From Cart to Checkout: How Django Elevates E-commerce
Django, the powerful Python-based web framework, is highly suitable for creating robust e-commerce websites. It offers a clean and pragmatic design, promoting rapid development and clean, reusable code. E-commerce platforms require a mix of functionalities, including product management, user authentication, payment processing, and more. In this post, we’ll explore how Django serves as the foundation for building modern online stores and provide a few illustrative examples.
Table of Contents
1. Introduction to Django for E-commerce
The features that make Django perfect for e-commerce applications are:
– Admin Interface: Django offers a built-in admin interface, which can be an excellent starting point for managing products, orders, and customers.
– Scalability: Django can handle large numbers of users and products, which is essential for growing e-commerce platforms.
– Security: It has built-in protections against various web vulnerabilities like CSRF, SQL injections, and XSS attacks.
– Extensibility: Django’s modular design allows the integration of a plethora of third-party apps to extend the functionality of an e-commerce site.
2. Building Basic E-commerce Features with Django
2.1. Product Management
You can model products using Django’s ORM. Here’s a basic product model:
```python from django.db import models class Product(models.Model): title = models.CharField(max_length=200) description = models.TextField() price = models.DecimalField(max_digits=6, decimal_places=2) inventory = models.IntegerField() image = models.ImageField(upload_to='products/') def __str__(self): return self.title ```
This model can be registered with the Django admin to manage products efficiently.
2.2. User Authentication
Django’s built-in `auth` module provides functionalities for user authentication. With a few lines of code, you can implement registration, login, and logout functionalities.
2.3. Shopping Cart
A shopping cart can be represented as a dictionary where keys are product IDs, and values are quantities. This can be stored in Django’s session framework.
```python def add_to_cart(request, product_id): cart = request.session.get('cart', {}) cart[product_id] = cart.get(product_id, 0) + 1 request.session['cart'] = cart return redirect('cart_view') ```
2.4. Payment Processing
Integrating third-party payment gateways like Stripe or PayPal can be done using Django packages such as `dj-stripe` or `django-paypal`.
3. Example E-commerce Platforms built with Django
3.1. Saleor
An open-source platform, Saleor provides a modern e-commerce storefront. It’s powered by a GraphQL API and is written entirely in Python/Django. With a rich set of features, like customizable products, multiple payment gateways, and extensive documentation, Saleor can be an excellent choice for those diving deep into e-commerce.
3.2. Oscar
Oscar is a flexible and extensible Django app, used by companies of varying sizes. It offers a comprehensive dashboard for managing products, stock, and orders. The modular system of Oscar enables developers to replace or extend any part of the core functionalities.
3.3. Shuup
Another open-source solution, Shuup, can cater to single-vendor shops to large multi-vendor platforms. It comes with customizable modules, extensive APIs, and multi-language support.
4. Extending Django for E-commerce
While Django offers a great start, for a feature-rich e-commerce experience, one often needs third-party applications. Here are some recommended extensions:
– Django Allauth: For enhanced user authentication.
– Django Crispy Forms: To style and work with forms effortlessly.
– Django Parler: For multi-language support.
5. Tips for Building E-commerce Websites with Django
- Opt for Progressive Web Apps (PWA): E-commerce platforms benefit from PWA features, like offline browsing and push notifications.
- Prioritize Security: Always keep Django and other dependencies up to date. Use Django’s `check` command to discover potential security vulnerabilities.
- Optimization: Use tools like Django Debug Toolbar to pinpoint bottlenecks and optimize database queries.
- Backup: Ensure that product data, user data, and transactions are regularly backed up.
Conclusion
Django’s robust features and the expansive ecosystem make it a prime choice for e-commerce web applications. With a wealth of third-party apps and a supportive community, building an online store with Django is both feasible and efficient. Whether you’re a budding entrepreneur or an established business, Django offers the tools and scalability you need to succeed in the digital marketplace.
Table of Contents
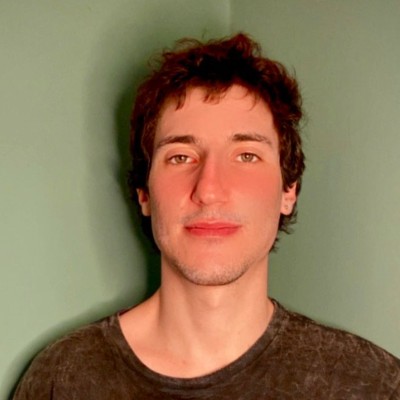
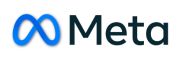