Creating Custom Animations in Flutter: A Step-by-Step Guide
Animations play a pivotal role in enhancing the user experience of mobile applications. They add flair, interactivity, and a sense of dynamism that can turn an ordinary app into an extraordinary one. In Flutter, Google’s UI toolkit for building natively compiled applications for mobile, web, and desktop from a single codebase, creating custom animations is both a rewarding and creative endeavor. In this step-by-step guide, we’ll explore the process of crafting your own custom animations in Flutter, from the basics to more advanced techniques.
1. Understanding the Importance of Custom Animations
Before we dive into the technical details, let’s briefly discuss why custom animations are crucial for a successful app. Animations provide visual cues that guide users through the app’s interface. They can help explain transitions between different views, provide feedback for actions, and make the overall user experience more engaging and intuitive.
2. Getting Started with Flutter Animations
2.1 Animating Widgets
Flutter offers a wide range of animation classes that make it easy to bring your UI elements to life. The AnimatedContainer, AnimatedOpacity, and AnimatedAlign are just a few examples of widgets that can be animated seamlessly. Here’s a quick code snippet illustrating the basic structure of animating a widget:
dart class MyAnimatedWidget extends StatefulWidget { @override _MyAnimatedWidgetState createState() => _MyAnimatedWidgetState(); } class _MyAnimatedWidgetState extends State<MyAnimatedWidget> { double _opacity = 1.0; @override Widget build(BuildContext context) { return GestureDetector( onTap: () { setState(() { _opacity = _opacity == 0.0 ? 1.0 : 0.0; }); }, child: AnimatedOpacity( opacity: _opacity, duration: Duration(milliseconds: 500), child: Container( width: 100, height: 100, color: Colors.blue, ), ), ); } }
2.2 Animation Controllers
To create more complex animations, you’ll need to use Animation Controllers. These controllers provide greater control over the animation process, allowing you to define animation curves, durations, and much more. Here’s an example of using an Animation Controller to create a rotation animation:
dart class RotationAnimation extends StatefulWidget { @override _RotationAnimationState createState() => _RotationAnimationState(); } class _RotationAnimationState extends State<RotationAnimation> with SingleTickerProviderStateMixin { late AnimationController _controller; late Animation<double> _rotationAnimation; @override void initState() { super.initState(); _controller = AnimationController( vsync: this, duration: Duration(seconds: 2), ); _rotationAnimation = Tween(begin: 0.0, end: 1.0).animate(_controller) ..addListener(() { setState(() {}); }); _controller.repeat(); } @override void dispose() { _controller.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return Transform.rotate( angle: _rotationAnimation.value * 2 * pi, child: Container( width: 100, height: 100, color: Colors.orange, ), ); } }
3. Building Basic Custom Animations
3.1 Fade Animation
A simple yet effective animation is the fade animation. This can be achieved using the FadeTransition widget. Here’s how you can create a fade-in animation:
dart class FadeInAnimation extends StatefulWidget { @override _FadeInAnimationState createState() => _FadeInAnimationState(); } class _FadeInAnimationState extends State<FadeInAnimation> with SingleTickerProviderStateMixin { late AnimationController _controller; late Animation<double> _fadeAnimation; @override void initState() { super.initState(); _controller = AnimationController( vsync: this, duration: Duration(seconds: 1), ); _fadeAnimation = Tween(begin: 0.0, end: 1.0).animate(_controller); _controller.forward(); } @override void dispose() { _controller.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return FadeTransition( opacity: _fadeAnimation, child: Container( width: 150, height: 150, color: Colors.green, ), ); } }
3.2 Scale Animation
Scaling animations can add a dynamic feel to your app’s interface. The ScaleTransition widget is your friend here. Let’s see how you can implement a scale animation:
dart class ScaleAnimation extends StatefulWidget { @override _ScaleAnimationState createState() => _ScaleAnimationState(); } class _ScaleAnimationState extends State<ScaleAnimation> with SingleTickerProviderStateMixin { late AnimationController _controller; late Animation<double> _scaleAnimation; @override void initState() { super.initState(); _controller = AnimationController( vsync: this, duration: Duration(seconds: 1), ); _scaleAnimation = Tween(begin: 1.0, end: 2.0).animate(_controller); _controller.forward(); } @override void dispose() { _controller.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return ScaleTransition( scale: _scaleAnimation, child: Container( width: 100, height: 100, color: Colors.purple, ), ); } }
