What is LINQ (Language Integrated Query)?
LINQ, which stands for Language Integrated Query, is a powerful feature introduced in the .NET framework that allows developers to query and manipulate data using a unified syntax directly within C# or other .NET languages. LINQ provides a consistent and expressive way to work with data from various sources such as collections, arrays, databases, XML, and more.
At its core, LINQ enables developers to write queries using a syntax that is similar to SQL (Structured Query Language), making it intuitive and familiar to those with database experience. However, unlike SQL, LINQ is integrated directly into the programming language, allowing queries to be written inline with C# or other .NET languages.
LINQ introduces several key components that enable querying and manipulation of data:
Standard Query Operators: LINQ provides a set of standard query operators that allow developers to perform common data manipulation tasks such as filtering, sorting, grouping, joining, and projecting data. These operators are available for various data sources and provide a consistent interface for working with different types of data.
Language Integration: LINQ is seamlessly integrated into the C# language through language extensions and compiler support. This integration allows developers to write queries using familiar language constructs such as lambda expressions, method chaining, and query syntax, making code more readable and maintainable.
Provider Model: LINQ follows a provider model architecture, which allows it to work with a variety of data sources including in-memory collections, databases, XML documents, and more. Providers are responsible for translating LINQ queries into the appropriate language or query syntax for the underlying data source.
Here’s a simplified example of LINQ in action:
csharp // Define a collection of integers List<int> numbers = new List<int> { 1, 2, 3, 4, 5 }; // Query the collection using LINQ var evenNumbers = from num in numbers where num % 2 == 0 select num; // Iterate over the result and print each even number foreach (var num in evenNumbers) { Console.WriteLine(num); }
In this example, LINQ is used to query a collection of integers and select only the even numbers. The query syntax reads similarly to SQL, making it easy to understand and maintain.
LINQ (Language Integrated Query) is a powerful feature of the .NET framework that enables developers to query and manipulate data using a unified syntax directly within C# or other .NET languages. LINQ provides a consistent and expressive way to work with data from various sources, improving developer productivity and code maintainability.
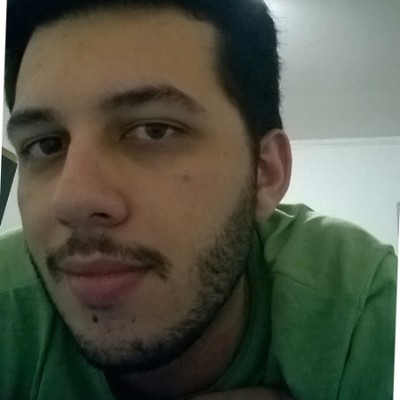
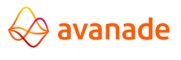