Unlock the Power of Django-Powered Chatbots with Real-world Examples
In today’s rapidly advancing digital age, conversational interfaces, particularly chatbots, are transforming how businesses interact with their users. While numerous tools and platforms can be employed to create chatbots, Django, a popular Python web framework, has gained traction for this purpose due to its scalability, robustness, and ease of use.
Table of Contents
This post will delve deep into how Django can be used to create conversational interfaces, complemented by real-world examples.
1. Why Django for Chatbots?
Django provides a perfect framework for chatbot development, thanks to the following features:
- ORM (Object Relational Mapping): Django’s ORM allows developers to create database tables using Python classes. This makes it easier to store and retrieve chatbot interactions, user data, and more.
- Scalability: As your user base grows, Django can handle increased loads with ease.
- Extensible: With Django’s “app” structure, one can easily add chatbot functionalities as a separate app, keeping the codebase clean and modular.
- Integrated Admin Interface: Monitor chatbot interactions and manage user data seamlessly.
1.1. Real-world Examples of Django-powered Chatbots
Let’s explore some hypothetical examples to illustrate the possibilities:
- E-commerce Chatbot: “ShopBot”
Scenario: An e-commerce website wants to facilitate users in product searching, inquiries, and order tracking.
Implementation:
Models:
Product (name, description, price, stock)
UserInteraction (user, query, response)
Chat Functionality:
Product Search: User types “Show me running shoes,” and the bot fetches relevant products from the database.
Order Tracking: The bot checks the order status in the database based on the order number provided by the user.
1.2. University Inquiry Chatbot: “UniBot”
Scenario: A university wants to answer frequent queries related to admission, courses, and events.
Implementation:
Models:
Courses (name, description, duration, fee)
Events (name, date, description)
Inquiry (user, question, answer)
Chat Functionality:
Admission Process: User asks “How can I apply?”, and the bot provides a step-by-step guide.
Courses Info: On queries like “Tell me about computer science courses,” the bot displays relevant courses from the database.
2. Building a Basic Django Chatbot
To give you a hands-on feel, let’s create a rudimentary chatbot using Django.
Setup:
- Install Django (`pip install django`)
- Create a new Django project (`django-admin startproject chatbot_project`)
- Create a new app (`python manage.py startapp chatbot_app`)
Models:
In `chatbot_app/models.py`, define a basic model for user interactions.
```python from django.db import models class UserInteraction(models.Model): user_query = models.TextField() bot_response = models.TextField() ```
URLs:
Define endpoint `/chat/` to process user messages. In `chatbot_app/urls.py`:
```python from django.urls import path from . import views urlpatterns = [ path('chat/', views.chat, name='chat'), ] ```
Views:
For simplicity, let’s create an echo bot. In `chatbot_app/views.py`:
```python from django.http import JsonResponse from .models import UserInteraction def chat(request): user_message = request.GET.get('message') bot_response = f"You said: {user_message}" # Storing interaction UserInteraction.objects.create(user_query=user_message, bot_response=bot_response) return JsonResponse({"response": bot_response}) ```
Frontend:
You can create a basic HTML page with an input box for the user message and a display area for the bot’s response. Use AJAX to send the user’s message to the `/chat/` endpoint and display the bot’s response.
Conclusion
Django, with its plethora of features, offers an excellent framework for creating scalable and efficient chatbots. Whether it’s assisting online shoppers or answering university inquiries, the potential applications are vast. If you’re looking to integrate a conversational interface into your platform, Django might be the right tool for you. With continuous advancements in AI and natural language processing, there’s no limit to how sophisticated and helpful your Django-powered chatbot can become.
Table of Contents
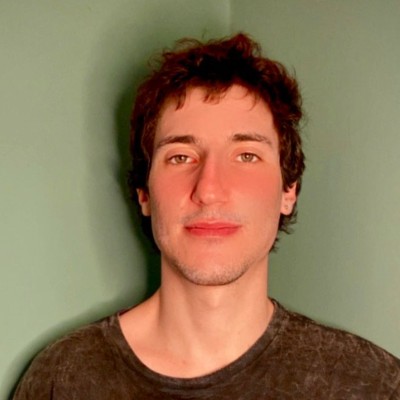
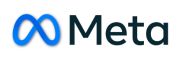