4. Complex Custom Animations
4.1 Animated Opacity
Animating the opacity of a widget can lead to elegant and subtle effects. Flutter provides the AnimatedOpacity widget for this purpose. Here’s an example:
dart class OpacityAnimation extends StatefulWidget { @override _OpacityAnimationState createState() => _OpacityAnimationState(); } class _OpacityAnimationState extends State<OpacityAnimation> { bool _isVisible = true; @override Widget build(BuildContext context) { return Column( mainAxisAlignment: MainAxisAlignment.center, children: [ AnimatedOpacity( opacity: _isVisible ? 1.0 : 0.0, duration: Duration(seconds: 1), child: Container( width: 150, height: 150, color: Colors.blue, ), ), SizedBox(height: 20), ElevatedButton( onPressed: () { setState(() { _isVisible = !_isVisible; }); }, child: Text(_isVisible ? 'Hide' : 'Show'), ), ], ); } }
4.2 Hero Animations
Hero animations are used to create smooth transitions between different screens. They work particularly well when you want to maintain a visual connection between two widgets. Here’s a basic example:
dart class HeroAnimation extends StatelessWidget { @override Widget build(BuildContext context) { return GestureDetector( onTap: () { Navigator.of(context).push(MaterialPageRoute( builder: (context) => HeroDetailScreen(), )); }, child: Hero( tag: 'heroTag', child: Container( width: 100, height: 100, color: Colors.red, ), ), ); } } class HeroDetailScreen extends StatelessWidget { @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Hero( tag: 'heroTag', child: Container( width: 300, height: 300, color: Colors.red, ), ), ), ); } }
5. User Interaction and Gestures in Animations
5.1 Drag and Drop
Incorporating drag-and-drop animations can make your app more interactive. Flutter’s Draggable and DragTarget widgets are essential for achieving this effect. Here’s a simple example:
dart class DragAndDropAnimation extends StatefulWidget { @override _DragAndDropAnimationState createState() => _DragAndDropAnimationState(); } class _DragAndDropAnimationState extends State<DragAndDropAnimation> { bool _isDropped = false; @override Widget build(BuildContext context) { return Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Draggable( child: Container( width: 100, height: 100, color: Colors.yellow, child: Center(child: Text('Drag me')), ), feedback: Container( width: 100, height: 100, color: Colors.yellow.withOpacity(0.7), child: Center(child: Text('Dragging')), ), childWhenDragging: Container( width: 100, height: 100, ), ), SizedBox(height: 20), DragTarget( builder: (context, candidateData, rejectedData) { return Container( width: 150, height: 150, color: _isDropped ? Colors.green : Colors.grey, child: Center(child: Text('Drop here')), ); }, onWillAccept: (data) { return true; }, onAccept: (data) { setState(() { _isDropped = true; }); }, ), ], ); } }
5.2 Gestures for Animation Control
Adding gestures to control animations gives users a sense of control and interactivity. Here’s an example of using swipe gestures to control an animation:
dart class SwipeAnimation extends StatefulWidget { @override _SwipeAnimationState createState() => _SwipeAnimationState(); } class _SwipeAnimationState extends State<SwipeAnimation> with SingleTickerProviderStateMixin { late AnimationController _controller; late Animation<double> _positionAnimation; @override void initState() { super.initState(); _controller = AnimationController( vsync: this, duration: Duration(seconds: 1), ); _positionAnimation = Tween(begin: 0.0, end: 200.0).animate(_controller); _controller.addListener(() { setState(() {}); }); } @override void dispose() { _controller.dispose(); super.dispose(); } void _handleSwipe(DragUpdateDetails details) { _controller.value -= details.primaryDelta! / 200; } @override Widget build(BuildContext context) { return GestureDetector( onVerticalDragUpdate: _handleSwipe, onVerticalDragEnd: (details) { if (_controller.isAnimating || _controller.status == AnimationStatus.completed) { return; } if (details.primaryVelocity! < 0) { _controller.forward(); } else if (details.primaryVelocity! > 0) { _controller.reverse(); } }, child: Transform.translate( offset: Offset(0, _positionAnimation.value), child: Container( width: 100, height: 100, color: Colors.blue, ), ), ); } }
6. Performance Optimization
6.1 Using AnimatedBuilder
Flutter’s AnimatedBuilder widget is a powerful tool for optimizing animations. It allows you to rebuild only a specific part of the widget tree, minimizing unnecessary rebuilds and improving performance. Here’s an example:
