How to handle user permissions and groups in Django?
Handling user permissions and groups in Django is essential for managing access control and defining what different users can and cannot do within your web application. Django provides a built-in and robust system for managing permissions and user groups. Here’s how to handle them effectively:
User Permissions:
- Defining Permissions: In Django, permissions are actions that users can perform, such as “view,” “add,” “change,” or “delete” for specific models. You can define custom permissions in your models using the `Meta` class. For example:
```python class YourModel(models.Model): # Fields class Meta: permissions = [ ("can_custom_action", "Can perform a custom action"), ] ```
- Assigning Permissions: Assign permissions to users or groups using the Django admin panel or programmatically in your code. For instance, to assign a permission to a user:
```python user.user_permissions.add(permission) ```
User Groups:
- Creating Groups: Groups are collections of users who share common permissions. Create groups based on roles or access levels (e.g., “Admins,” “Editors,” “Viewers”) using the Django admin panel or programmatically.
- Assigning Users to Groups: Add users to groups using the Django admin panel or in your code. For example, to add a user to a group:
```python user.groups.add(group) ```
Checking Permissions:
- In Views: In your views, use the `@permission_required` decorator to restrict access to specific views based on user permissions.
```python from django.contrib.auth.decorators import permission_required @permission_required('app_name.can_custom_action') def custom_action_view(request): # View logic ```
- In Templates: In templates, use the `{% if user.has_perm %}` template tag to conditionally display content based on user permissions.
```html {% if user.has_perm 'app_name.can_custom_action' %} <!-- Display content for users with the 'can_custom_action' permission --> {% endif %} ```
By effectively managing user permissions and groups in Django, you can implement fine-grained access control, ensuring that users only have access to the parts of your application that are appropriate for their roles or responsibilities. This enhances security and helps maintain data integrity within your web application.
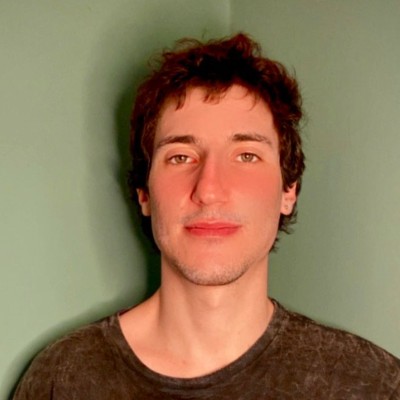
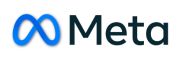