Flutter for ARKit: Building AR Experiences for iOS
Augmented Reality (AR) has revolutionized how we interact with the digital world, providing immersive experiences that blend the real and virtual realms. With Flutter’s cross-platform capabilities and ARKit’s powerful AR framework, developers can create compelling AR applications for iOS. This blog explores how to harness Flutter for building AR experiences using ARKit, complete with practical examples and integration tips.
Understanding Augmented Reality with ARKit
ARKit is Apple’s framework for creating augmented reality experiences on iOS. It enables the integration of virtual objects into the real world by leveraging device sensors and cameras. Flutter, a popular cross-platform framework, can work seamlessly with ARKit to build high-quality AR applications for iOS.
Using Flutter for ARKit Integration
While Flutter doesn’t natively support ARKit, you can use platform channels to bridge Flutter with ARKit’s native capabilities. This section covers the key aspects and code examples for integrating ARKit with Flutter.
1. Setting Up ARKit in a Flutter Project
To start using ARKit with Flutter, you need to set up a Flutter project and add ARKit support using platform channels.
Example: Configuring ARKit with Flutter
1. Create a Flutter Project:
```bash flutter create arkit_flutter_example cd arkit_flutter_example ```
2. Add Dependencies:
Add the `arkit_flutter` plugin to your `pubspec.yaml` file to interface with ARKit.
```yaml dependencies: flutter: sdk: flutter arkit_flutter: ^0.7.0 ```
3. Configure iOS for ARKit:
Update the `Info.plist` file to include ARKit permissions and capabilities:
```xml <key>NSCameraUsageDescription</key> <string>We need access to the camera for AR experiences.</string> <key>ARKit</key> <true/> ```
2. Building a Basic AR Experience
Here’s how you can build a basic AR experience using Flutter and ARKit. This example demonstrates placing a virtual object in an AR scene.
Example: Adding a 3D Object to an AR Scene
```dart import 'package:flutter/material.dart'; import 'package:arkit_flutter/arkit_flutter.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: ARKitExample(), ); } } class ARKitExample extends StatefulWidget { @override _ARKitExampleState createState() => _ARKitExampleState(); } class _ARKitExampleState extends State<ARKitExample> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('ARKit with Flutter')), body: ARKitSceneView( onARKitViewCreated: _onARKitViewCreated, ), ); } void _onARKitViewCreated(ARKitController arkitController) { final node = ARKitNode( geometry: ARKitSphere(radius: 0.1), position: Vector3(0, 0, -0.5), materials: [ARKitMaterial(diffuse: ARKitMaterialColorProperty(color: Colors.blue))], ); arkitController.add(node); } } ```
3. Handling AR Interactions
To create interactive AR experiences, you need to handle user interactions, such as tapping on virtual objects.
Example: Responding to Tap Gestures
```dart void _onARKitViewCreated(ARKitController arkitController) { final node = ARKitNode( geometry: ARKitSphere(radius: 0.1), position: Vector3(0, 0, -0.5), materials: [ARKitMaterial(diffuse: ARKitMaterialColorProperty(color: Colors.blue))], ); arkitController.add(node); arkitController.onNodeTap = (nodes) { for (var node in nodes) { print('Tapped on node: ${node.name}'); // Handle interaction } }; } ```
4. Visualizing AR Data
Visualizing AR data involves creating overlays and annotations in the AR scene. Flutter and ARKit allow you to render text, images, or custom graphics.
Example: Adding Text Overlays in AR
```dart void _onARKitViewCreated(ARKitController arkitController) { final text = ARKitText( text: 'Hello AR', extrusionDepth: 1, materials: [ARKitMaterial(diffuse: ARKitMaterialColorProperty(color: Colors.red))], ); final node = ARKitNode( geometry: text, position: Vector3(0, 0, -0.5), rotation: Vector4(1, 0, 0, 0), ); arkitController.add(node); } ```
Conclusion
Flutter, combined with ARKit, opens up exciting possibilities for creating augmented reality applications on iOS. By leveraging Flutter’s cross-platform capabilities and ARKit’s powerful AR features, developers can build immersive and engaging AR experiences. Whether you’re adding simple 3D objects, handling user interactions, or visualizing data, Flutter and ARKit provide the tools needed for effective AR development.
Further Reading:
Table of Contents
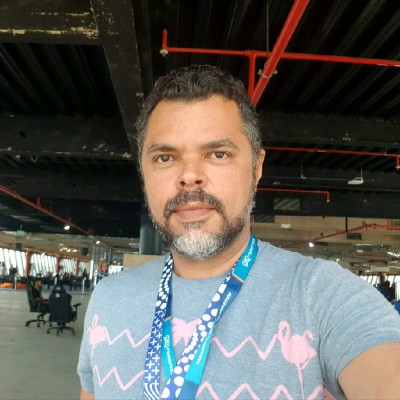
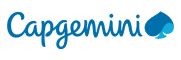