dart class AnimatedBuilderExample extends StatefulWidget { @override _AnimatedBuilderExampleState createState() => _AnimatedBuilderExampleState(); } class _AnimatedBuilderExampleState extends State<AnimatedBuilderExample> with SingleTickerProviderStateMixin { late AnimationController _controller; late Animation<double> _fadeAnimation; @override void initState() { super.initState(); _controller = AnimationController( vsync: this, duration: Duration(seconds: 1), ); _fadeAnimation = Tween(begin: 0.0, end: 1.0).animate(_controller); _controller.forward(); } @override void dispose() { _controller.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return Center( child: AnimatedBuilder( animation: _controller, builder: (context, child) { return Opacity( opacity: _fadeAnimation.value, child: Container( width: 150, height: 150, color: Colors.orange, ), ); }, ), ); } }
6.2 Tween vs. CurvedAnimation
When creating animations, you’ll often need to specify the range of values the animation should cover. Flutter provides the Tween class for this purpose. Additionally, you can enhance your animations using CurvedAnimation to apply different easing curves. Here’s an example:
dart class CurvedAnimationExample extends StatefulWidget { @override _CurvedAnimationExampleState createState() => _CurvedAnimationExampleState(); } class _CurvedAnimationExampleState extends State<CurvedAnimationExample> with SingleTickerProviderStateMixin { late AnimationController _controller; late Animation<double> _animation; @override void initState() { super.initState(); _controller = AnimationController( vsync: this, duration: Duration(seconds: 2), ); _animation = CurvedAnimation( parent: _controller, curve: Curves.easeInOut, ); _controller.repeat(reverse: true); } @override void dispose() { _controller.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return Center( child: ScaleTransition( scale: _animation, child: Container( width: 100, height: 100, color: Colors.blue, ), ), ); } }
7. Putting It All Together: Creating an Animated UI Component
Now that you’ve learned the basics of creating custom animations in Flutter, let’s combine these techniques to create a more complex animated UI component. For instance, you can create a button that scales and changes color on tap:
dart class AnimatedButton extends StatefulWidget { @override _AnimatedButtonState createState() => _AnimatedButtonState(); } class _AnimatedButtonState extends State<AnimatedButton> with SingleTickerProviderStateMixin { late AnimationController _controller; late Animation<double> _scaleAnimation; late Animation<Color?> _colorAnimation; @override void initState() { super.initState(); _controller = AnimationController( vsync: this, duration: Duration(milliseconds: 300), ); _scaleAnimation = Tween(begin: 1.0, end: 0.8).animate(_controller); _colorAnimation = ColorTween(begin: Colors.blue, end: Colors.red) .animate(_controller); _controller.addListener(() { setState(() {}); }); _controller.addStatusListener((status) { if (status == AnimationStatus.completed) { _controller.reverse(); } }); } @override void dispose() { _controller.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return GestureDetector( onTapDown: (_) { _controller.forward(); }, onTapUp: (_) { _controller.reverse(); }, child: Transform.scale( scale: _scaleAnimation.value, child: Container( width: 150, height: 50, color: _colorAnimation.value, child: Center( child: Text( 'Tap Me', style: TextStyle(color: Colors.white), ), ), ), ), ); } }
8. Going Beyond: Custom Animations with Rive
While Flutter provides a robust set of animation tools, you can also integrate third-party tools like Rive for more advanced animations. Rive is a popular design and animation tool that allows you to create interactive vector animations. Integrating Rive animations into your Flutter app involves exporting animations from Rive and using the rive package to render them. This enables you to achieve stunning and complex animations with ease.
9. Testing and Debugging Your Animations
As with any code, testing and debugging are crucial when working with animations. Flutter offers various testing tools, including widget tests and integration tests, to ensure your animations work as expected. Additionally, Flutter’s rich set of debugging tools, such as the DevTools suite, can help you identify and resolve any issues in your animations.
Conclusion
Custom animations are a fantastic way to elevate your Flutter apps and deliver a captivating user experience. By mastering the fundamentals of animations, understanding animation controllers, and exploring various animation widgets, you can create intricate and engaging UI interactions. Remember that practice makes perfect, so don’t hesitate to experiment with different animation techniques to find the ones that best suit your app’s style and functionality. Happy animating!
Table of Contents
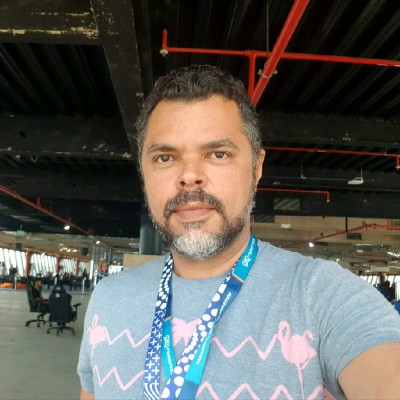
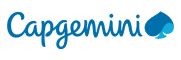