Using Flutter with ARCore: Building Augmented Reality Experiences
Augmented Reality (AR) has transformed the way we interact with digital content, seamlessly blending the virtual world with the real world. With the advent of powerful frameworks like ARCore and flexible UI frameworks like Flutter, creating captivating AR experiences has become more accessible than ever. In this blog post, we will dive into the world of AR development by harnessing the capabilities of Flutter and ARCore to build immersive AR applications. Whether you’re a seasoned developer or just starting, this guide will provide you with the insights and code samples you need to embark on your AR journey.
Table of Contents
1. Understanding Augmented Reality and ARCore
1.1. What is Augmented Reality?
Augmented Reality is a technology that overlays digital content such as 3D models, images, and animations onto the real world. This creates a mixed reality experience where virtual elements seamlessly integrate with the physical environment.
1.2. Introducing ARCore
ARCore is Google’s platform for building augmented reality experiences on Android devices. It provides tools and APIs for motion tracking, environmental understanding, and interaction with virtual objects. Combining ARCore’s capabilities with Flutter’s expressive UI framework opens up new possibilities for creating immersive AR apps.
2. Setting Up Your Development Environment
2.1. Installing Flutter
Before you can start building AR experiences with Flutter, you need to set up your development environment. Install Flutter by following the official documentation for your platform.
2.2. Adding ARCore to Your Flutter Project
To integrate ARCore with your Flutter project, you’ll need to use the arcore_flutter_plugin. Add this package to your pubspec.yaml file:
yaml dependencies: arcore_flutter_plugin: ^latest_version
Run flutter pub get to install the package.
3. Building Your First AR Flutter App
3.1. Creating the UI Layout
Start by creating the UI for your AR app using Flutter’s widgets. You can use a Scaffold with various UI components to create a visually appealing layout.
dart import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'AR Flutter App', home: MyARPage(), ); } } class MyARPage extends StatefulWidget { @override _MyARPageState createState() => _MyARPageState(); } class _MyARPageState extends State<MyARPage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('AR Flutter App'), ), body: Center( child: ARWidget(), // Your AR content widget ), ); } } class ARWidget extends StatelessWidget { @override Widget build(BuildContext context) { // Your AR content goes here return Text('AR Content Placeholder'); } }
3.2. Initializing ARCore
Next, initialize ARCore within your AR content widget. You’ll need to import the necessary ARCore package and use its APIs to set up the AR session.
dart import 'package:arcore_flutter_plugin/arcore_flutter_plugin.dart'; class ARWidget extends StatefulWidget { @override _ARWidgetState createState() => _ARWidgetState(); } class _ARWidgetState extends State<ARWidget> { late ARCoreController arCoreController; @override void dispose() { arCoreController.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return ARCoreView( onARCoreViewCreated: _onARCoreViewCreated, ); } void _onARCoreViewCreated(ARCoreController controller) { arCoreController = controller; // Set up your AR session here } }
3.3. Placing Objects in the AR Scene
With ARCore initialized, you can start placing objects in the AR scene. ARCore uses anchors to position and orient objects in the real world.
dart void _onARCoreViewCreated(ARCoreController controller) { arCoreController = controller; // Create an anchor at the origin (0, 0, 0) final anchor = Anchor( pose: Pose.translation( vector.Vector3(0, 0, 0), ), isTrackable: true, ); // Load a 3D model and attach it to the anchor arCoreController.addArCoreNode( ArCoreReferenceNode( name: 'MyModel', objectUrl: 'path_to_your_3d_model.glb', position: vector.Vector3(0, 0, 0), scale: vector.Vector3(0.1, 0.1, 0.1), rotation: vector.Vector3(0, 0, 0), isOpaque: false, isShadowReceiver: false, isShadowCaster: false, // Other properties ), parent: anchor, ); }
4. Interacting with AR Objects
4.1. Detecting Tap Gestures
Interaction is a crucial part of any AR experience. You can detect tap gestures on AR objects to trigger actions or animations.
dart class _ARWidgetState extends State<ARWidget> { // ... (previous code) @override void _onTapArPlanePlane( List<ArCoreHitTestResult> hits, vector.Vector3? planeCenter, vector.Plane plane, ) { if (hits.isNotEmpty) { // Handle tap gesture on AR plane final hit = hits.first; // Perform your action here } } @override Widget build(BuildContext context) { return ARCoreView( onARCoreViewCreated: _onARCoreViewCreated, enableTapRecognizer: true, onTap: _onTapArPlanePlane, ); } }
4.2. Animating AR Objects
Animating AR objects can bring life to your AR experience. You can use Flutter’s animation framework to smoothly animate object properties.
dart class _ARWidgetState extends State<ARWidget> with SingleTickerProviderStateMixin { late AnimationController _animationController; @override void initState() { super.initState(); _animationController = AnimationController( vsync: this, duration: Duration(seconds: 2), )..repeat(reverse: true); } @override void dispose() { _animationController.dispose(); super.dispose(); } // ... (other code) @override Widget build(BuildContext context) { return ARCoreView( onARCoreViewCreated: _onARCoreViewCreated, enableTapRecognizer: true, onTap: _onTapArPlanePlane, // Other properties ); } void _onARCoreViewCreated(ARCoreController controller) { arCoreController = controller; // ... (other code) arCoreController.addArCoreNode( ArCoreReferenceNode( // ... (other properties) rotation: vector.Vector3( 0, _animationController.value * 360, // Rotate around Y-axis 0, ), // ... (other properties) ), parent: anchor, ); } }
4.3. Adding Realism with Lighting and Shadows
Lighting and shadows play a crucial role in creating realistic AR scenes. ARCore allows you to add light sources to your AR scene.
