Web Defense 101: Enhancing Security in Django Web Applications
When it comes to creating web applications, security should never be an afterthought. As a developer, your primary goal should be to protect your user’s data and your system’s integrity from a variety of potential attacks. This is where the need to hire Django developers who are well-versed in these aspects becomes critical.
This post will focus on Django, a popular Python web framework known for its “batteries-included” philosophy. Django comes with robust security features out of the box, however, it’s still crucial to understand these protections and know how to enhance them when necessary. This understanding can be easily leveraged when you hire Django developers who are proficient in the framework.
With their expertise, you can build secure and reliable web applications that not only cater to your business needs but also uphold the utmost data integrity and security. Django developers can guide you through this process, ensuring your web application is well-protected against potential attacks.
Introduction to Django Security
Django provides several built-in protections against common web attack vectors. These include Cross-Site Scripting (XSS), Cross-Site Request Forgery (CSRF), SQL Injection, Clickjacking, and Host header validation, among others. However, these protections are effective only when used correctly. So, let’s dive into some of these security measures and provide examples of how they can be implemented to secure your Django web applications.
1. Cross-Site Scripting (XSS) Protection
Cross-site scripting attacks involve malicious scripts being injected into trusted websites, which are then executed by the victim’s browser. Django templates protect against most XSS attacks by escaping specific characters that are potentially dangerous in HTML.
Let’s take a look at a simple Django template:
```django <html> <body> <h1>Welcome, {{ user.username }}!</h1> </body> </html> ```
In this case, if a user’s username contains characters like `<` or `>`, Django will replace them with their HTML escaped counterparts—`<` and `>`, respectively—preventing any injected script from being executed.
However, beware of the `|safe` filter and `{% autoescape off %}` tag in Django templates. They prevent this automatic character escaping, potentially making your application vulnerable to XSS attacks.
2. Cross-Site Request Forgery (CSRF) Protection
Cross-site request forgery attacks trick a victim into submitting a malicious request. They exploit the trust that a site has for a user, not the user’s trust for the site. Django provides CSRF protection by checking for a special token in each POST request. This ensures that the request is made intentionally by the user, not by a malicious site.
To include the CSRF token in your templates, use the `{% csrf_token %}` template tag inside every `<form>` tag:
```django <form method="POST"> {% csrf_token %} <!-- Form fields go here --> <input type="submit" value="Submit"> </form> ```
If you’re working with AJAX requests, you’ll need to add the CSRF token to your JavaScript as well. Django provides a way to get the token from a cookie and include it in the AJAX request.
3. SQL Injection Protection
SQL injection attacks involve inserting malicious SQL code into queries, tricking the system into executing unintended commands. Django protects against SQL injections by using query parameterization, effectively separating the data from the command.
Consider this example:
```python User.objects.get(username=username) ```
In this case, even if `username` contained potentially harmful SQL, Django’s ORM would ensure it’s treated strictly as data, not as a SQL command.
4. Clickjacking Protection
Clickjacking is an attack that tricks a user into clicking a webpage element that is invisible or disguised as another element. This can cause users to unknowingly perform actions on the site. Django prevents clickjacking by providing a middleware that sets the X-Frame-Options header in every HttpResponse.
Enable the clickjacking protection by adding the following middleware to your `MIDDLEWARE` settings:
```python MIDDLEWARE = [ # ... 'django.middleware.clickjacking.XFrameOptionsMiddleware', # ... ] ```
This middleware will set the X-Frame-Options header to ‘SAMEORIGIN’, meaning the response can only be framed by a page of the same origin.
5. Host Header Validation
HTTP Host header attacks occur when a request’s Host header is manipulated, potentially leading to cache poisoning, password reset poisoning, and other security risks. Django protects against this by validating the Host header against the `ALLOWED_HOSTS` setting in your settings.py.
Always set `ALLOWED_HOSTS` to the hostnames that your Django application can serve. For example:
```python ALLOWED_HOSTS = ['yourhostname.com'] ```
Remember, setting `ALLOWED_HOSTS` to [‘*’] in a production setting is a security risk.
Additional Django Security Measures
While Django provides several security measures out of the box, there are additional steps you can take to further secure your application.
1. Use HTTPS
HTTPS encrypts the data between your server and your users. This makes it harder for attackers to eavesdrop or tamper with the data being sent. You should enforce HTTPS for all communication, not just for sensitive transactions.
2. Keep Your Software Updated
Ensure that your Django version, Python version, and all dependencies are kept up to date. Updates often include security patches for known vulnerabilities. Using a package like `pipenv` can help you keep track of your dependencies and their updates.
3. Limit File Uploads
If your Django app allows for file uploads, ensure you validate and sanitize the uploaded files. This will prevent a potential malicious file upload. Also, consider the storage location of these files; do not store them in a location accessible to the public.
Conclusion
In conclusion, security should be an integral part of your web application development process. Django provides robust protections out of the box, but these are only effective when used correctly and complemented with other good security practices. Therefore, it can be highly beneficial to hire Django developers who are well-versed in these practices.
Keeping your software updated, being mindful of user inputs, enforcing secure communications, and routinely auditing your application for potential security vulnerabilities are crucial tasks that competent Django developers can effectively handle.
Remember, a secure application not only protects you and your users, but it also builds trust, a crucial component for any successful web application. Stay vigilant and secure your Django application from potential attacks! With the help of professional Django developers, you can ensure that security remains a top priority in your application’s lifecycle.
Table of Contents
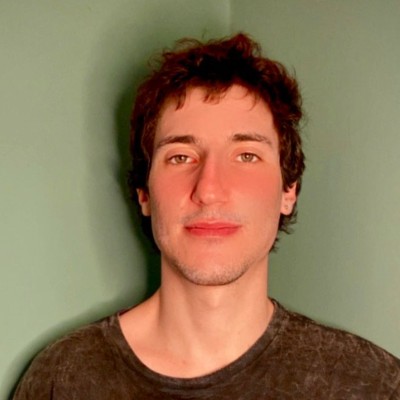
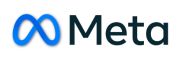