How to set up a Django database?
Setting up a database in Django is a fundamental step in building web applications, as it allows you to store and manage your application’s data efficiently. Django makes database setup relatively painless by providing a high-level and database-agnostic Object-Relational Mapping (ORM) system. Here’s a concise guide on how to set up a database in Django:
- Database Configuration in `settings.py`: Open your project’s `settings.py` file, and locate the `DATABASES` configuration section. Django supports various database backends, including PostgreSQL, MySQL, SQLite, and Oracle. Choose the database backend that suits your project and adjust the settings accordingly. You’ll need to specify the database engine, name, user, password, host, and port. For example, if you’re using SQLite (the default for new projects), the configuration might look like this:
```python DATABASES = { 'default': { 'ENGINE': 'django.db.backends.sqlite3', 'NAME': BASE_DIR / 'db.sqlite3', } } ```
- Creating Database Tables: With the database settings configured, you can create the database tables based on your Django models using the `makemigrations` and `migrate` management commands. Run these commands:
``` python manage.py makemigrations python manage.py migrate ```
The first command generates migration files that describe the changes to your database schema, and the second command applies those changes to the database. This creates the necessary tables for your Django models.
- Administering the Database: You can use the Django admin panel to interact with your database. Log in as a superuser, and you’ll have the ability to add, edit, and delete data directly through the admin interface.
- Interacting with the Database in Views: In your Django views, you can use the ORM to interact with the database. You can query, create, update, and delete records using Python code, without writing SQL queries manually. This simplifies database interactions and ensures database independence.
- Database Queries: Django’s ORM provides a powerful and expressive QuerySet API for querying the database. You can use methods like `filter()`, `get()`, `annotate()`, and `aggregate()` to retrieve data from the database efficiently.
By following these steps, you can set up and configure a database for your Django project. Django’s built-in ORM abstracts away many of the complexities associated with database management, making it easier to develop and maintain your web application’s data layer.
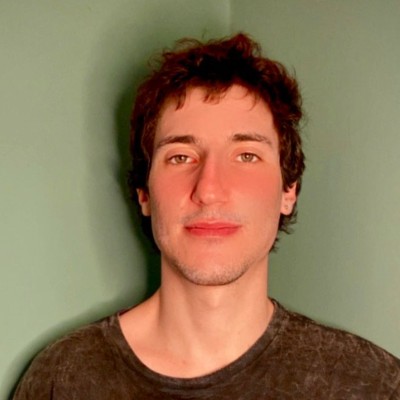
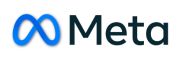