How to Build Multilingual Web Apps with Django: A Detailed Guide
Internationalization (i18n), Localization (L10n), and Multilingualism are no longer mere buzzwords in the field of web development. Today, they’re necessities. As the Internet connects people worldwide, businesses must provide their services in different languages to reach a broader audience. In this context, Django, a Python web framework, offers robust support for internationalization and localization. This has led to an increase in demand to hire Django developers who can adeptly navigate these features. This post will guide you on using Django’s built-in features for building multilingual web applications, showcasing why hiring expert Django developers can be beneficial. We’ll be walking through some hands-on examples to facilitate a better understanding.
Table of Contents
1. Internationalization and Localization: A Brief Overview
Before we delve into the depths of Django, let’s familiarize ourselves with some key terms:
– Internationalization (i18n): It’s the process of designing and preparing your app to be adaptable to different languages, regional differences, and technical requirements of a region or a locale.
– Localization (L10n): Once your app is internationalized, localization means the adaptation of your app to meet the language, cultural, and other requirements of a specific target market (a locale).
2. Django’s Internationalization Framework
Django’s internationalization system lets you translate your application into various languages. It has a unified and active internationalization and localization framework, making it easier for developers to build multilingual applications.
This system consists of:
- A way to specify locale-specific data formats (date, time, number formats).
- A translation mechanism to translate static pieces of text into other languages.
- A method to serve different translated versions of your application to your users.
3. Setting up Internationalization in Django
Here’s a step-by-step guide on how you can use Django’s i18n framework:
Step 1: Install the Required Libraries
The first step is to install gettext libraries, which Django uses for translating text messages. Depending on your operating system, you can install them as follows:
– Ubuntu/Debian: `sudo apt-get install gettext`
– macOS: `brew install gettext`
– Windows: You can download it from [GNU gettext utilities] (http://gnuwin32.sourceforge.net/downlinks/gettext.php).
Step 2: Enable Internationalization in Settings
By default, Django comes with internationalization turned on. If it isn’t, you can enable it by making sure your settings file (`settings.py`) contains the following:
```python USE_I18N = True ```
Also, include Django’s middleware class `LocaleMiddleware` in your MIDDLEWARE setting:
```python MIDDLEWARE = [ # ... 'django.middleware.locale.LocaleMiddleware', # ... ] ```
Note that the order of MIDDLEWARE matters. Place `LocaleMiddleware` after `SessionMiddleware` and `CacheMiddleware`, and before `CommonMiddleware`.
Step 3: Setting Languages
In the `settings.py` file, you can define the languages that your application will support. Django uses the language code as defined by the [ISO 639-1 standard] (https://www.iso.org/iso-639-language-codes.html). For instance, here’s how you’d support English and Spanish:
```python LANGUAGES = [ ('en', 'English'), ('es', 'Spanish'), ] ```
The default language is English. If you want to change it, use `LANGUAGE_CODE` in your settings file:
```python LANGUAGE_CODE = 'es' ```
Step 4: Marking Strings as Translatable
To mark strings as translatable, you’ll use the `gettext` function available in Django:
```python from django.utils.translation import gettext as _ def greet(request): output = _("Hello, world!") return HttpResponse(output) ```
In this example, the string “Hello, world!” is marked for translation. Django will use the appropriate language file to translate this string based on the active language.
Step 5: Creating Language Files
You must run the `makemessages` command to create or update your language files. This command searches all Python, HTML, and templates files in your project and extracts all the strings marked for translation:
```bash python manage.py makemessages -l es ```
This command will generate a `django.po` file in the `locale/es/LC_MESSAGES` directory. Here, ‘es’ is the language code for Spanish.
Step 6: Translating Strings
Open the generated `django.po` file. You’ll find the strings marked for translation:
```po #: main/views.py:4 msgid "Hello, world!" msgstr "" ```
Now, you can translate the string into the target language:
```po #: main/views.py:4 msgid "Hello, world!" msgstr "¡Hola, mundo!" ```
Step 7: Compiling Translations
Once the translation is done, compile the `.po` files into `.mo` files, which Django uses to serve translations:
```bash python manage.py compilemessages ```
Now, your Django application will serve this string in Spanish whenever the active language is set to Spanish.
Step 8: Changing the Active Language
You can use Django’s `set_language` redirect view to provide a way for users to change their language preference. Here’s a simple form that does just that:
```html <form action="{% url 'set_language' %}" method="post">{% csrf_token %} <input name="next" type="hidden" value="{{ redirect_to }}"> <select name="language"> {% get_current_language as LANGUAGE_CODE %} {% get_available_languages as LANGUAGES %} {% get_language_info_list for LANGUAGES as languages %} {% for language in languages %} <option value="{{ language.code }}"{% if language.code == LANGUAGE_CODE %} selected{% endif %}> {{ language.name_local }} ({{ language.code }}) </option> {% endfor %} </select> <input type="submit" value="Go"> </form> ```
Remember to include `i18n` in your template:
```html {% load i18n %} ```
Conclusion
With Django’s robust i18n framework, creating multilingual web applications has never been easier, making it a compelling reason to hire Django developers. Keep in mind, however, that language is just one aspect of localization. True localization also involves addressing cultural nuances, local regulations, and UI/UX practices. Therefore, the way you approach i18n and L10n, possibly with the help of expert Django developers, could be the deciding factor for the global success of your web application.
Table of Contents
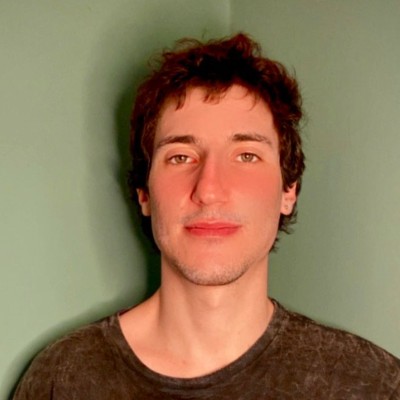
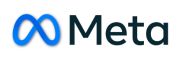