Flutter and Bluetooth: Connecting Devices in Your App
In the ever-expanding world of mobile app development, the ability to connect to external hardware and devices has become increasingly important. Bluetooth technology plays a pivotal role in this, allowing your Flutter app to interact with various devices such as fitness trackers, IoT devices, and even smart home appliances. In this comprehensive guide, we’ll explore how to harness the power of Bluetooth to seamlessly connect devices to your Flutter app, opening up a world of possibilities for your projects.
Table of Contents
1. Understanding Bluetooth Connectivity
Before we dive into the code, it’s essential to grasp the fundamentals of Bluetooth connectivity. Bluetooth, a wireless communication protocol, enables devices to exchange data over short distances. There are two primary roles in a Bluetooth connection:
1.1. Peripheral
A peripheral device typically provides data or services to other devices.
Examples include heart rate monitors, temperature sensors, and smart locks.
In the context of Flutter, your app often takes on the role of a central device that interacts with peripheral devices.
1.2. Central
A central device initiates connections and requests data or services from peripherals.
Your Flutter app will assume the central role when connecting to Bluetooth devices.
Now that we’ve established the basic concepts, let’s jump into the steps required to connect Bluetooth devices to your Flutter app.
2. Setting Up Your Flutter Project
To get started, make sure you have Flutter installed on your system. If you haven’t already, you can install Flutter by following the official documentation.
Once Flutter is up and running, create a new Flutter project or open an existing one that you want to enhance with Bluetooth functionality.
3. Adding Bluetooth Package to Your Flutter Project
Flutter provides a range of packages to simplify the integration of Bluetooth into your app. One of the most popular packages is flutter_blue. To add it to your project, include the following dependency in your pubspec.yaml file:
yaml dependencies: flutter: sdk: flutter flutter_blue: ^0.7.0
After adding the dependency, run flutter pub get in your terminal to fetch and install the package.
4. Scanning for Nearby Bluetooth Devices
To connect to a Bluetooth device, your app needs to discover and establish a connection with it. Here’s how you can scan for nearby Bluetooth devices using the flutter_blue package:
dart import 'package:flutter/material.dart'; import 'package:flutter_blue/flutter_blue.dart'; class BluetoothScanner extends StatefulWidget { @override _BluetoothScannerState createState() => _BluetoothScannerState(); } class _BluetoothScannerState extends State<BluetoothScanner> { FlutterBlue flutterBlue = FlutterBlue.instance; List<BluetoothDevice> devices = []; @override void initState() { super.initState(); _startScanning(); } void _startScanning() { flutterBlue.scanResults.listen((results) { for (ScanResult result in results) { if (!devices.contains(result.device)) { setState(() { devices.add(result.device); }); } } }); flutterBlue.startScan(); } @override void dispose() { flutterBlue.stopScan(); super.dispose(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Bluetooth Devices'), ), body: ListView.builder( itemCount: devices.length, itemBuilder: (context, index) { return ListTile( title: Text(devices[index].name), subtitle: Text(devices[index].id.toString()), onTap: () { // Connect to the selected device here }, ); }, ), ); } }
In this code snippet, we create a BluetoothScanner widget that scans for nearby Bluetooth devices using flutter_blue. The discovered devices are displayed in a ListView. When a device is tapped, you can add the logic to connect to it.
5. Establishing a Connection
Once you’ve identified the Bluetooth device you want to connect to, you can establish a connection. Here’s how you can do it:
dart void _connectToDevice(BluetoothDevice device) async { try { await device.connect(); // Device connected successfully } catch (e) { // Handle connection errors print('Error: $e'); } }
In the onTap handler of your device list, call the _connectToDevice function to initiate the connection.
6. Discovering Services and Characteristics
After successfully connecting to a Bluetooth device, the next step is to discover the services and characteristics it offers. Services are logical groupings of functionality, while characteristics are data points within those services. You can think of characteristics as attributes that you can read from or write data to.
dart void _discoverServices(BluetoothDevice device) async { List<BluetoothService> services = await device.discoverServices(); for (BluetoothService service in services) { // Process each service print('Service: ${service.uuid}'); for (BluetoothCharacteristic characteristic in service.characteristics) { // Process each characteristic print('Characteristic: ${characteristic.uuid}'); } } }
In the code above, we use the discoverServices method to retrieve the list of services offered by the connected device. Then, we iterate through each service and its characteristics, allowing us to interact with the device’s functionality.
7. Reading and Writing Data
Now that you’ve discovered the characteristics of a service, you can read data from or write data to these characteristics. Here’s how you can read data from a characteristic:
dart void _readCharacteristic(BluetoothCharacteristic characteristic) async { List<int> value = await characteristic.read(); print('Read value: $value'); }
In this code, we use the read method to retrieve the current value of the characteristic.
To write data to a characteristic, you can use the write method:
dart void _writeCharacteristic(BluetoothCharacteristic characteristic, List<int> data) async { await characteristic.write(data); print('Data written successfully'); }
These are the fundamental operations you’ll perform when communicating with Bluetooth devices. Reading and writing data enable you to control and monitor various features and functions of the connected device.
8. Handling Notifications
Some Bluetooth devices send notifications when specific events occur. To receive these notifications, you can set up a listener on a characteristic:
dart void _setupNotifications(BluetoothCharacteristic characteristic) async { await characteristic.setNotifyValue(true); characteristic.value.listen((value) { // Handle notifications here print('Notification received: $value'); }); }
In this code, we enable notifications on a characteristic using setNotifyValue(true), and then we listen for incoming notifications using the value stream. This allows your Flutter app to react in real-time to events generated by the Bluetooth device.
9. Handling Disconnects
It’s crucial to handle disconnects gracefully in your Flutter app. When a device disconnects unexpectedly or intentionally, you should clean up resources and provide appropriate feedback to the user. Here’s an example of how to handle disconnects:
dart void _handleDisconnect(BluetoothDevice device) { device.state.listen((event) { if (event == BluetoothDeviceState.disconnected) { // Device disconnected, perform cleanup print('Device disconnected'); } }); }
By listening to the state stream of the BluetoothDevice, you can detect when a device disconnects and trigger any necessary actions.
Conclusion
Integrating Bluetooth functionality into your Flutter app opens up a world of possibilities, from creating fitness tracking apps to controlling IoT devices. By following the steps outlined in this guide, you can seamlessly connect and communicate with Bluetooth devices, providing your users with a richer and more interactive experience.
Remember that Bluetooth connectivity can vary between different devices and manufacturers. Always consult the documentation provided by the device manufacturer to ensure a smooth integration process. With the right approach and the power of Flutter, you can create innovative apps that leverage the capabilities of Bluetooth technology to the fullest. So, go ahead and start building your next Bluetooth-enabled Flutter app today!
Table of Contents
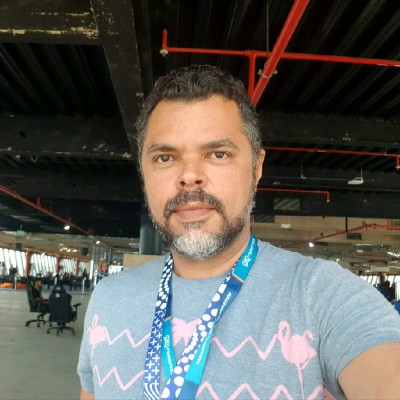
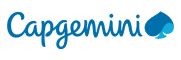