What is the difference between boxing and unboxing in .NET?
In .NET programming, boxing and unboxing are concepts related to the conversion between value types and reference types. While they both involve the transformation of data, they serve different purposes and occur under different circumstances within the .NET runtime.
Boxing:
Boxing is the process of converting a value type to an object type (also known as a reference type) in .NET. When a value type, such as an integer or a structure, needs to be treated as an object, it is boxed by the runtime environment. The value type is encapsulated within an object, which allows it to be stored on the managed heap and treated as a reference type.
For example, if you have an integer variable x, and you assign it to an object variable obj, the integer x is automatically boxed into an object:
csharp int x = 10; object obj = x; // Boxing occurs here
Boxing allows value types to be used in scenarios where reference types are expected, such as collections like ArrayList or List<object>.
Unboxing:
Unboxing is the reverse process of boxing. It involves extracting the value type from the object and converting it back to its original value type. Unboxing is necessary when retrieving a value type from a collection or object and restoring its original type.
Here’s an example of unboxing:
csharp object obj = 10; // Boxed integer int y = (int)obj; // Unboxing occurs here
In this example, the integer value is extracted from the object obj and assigned to the integer variable y.
Performance Considerations:
Boxing and unboxing operations incur performance overhead because they involve memory allocations and type conversions. Therefore, excessive boxing and unboxing operations can degrade the performance of an application, especially in performance-critical code paths.
Boxing and unboxing are mechanisms in .NET that allow value types to be treated as reference types and vice versa. While boxing converts a value type to an object type, unboxing retrieves the original value type from an object. Understanding the differences between boxing and unboxing is essential for writing efficient and performant .NET code.
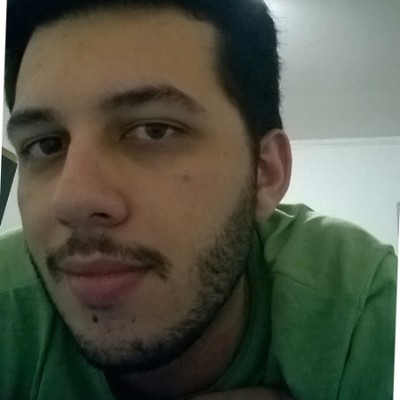
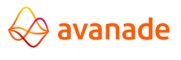