How to start a new app within a Django project?
Starting a new Django app within an existing project is a common task when building complex web applications. Django’s architecture encourages modularity by allowing you to create separate apps for different functionalities, making your codebase organized and maintainable. Here’s how you can start a new Django app within your project:
- Navigate to Your Project Directory: Open your terminal or command prompt and navigate to the root directory of your Django project. This is the directory that contains the `manage.py` file.
- Create a New App: Use the `manage.py` script to create a new app. Replace “appname” with your desired app name. For example:
``` python manage.py startapp appname ```
This command generates a new directory named “appname” in your project’s root directory, along with the necessary files and directories for a Django app.
- Add the App to Your Project: To make Django aware of your new app, you need to add it to your project’s settings. Open the `settings.py` file within your project’s main directory and locate the `INSTALLED_APPS` list. Add the name of your app (e.g., `’appname’`) to the list of installed apps.
```python INSTALLED_APPS = [ # ... 'appname', # ... ] ```
This step informs Django that your app is part of the project and should be included in the project’s functionality.
- Define Models and Views: Now that your app is set up, you can start defining models, views, and templates within the app. Models define your data structure, views handle user interactions, and templates manage the HTML rendering. Be sure to create appropriate directories within your app for these components.
- Configure URLs: Create a `urls.py` file within your app’s directory to define the URL patterns specific to your app. You can then include these patterns in your project’s main `urls.py` file using the `include` function.
- Run Migrations: After defining models, run the following commands to create migrations and apply them to the database:
``` python manage.py makemigrations appname python manage.py migrate ```
These commands ensure that your app’s database tables are created or updated as needed.
- Develop and Test: With your new app set up and configured, you can start developing its functionality, write tests, and ensure that it works as expected. Test your app by running the development server (`python manage.py runserver`) and accessing its URLs in a web browser.
By following these steps, you can efficiently create a new Django app within your existing project, allowing you to modularize your code and organize your project’s functionality effectively. Each app you create can focus on a specific feature or component of your web application, making it easier to manage and maintain your Django project as it grows.
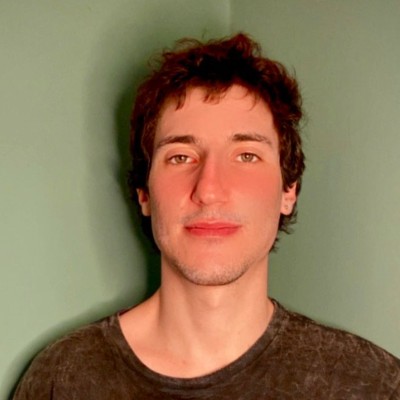
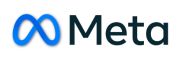