Building a Social Media App with Flutter: UI and Functionality
In today’s digital age, social media platforms have become an integral part of our lives. The ability to connect, share, and communicate with others seamlessly has transformed the way we interact. If you’re an aspiring app developer looking to dive into the world of social media, this tutorial is for you. We’ll guide you through the process of building a social media app using Flutter, a popular open-source UI software development kit.
1. Introduction to Flutter and its Advantages
Flutter, developed by Google, is a powerful framework for building natively compiled applications for mobile, web, and desktop from a single codebase. Its key advantage lies in its expressive and flexible UI components, which enable developers to create visually appealing and responsive interfaces. With Flutter’s “hot reload” feature, developers can see changes instantly, making the development process efficient and iterative.
2. Setting Up Your Development Environment
Before diving into the app development process, make sure you have Flutter and Dart installed on your machine. You can follow the official Flutter installation guide for your specific operating system. Once installed, verify your installation using the flutter doctor command in your terminal. This command will help you identify any missing dependencies.
3. Designing the User Interface
A captivating user interface is crucial for any social media app’s success. Flutter provides a wide range of widgets and tools to create stunning UIs. Let’s break down the UI design into key components.
3.1 Creating the Login and Signup Screens
The first interaction users have with your app is the login and signup process. Design a user-friendly and visually appealing login and signup screen using Flutter’s TextField, Button, and Image widgets. Here’s a snippet of code for reference:
dart class LoginScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Login')), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Image.asset('assets/logo.png'), SizedBox(height: 20), TextField( decoration: InputDecoration(labelText: 'Username'), ), TextField( decoration: InputDecoration(labelText: 'Password'), obscureText: true, ), SizedBox(height: 20), ElevatedButton( onPressed: () { // Implement login logic }, child: Text('Login'), ), TextButton( onPressed: () { // Navigate to signup screen }, child: Text('Create an account'), ), ], ), ), ); } }
3.2 Designing the News Feed
The heart of any social media app is the news feed, where users can scroll through posts and updates from people they follow. Use Flutter’s ListView widget to create a scrollable feed. Each post can be designed using Card and various other widgets. Here’s a simplified example:
dart class NewsFeedScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('News Feed')), body: ListView.builder( itemCount: posts.length, itemBuilder: (context, index) { return Card( child: ListTile( leading: CircleAvatar( backgroundImage: NetworkImage(posts[index].userAvatar), ), title: Text(posts[index].username), subtitle: Text(posts[index].timestamp), content: Text(posts[index].content), // Add like, comment, and share buttons ), ); }, ), ); } }
3.3 Building User Profiles
User profiles allow users to showcase their information and posts. Design a user profile screen that displays the user’s avatar, username, bio, and posts. You can use a combination of widgets like Column, Row, and Container to achieve the desired layout.
dart class UserProfileScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('User Profile')), body: Column( children: [ CircleAvatar( backgroundImage: NetworkImage(currentUser.avatar), ), SizedBox(height: 10), Text(currentUser.username), Text(currentUser.bio), // Display user's posts using ListView or GridView ], ), ); } }
4. Implementing Functionality
A social media app is more than just a pretty UI – it needs to have robust functionality. Let’s explore how to implement essential features using Flutter.
4.1 User Authentication and Authorization
User authentication is the foundation of your app’s security. Implement authentication using packages like firebase_auth or your preferred authentication solution. Once a user is authenticated, manage authorization to ensure users can access the right content and actions.
dart Future<void> signInWithEmailAndPassword(String email, String password) async { try { UserCredential userCredential = await FirebaseAuth.instance.signInWithEmailAndPassword( email: email, password: password, ); // User is signed in } catch (e) { // Handle authentication errors } }
4.2 Creating and Posting Content
Allow users to create and post content to their profiles or the news feed. Use Flutter’s TextFormField or other input widgets to capture user-generated content and images. Upon submission, update the relevant database or API with the new content.
dart class CreatePostScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Create Post')), body: Column( children: [ TextFormField( maxLines: 5, decoration: InputDecoration(labelText: 'Write something...'), ), // Add image upload functionality ElevatedButton( onPressed: () { // Implement post creation logic }, child: Text('Post'), ), ], ), ); } }
4.3 Implementing the Follow System
The follow system allows users to connect with others and see their posts in their news feed. Create a function to toggle a user’s follow status and update the database accordingly.
dart void toggleFollow(UserProfile user) { // Toggle user's follow status and update database if (currentUser.following.contains(user)) { currentUser.following.remove(user); } else { currentUser.following.add(user); } }
4.4 Adding Likes, Comments, and Shares
Enhance user engagement by allowing likes, comments, and shares on posts. Implement these features using Flutter’s IconButton or similar widgets.
dart class PostItem extends StatelessWidget { final Post post; PostItem(this.post); @override Widget build(BuildContext context) { return Card( child: ListTile( // ... trailing: Row( mainAxisSize: MainAxisSize.min, children: [ IconButton( icon: Icon(Icons.thumb_up), onPressed: () { // Implement like functionality }, ), IconButton( icon: Icon(Icons.comment), onPressed: () { // Implement comment functionality }, ), IconButton( icon: Icon(Icons.share), onPressed: () { // Implement share functionality }, ), ], ), ), ); } }
5. Connecting to Backend Services
To store and retrieve user data, posts, and interactions, connect your app to a backend service. You can use Firebase, REST APIs, or GraphQL, depending on your preferences.
6. Testing and Debugging Your App
Throughout the development process, regularly test your app on different devices and screen sizes. Use Flutter’s built-in debugging tools to identify and fix any issues that arise. Utilize the “hot reload” feature to quickly see changes in action.
Conclusion
Building a social media app with Flutter is an exciting journey that combines creative UI design with powerful functionality implementation. By following the steps outlined in this tutorial, you’re well on your way to creating a captivating social media experience for your users. Remember to continuously iterate, test, and gather user feedback to refine your app and deliver a seamless user experience. Happy coding!
Table of Contents
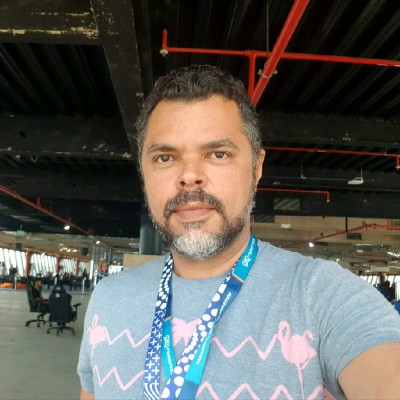
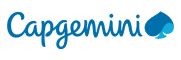