Creating Custom Widgets in Flutter: Reusable UI Components
In the world of mobile app development, creating stunning and functional user interfaces is a priority. Flutter, Google’s open-source UI software development toolkit, has gained immense popularity for its capability to craft beautiful and responsive interfaces. One of the key aspects that Flutter emphasizes is the concept of creating custom widgets – self-contained, reusable UI components that streamline development and enhance code maintainability. In this guide, we will delve into the art of crafting custom widgets in Flutter, exploring the benefits they offer, the steps to create them, and best practices to keep in mind.
Table of Contents
1. The Power of Custom Widgets:
Custom widgets are the building blocks of Flutter applications. They enable developers to encapsulate complex UI elements into reusable components, promoting modularity, reducing code duplication, and improving overall development efficiency. By creating custom widgets, you can maintain a consistent design language throughout your app, making it easier to update and scale your UI as your app evolves. Let’s explore the advantages they bring:
1.1. Modularity and Reusability:
Custom widgets embody the “Don’t Repeat Yourself” (DRY) principle by allowing you to define a UI element once and reuse it across different parts of your app. This not only saves time but also ensures consistent styling and behavior throughout the application.
1.2. Maintainability:
As your app grows, managing and updating various UI elements can become challenging. Custom widgets act as isolated entities, making it easier to troubleshoot issues, apply changes, and perform updates without affecting the rest of the application.
1.3. Abstraction of Complexity:
Complex UI components can be challenging to implement and comprehend. By encapsulating such complexity within a custom widget, you provide a higher-level abstraction to other developers, making the codebase more accessible and understandable.
2. Creating a Custom Widget:
Now that we understand the benefits, let’s dive into the process of creating a custom widget in Flutter. In this example, we’ll create a custom “GradientButton” widget that displays a button with a gradient background.
Step 1: Define the Widget Class
To create a custom widget, start by defining a new Dart class that extends the StatelessWidget or StatefulWidget class. In this case, we’ll use StatelessWidget:
dart import 'package:flutter/material.dart'; class GradientButton extends StatelessWidget { final String text; final List<Color> gradientColors; final VoidCallback onPressed; GradientButton({ required this.text, required this.gradientColors, required this.onPressed, }); @override Widget build(BuildContext context) { return ElevatedButton( onPressed: onPressed, style: ElevatedButton.styleFrom( padding: EdgeInsets.symmetric(vertical: 16), shape: RoundedRectangleBorder( borderRadius: BorderRadius.circular(8), ), primary: Colors.transparent, ), child: Ink( decoration: BoxDecoration( gradient: LinearGradient( colors: gradientColors, ), borderRadius: BorderRadius.circular(8), ), child: Container( alignment: Alignment.center, child: Text( text, style: TextStyle( color: Colors.white, fontWeight: FontWeight.bold, ), ), ), ), ); } }
In this code snippet, we’ve defined a GradientButton class that takes three required parameters: text (the button label), gradientColors (a list of colors for the gradient background), and onPressed (a callback function when the button is pressed).
Step 2: Using the Custom Widget
Once the custom widget is defined, you can easily use it within your app. Import the file where the GradientButton class is defined and include the widget in your UI tree:
dart import 'package:flutter/material.dart'; import 'gradient_button.dart'; // Import the custom widget void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Gradient Button Example', theme: ThemeData(primarySwatch: Colors.blue), home: Scaffold( appBar: AppBar(title: Text('Gradient Button Example')), body: Center( child: GradientButton( text: 'Get Started', gradientColors: [Colors.blue, Colors.green], onPressed: () { print('Button pressed!'); }, ), ), ), ); } }
In this example, we’ve imported the GradientButton widget and used it inside the Center widget in the MyApp class. The button’s properties are set through the constructor, and the onPressed callback is defined.
3. Best Practices for Custom Widgets:
While creating custom widgets can significantly improve your Flutter development process, adhering to certain best practices can further enhance their effectiveness:
3.1. Single Responsibility Principle:
Each custom widget should have a clear and focused responsibility. This makes the widget easier to understand, reuse, and maintain. If a widget becomes too complex, consider breaking it down into smaller, more specialized widgets.
3.2. Parameterization:
Design your custom widgets with parameters that allow users to customize appearance and behavior. This promotes flexibility and reusability. However, avoid excessive parameterization, as it can make the widget difficult to use.
3.3. Documentation:
Provide clear and concise documentation for your custom widgets, including information about their purpose, required parameters, and usage examples. This helps other developers (and your future self) understand and use the widgets effectively.
3.4. Testing:
Just like any other part of your codebase, custom widgets should be tested. Write unit tests to ensure that the widgets render correctly and behave as expected under various scenarios.
3.5. Folder Structure:
Organize your custom widgets into a well-defined folder structure within your project. This makes it easier to locate and manage widgets as your app grows.
Conclusion
Custom widgets are a fundamental concept in Flutter that empowers developers to build reusable UI components and improve the efficiency of the development process. By encapsulating UI elements within custom widgets, you ensure a consistent design, enhance code maintainability, and promote modularity. As you continue your journey with Flutter, mastering the art of creating custom widgets will enable you to create elegant and responsive user interfaces that leave a lasting impression on your users. Happy coding!
Table of Contents
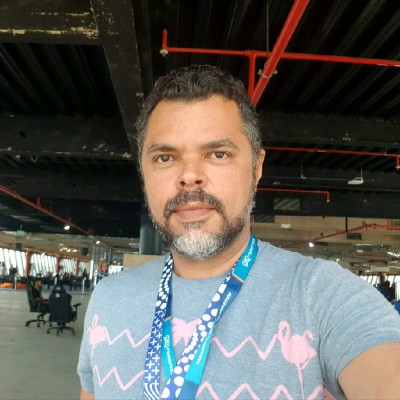
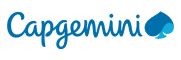