites, you’ll want to store it for further analysis or future use. Common data storage formats include CSV, JSON, databases (SQLite, MongoDB), or cloud storage services (AWS S3, Google Cloud Storage).
6.2 Saving Data to a File or Database
Here’s an example of saving scraped data to a CSV file:
dart import 'dart:convert'; import 'package:http/http.dart' as http; import 'package:csv/csv.dart'; void main() async { var url = Uri.parse('https://example.com/data'); var response = await http.get(url); if (response.statusCode == 200) { var data = jsonDecode(response.body); var rows = List<List<dynamic>>(); data.forEach((item) { rows.add([item['name'], item['price']]); }); var csvData = ListToCsvConverter().convert(rows); await File('data.csv').writeAsString(csvData); } else { print('Failed to load data, status code: ${response.statusCode}'); } }
This example converts scraped data into a CSV format and saves it to a file.
7. Dealing with Anti-Scraping Measures
7.1 Understanding Anti-Scraping Techniques
Some websites implement measures to prevent web scraping, such as rate-limiting, CAPTCHAs, or IP blocking. As a responsible web scraper, it’s essential to understand these measures and avoid actions that may harm the website or violate its policies.
7.2 Implementing Strategies to Avoid Detection
To avoid detection and mitigate anti-scraping measures, you can use techniques like rotating IP addresses, randomizing request headers, or using proxies. However, always remember to adhere to ethical guidelines and respect a website’s terms of service.
8. Best Practices for Web Scraping
8.1 Respect Robots.txt
Robots.txt is a standard used by websites to communicate with web crawlers and scrapers. Always check a website’s robots.txt file and abide by its rules.
8.2 Throttling Requests
Avoid sending too many requests in a short period. Throttle your requests to avoid overloading the server and being mistaken for a DDoS attack.
8.3 Monitoring and Error Handling
Implement monitoring and error handling to ensure your web scraper continues to function correctly and detects issues promptly.
Conclusion
In conclusion, web scraping with Dart opens up a world of possibilities for data extraction and analysis. By utilizing Dart’s power and flexibility, combined with the right libraries and strategies, you can build effective web scrapers to collect valuable data from various websites. However, always remember to act responsibly and ethically while scraping, respecting the terms of service of the websites you access. Happy scraping!
Table of Contents
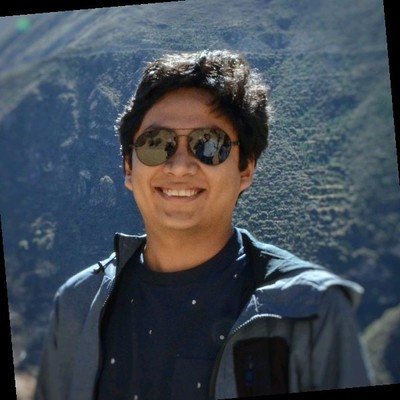
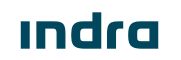