Exploring Flutter: Your Ultimate Toolkit for Cross-Platform App Development
The landscape of mobile application development has seen several transitions, from native app development (Java for Android and Objective-C/Swift for iOS) to hybrid frameworks (like React Native, Ionic, and Xamarin). Recently, Google’s Flutter has emerged as a solid choice for creating cross-platform applications. Developers, including many who seek to hire Flutter developers, are drawn to its ability to allow writing code once and deploying it on multiple platforms, thereby saving time and resources. This growing demand to hire Flutter developers attests to its popularity and effectiveness. In this blog post, we will walk you through the process of building cross-platform apps using Flutter and explore why it has become the ultimate solution in the industry.
What is Flutter?
Flutter is an open-source UI toolkit, created by Google, that allows developers to build natively compiled applications for mobile, web, and desktop platforms from a single codebase. This means you can use one programming language (Dart) and one codebase to create an application that runs on different platforms.
Flutter’s main advantages are:
– Single Codebase: Build your iOS and Android apps from a single codebase.
– Hot Reload: See your changes as soon as you save. Experience sub-second reload times, without losing state, on emulators, simulators, and hardware for iOS and Android.
– Modern, Reactive Framework: Create powerful, flexible, and expressive user interfaces.
– Rich, beautiful Widgets: Delight your users with sophisticated UI designs.
Flutter Installation & Setup
To start building with Flutter, you first need to have it set up on your local system. Here are the steps you should follow:
- System requirements: Ensure you have a compatible operating system like macOS (64-bit), Windows 7 SP1 or later (64-bit), or Linux (64-bit).
- Get the Flutter SDK: Download the stable release of the Flutter SDK from the Flutter website.
- Update your path: Unzip the file and add the `flutter/bin` directory to your path.
- Run Flutter doctor: This tool checks your system and provides a report about the status of your Flutter installation.
Dart: The Language of Flutter
Dart, a language developed by Google, is the primary language used in Flutter. Dart’s syntax might feel familiar if you’ve used a language like JavaScript or Java. It brings object-orientation, strong typing, and an easy-to-understand syntax, making it a pleasure to work with.
Flutter Architecture
Flutter’s architecture is designed to support fast development and deliver a smooth UI experience to users. Its layered architecture allows full customization, which results in incredibly fast rendering and expressive, flexible designs. The architecture comprises the Dart platform, Flutter engine, Foundation library, and Design-specific widgets.
Building a Simple Flutter App
To understand the power and simplicity of Flutter, let’s create a simple Flutter app.
- Create a new project: Run `flutter create my_app` in the terminal. This command creates a new Flutter project named `my_app`.
- Run the application: Navigate into the `my_app` directory and run `flutter run`. This will launch your app in a connected emulator or device.
- Open the main Dart file: Open `lib/main.dart` in your favorite editor and delete its contents. We’re going to start from scratch.
- Create a main function: Every app must have a main function. To create it, add the following code:
```dart void main() { runApp(MyApp()); } ```
- Create a Stateless Widget: In Flutter, almost everything is a widget, including alignment, padding, and layout. We’ll define a `MyApp` widget:
```dart class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(title: 'Flutter Demo Home Page'), ); } } ```
- Create a Stateful Widget: A Stateful widget can change over time. Let’s create a `MyHomePage` widget:
```dart class MyHomePage extends StatefulWidget { MyHomePage({Key? key, required this.title}) : super(key: key); final String title; @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { int _counter = 0; void _incrementCounter() { setState(() { _counter++; }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text(widget.title), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( 'You have pushed the button this many times:', ), Text( '$_counter', style: Theme.of(context).textTheme.headline4, ), ], ), ), floatingActionButton: FloatingActionButton( onPressed: _incrementCounter, tooltip: 'Increment', child: Icon(Icons.add), ), ); } } ```
Save the file and run `flutter run`. You will see a basic Flutter app with a button. Each time you press the button, the counter is incremented.
Final Thoughts
It’s evident that Flutter brings a lot to the table when it comes to cross-platform mobile app development. It’s a game-changer, offering a single codebase, a plethora of beautiful widgets, a reactive framework, and most importantly, performance that’s almost indistinguishable from a native app. Developers, and even businesses looking to hire Flutter developers, can leverage this tool to craft beautiful, high-performance applications in record time, making it the ultimate solution for cross-platform app development. The increasing need to hire Flutter developers in the industry is a testament to its growing acceptance. Remember, learning a new technology takes time, so keep practicing, keep building, and let Flutter’s capabilities unfold for you.
Table of Contents
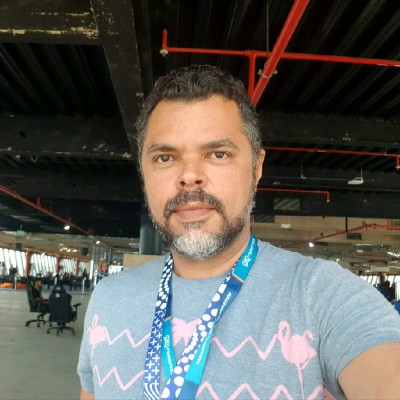
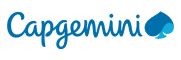