dart void _onARCoreViewCreated(ARCoreController controller) { arCoreController = controller; // ... (other code) // Add a light source to the scene final light = ArCoreLight( type: ArCoreLightType.directional, color: Colors.white, ); arCoreController.addArCoreNode( light, // ... (other properties) ); // ... (other code) }
5. Implementing Motion Tracking and Environmental Understanding
5.1. Tracking Device Movement
ARCore provides accurate motion tracking, allowing virtual objects to stay anchored in the real world as the user moves the device.
dart class _ARWidgetState extends State<ARWidget> { @override void _onArCoreViewCreated(ARCoreController controller) { arCoreController = controller; // Enable motion tracking arCoreController.startImageStream((images) { // Process device camera images for motion tracking }); // ... (other code) } // ... (other code) }
5.2. Understanding the Environment
ARCore also enables your app to understand the physical environment by detecting planes and other real-world features.
dart class _ARWidgetState extends State<ARWidget> { @override void _onArCoreViewCreated(ARCoreController controller) { arCoreController = controller; // Enable environmental understanding arCoreController.onPlaneTap = (plane) { // Handle tap on detected plane }; // ... (other code) } // ... (other code) }
6. Advanced AR Experiences with Flutter and ARCore
6.1. Adding Surface Detection
Surface detection allows your app to find and interact with real-world surfaces. This is essential for placing AR objects accurately.
dart class _ARWidgetState extends State<ARWidget> { @override void _onArCoreViewCreated(ARCoreController controller) { arCoreController = controller; // Enable surface detection arCoreController.onPlaneTap = (plane) { // Handle tap on detected plane arCoreController.addArCoreNode( // ... (AR node properties) parent: plane.anchor, ); }; // ... (other code) } // ... (other code) }
6.2. Incorporating User-Generated Content
Empower users to create their own AR experiences by enabling them to add and interact with their 3D models.
dart class _ARWidgetState extends State<ARWidget> { @override void _onArCoreViewCreated(ARCoreController controller) { arCoreController = controller; // ... (other code) arCoreController.onPlaneTap = (plane) { // Prompt user to select a 3D model // Load the selected model and attach it to the plane anchor }; // ... (other code) } // ... (other code) }
6.3. Multi-user AR Experiences
Take AR experiences to the next level by enabling multi-user interactions, allowing users to collaborate in the same AR environment.
dart class _ARWidgetState extends State<ARWidget> { late ARCoreController arCoreController; late List<ArCoreNode> arNodes = []; // ... (other code) @override void _onArCoreViewCreated(ARCoreController controller) { arCoreController = controller; arCoreController.onPlaneTap = (plane) { final newNode = ArCoreReferenceNode( // ... (AR node properties) parent: plane.anchor, ); setState(() { arNodes.add(newNode); }); }; } @override Widget build(BuildContext context) { return ARCoreView( onARCoreViewCreated: _onARCoreViewCreated, enableTapRecognizer: true, onTap: _onTapArPlanePlane, children: arNodes, ); } // ... (other code) }
7. Enhancing Performance and Optimization
7.1. Optimizing 3D Models
To ensure smooth performance, optimize your 3D models for mobile devices. Reduce polygon count, texture sizes, and use formats like GLB for efficient loading.
7.2. Managing Memory and Resources
AR apps can be resource-intensive. Properly manage memory and release resources when they are no longer needed to prevent performance issues.
7.3. Handling AR Session Lifecycle
Handle the AR session lifecycle events, such as pausing and resuming, to ensure a seamless experience for users.
Conclusion
Flutter and ARCore open up a world of possibilities for building immersive augmented reality experiences. By combining Flutter’s expressive UI framework with ARCore’s powerful capabilities, developers can create engaging AR apps that seamlessly integrate digital content with the real world. Whether you’re interested in building simple AR interactions or diving into advanced multi-user experiences, this guide provides you with the foundation and code samples to get started on your AR journey. Embrace the future of technology by creating captivating AR applications that bring together the virtual and physical worlds. Happy coding!
Table of Contents
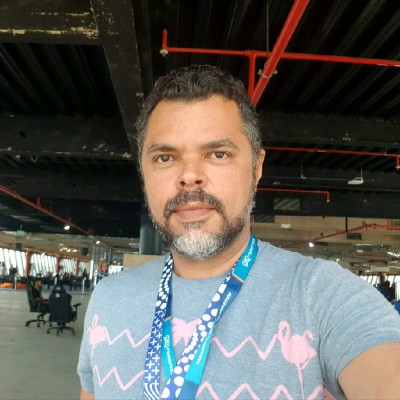
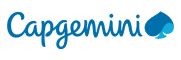