Advanced UI Techniques in Flutter: Custom Painters and Effects
Flutter is renowned for its flexibility and ease of use in creating beautiful UIs. For developers looking to push the boundaries of what’s possible, Flutter’s `CustomPainter` class offers powerful capabilities for creating intricate and bespoke designs. This article delves into advanced UI techniques in Flutter, focusing on how to leverage `CustomPainter` and other effects to elevate your application’s appearance.
Understanding Flutter’s Custom Painter
`CustomPainter` is a core component in Flutter’s rendering pipeline, allowing developers to draw directly onto the canvas with complete control over the painting process. This class is ideal for creating custom graphics, animations, and complex UI elements.
Using `CustomPainter` for Custom Drawings
To start using `CustomPainter`, you need to extend the `CustomPainter` class and override the `paint` method, where you can use the `Canvas` and `Paint` classes to draw shapes and effects.
Example: Drawing a Custom Circular Progress Indicator
```dart import 'package:flutter/material.dart'; class CircularProgressPainter extends CustomPainter { final double progress; CircularProgressPainter(this.progress); @override void paint(Canvas canvas, Size size) { final paint = Paint() ..color = Colors.blue ..style = PaintingStyle.stroke ..strokeWidth = 8.0; final rect = Rect.fromLTWH(0, 0, size.width, size.height); final sweepAngle = 2 * 3.141592653589793 * (progress / 100); canvas.drawArc(rect, -3.141592653589793 / 2, sweepAngle, false, paint); } @override bool shouldRepaint(CustomPainter oldDelegate) { return oldDelegate != this; } } class CircularProgressIndicator extends StatelessWidget { final double progress; CircularProgressIndicator(this.progress); @override Widget build(BuildContext context) { return CustomPaint( size: Size(100, 100), painter: CircularProgressPainter(progress), ); } } ```
Implementing Advanced Effects
Beyond basic drawings, you can use Flutter’s `CustomPainter` to implement advanced effects like gradients, shadows, and animations.
Example: Applying a Gradient to a Custom Shape
```dart import 'package:flutter/material.dart'; class GradientPainter extends CustomPainter { @override void paint(Canvas canvas, Size size) { final paint = Paint() ..shader = LinearGradient( colors: [Colors.red, Colors.blue], begin: Alignment.topLeft, end: Alignment.bottomRight, ).createShader(Rect.fromLTWH(0, 0, size.width, size.height)); canvas.drawRect(Rect.fromLTWH(0, 0, size.width, size.height), paint); } @override bool shouldRepaint(CustomPainter oldDelegate) { return false; } } class GradientBox extends StatelessWidget { @override Widget build(BuildContext context) { return CustomPaint( size: Size(200, 200), painter: GradientPainter(), ); } } ```
Creating Animations with Custom Painters
Animations can be seamlessly integrated into custom drawings by combining `CustomPainter` with Flutter’s animation framework.
Example: Animated Radial Progress Bar
```dart import 'package:flutter/material.dart'; class AnimatedProgressPainter extends CustomPainter { final double progress; final Animation<double> animation; AnimatedProgressPainter(this.progress, this.animation) : super(repaint: animation); @override void paint(Canvas canvas, Size size) { final paint = Paint() ..color = Colors.green ..style = PaintingStyle.stroke ..strokeWidth = 8.0; final rect = Rect.fromLTWH(0, 0, size.width, size.height); final sweepAngle = 2 * 3.141592653589793 * (progress / 100); canvas.drawArc(rect, -3.141592653589793 / 2, sweepAngle * animation.value, false, paint); } @override bool shouldRepaint(CustomPainter oldDelegate) { return true; } } class AnimatedProgress extends StatefulWidget { @override _AnimatedProgressState createState() => _AnimatedProgressState(); } class _AnimatedProgressState extends State<AnimatedProgress> with SingleTickerProviderStateMixin { AnimationController _controller; Animation<double> _animation; @override void initState() { super.initState(); _controller = AnimationController( duration: Duration(seconds: 2), vsync: this, )..repeat(); _animation = Tween<double>(begin: 0, end: 1).animate(_controller); } @override Widget build(BuildContext context) { return CustomPaint( size: Size(100, 100), painter: AnimatedProgressPainter(75, _animation), ); } @override void dispose() { _controller.dispose(); super.dispose(); } } ```
Integrating Custom Painters with Widgets
Custom painters can be combined with standard Flutter widgets to create complex UI components.
Example: Custom Header Widget with a Background
```dart import 'package:flutter/material.dart'; class CustomHeader extends StatelessWidget { @override Widget build(BuildContext context) { return Stack( children: [ CustomPaint( size: Size(double.infinity, 200), painter: GradientPainter(), ), Positioned( top: 80, left: 20, child: Text( 'Custom Header', style: TextStyle(color: Colors.white, fontSize: 32), ), ), ], ); } } ```
Conclusion
Flutter’s `CustomPainter` class opens up a world of possibilities for creating custom, high-performance UIs. From basic shapes and gradients to advanced animations and interactive designs, mastering these techniques can greatly enhance the visual appeal and functionality of your Flutter applications.
Further Reading:
Table of Contents
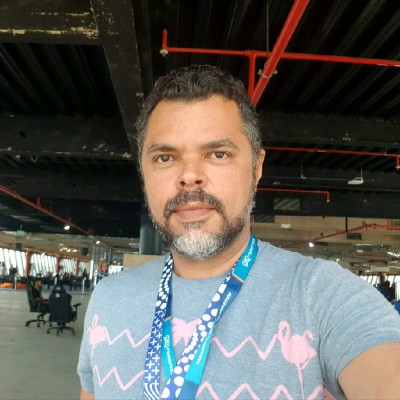
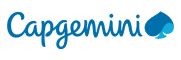