Creating a Budgeting App with Flutter: Expense Tracking and Analytics
Flutter is an excellent framework for building cross-platform apps, and creating a budgeting app with it can provide users with a powerful tool for managing their finances. This article will guide you through the process of building a budgeting app using Flutter, emphasizing expense tracking and analytics to offer valuable insights into financial management.
Understanding Budgeting Apps
Budgeting apps help users track their expenses, manage their budgets, and analyze their spending habits. By leveraging Flutter’s capabilities, you can create an app that not only tracks expenses but also provides insightful analytics to help users make informed financial decisions.
Building Your Budgeting App with Flutter
Flutter offers a range of features and packages to help you build a fully functional budgeting app. Here are key aspects and examples to guide you through the development process.
1. Setting Up Your Flutter Project
Before diving into specific features, set up your Flutter project to create a solid foundation for your app.
Example: Setting Up a New Flutter Project
- Create a New Project
Open your terminal and run:
```bash flutter create budgeting_app ```
- Navigate to Your Project Directory
```bash cd budgeting_app ```
- Open the Project in Your Preferred IDE
Open the project in Visual Studio Code, Android Studio, or another IDE of your choice.
2. Implementing Expense Tracking
Expense tracking is a core feature of any budgeting app. Flutter provides various packages to facilitate expense tracking.
Example: Using the [sqflite](https://pub.dev/packages/sqflite) Package for Local Storage
- Add Dependencies
Add `sqflite` and `path` packages to your `pubspec.yaml`:
```yaml dependencies: flutter: sdk: flutter sqflite: ^2.0.0+4 path: ^1.8.0 ```
- Create a Database Helper
Develop a class to handle database operations, such as adding, retrieving, and deleting expenses.
```dart import 'package:sqflite/sqflite.dart'; import 'package:path/path.dart'; class DatabaseHelper { static final _databaseName = "budgeting_app.db"; static final _databaseVersion = 1; static final table = 'expenses'; static final columnId = '_id'; static final columnName = 'name'; static final columnAmount = 'amount'; static final columnDate = 'date'; DatabaseHelper._privateConstructor(); static final DatabaseHelper instance = DatabaseHelper._privateConstructor(); static Database? _database; Future<Database> get database async { if (_database != null) return _database!; _database = await _initDatabase(); return _database!; } Future<Database> _initDatabase() async { return await openDatabase( join(await getDatabasesPath(), _databaseName), version: _databaseVersion, onCreate: _onCreate, ); } Future _onCreate(Database db, int version) async { await db.execute(''' CREATE TABLE $table ( $columnId INTEGER PRIMARY KEY, $columnName TEXT NOT NULL, $columnAmount REAL NOT NULL, $columnDate TEXT NOT NULL ) '''); } // Insert, query, update, and delete methods... } ```
3. Creating Analytics and Reports
Analytics help users understand their spending habits and budget more effectively. Flutter can integrate with various charting libraries to visualize this data.
Example: Using the [fl_chart](https://pub.dev/packages/fl_chart) Package for Visualization
- Add the `fl_chart` Dependency
Include the `fl_chart` package in your `pubspec.yaml`:
```yaml dependencies: fl_chart: ^0.40.0 ```
- Create Charts
Use `fl_chart` to display expense data in pie charts or bar charts.
```dart import 'package:fl_chart/fl_chart.dart'; class ExpenseChart extends StatelessWidget { final List<Expense> expenses; ExpenseChart({required this.expenses}); @override Widget build(BuildContext context) { return PieChart( PieChartData( sections: _createChartSections(), ), ); } List<PieChartSectionData> _createChartSections() { // Create chart sections based on expenses... } } ```
4. Implementing Budget Alerts
Alerts can notify users when they approach or exceed their budget limits.
Example: Using Flutter’s Local Notifications Package
- Add the `flutter_local_notifications` Dependency
Add the package to your `pubspec.yaml`:
“`yaml
dependencies:
flutter_local_notifications: ^13.0.0
“`
- Configure Notifications
Set up notifications to alert users when they exceed their budget.
```dart import 'package:flutter_local_notifications/flutter_local_notifications.dart'; class NotificationService { final FlutterLocalNotificationsPlugin _flutterLocalNotificationsPlugin = FlutterLocalNotificationsPlugin(); Future<void> _initializeNotifications() async { final initializationSettings = InitializationSettings( android: AndroidInitializationSettings('@mipmap/ic_launcher'), iOS: IOSInitializationSettings(), ); await _flutterLocalNotificationsPlugin.initialize(initializationSettings); } Future<void> _showNotification(String title, String body) async { const androidPlatformChannelSpecifics = AndroidNotificationDetails( 'channel_id', 'channel_name', importance: Importance.max, priority: Priority.high, ); const platformChannelSpecifics = NotificationDetails( android: androidPlatformChannelSpecifics, ); await _flutterLocalNotificationsPlugin.show(0, title, body, platformChannelSpecifics); } } ```
Conclusion
Creating a budgeting app with Flutter offers a powerful way to help users manage their finances. By implementing features like expense tracking, analytics, and budget alerts, you can build an app that provides valuable insights and improves financial management.
Further Reading:
Table of Contents
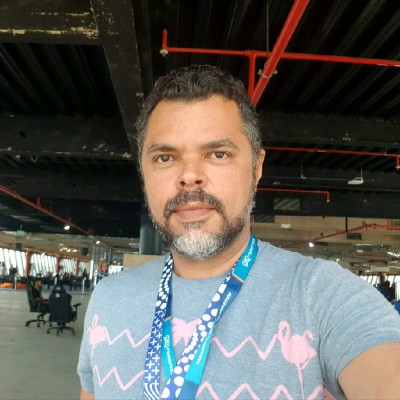
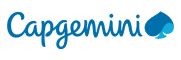