Flutter for Financial Apps: Secure Transactions and Data Handling
In today’s digital age, financial transactions are increasingly being carried out through mobile applications. With the growing popularity of smartphones and the convenience they offer, it’s no surprise that people are relying on mobile apps for their financial needs. However, this convenience comes with a significant responsibility: ensuring the security of financial transactions and sensitive data.
Table of Contents
Flutter, Google’s open-source UI software development toolkit, has gained immense popularity for building cross-platform mobile applications. In this blog, we will explore how Flutter can be used to create secure financial apps, focusing on secure transaction handling and data protection. We’ll delve into best practices, security considerations, and provide code samples to guide you in developing robust financial applications.
1. Why Flutter for Financial Apps?
Before we dive into the specifics of secure transactions and data handling, let’s briefly discuss why Flutter is an excellent choice for developing financial apps.
1.1. Cross-Platform Compatibility
Flutter allows developers to create a single codebase that runs seamlessly on both Android and iOS platforms. This is a significant advantage in the world of financial apps, where reaching a broad user base is essential.
1.2. Rich User Interface
Flutter provides a wide range of pre-designed widgets and the flexibility to create custom UI components, enabling developers to design intuitive and visually appealing financial app interfaces.
1.3. Speed and Efficiency
Financial apps often require real-time data updates and quick response times. Flutter’s hot-reload feature helps developers make changes and see the results instantly, improving development efficiency.
1.4. Strong Community Support
Flutter boasts a thriving community of developers who contribute to its growth. This ensures that you have access to a wealth of resources, plugins, and libraries to expedite development.
Now that we understand why Flutter is an excellent choice, let’s focus on ensuring the security of financial apps built with Flutter.
2. Secure Transactions
Financial apps deal with sensitive user data and transactions, making security a top priority. Here are some best practices and considerations for implementing secure transactions in your Flutter-based financial app.
2.1. Secure Communication
2.1.1. Using HTTPS
To protect data transmitted between the app and the server, always use HTTPS (HTTP Secure) for communication. Flutter’s http package provides a straightforward way to make secure HTTP requests. Here’s a simple code sample:
dart import 'package:http/http.dart' as http; Future<void> fetchData() async { final response = await http.get(Uri.parse('https://example.com/data')); if (response.statusCode == 200) { // Handle the data } else { // Handle errors } }
By using HTTPS, you encrypt the data exchanged between the app and the server, making it challenging for malicious actors to intercept or tamper with sensitive information.
2.1.2. Certificate Pinning
Certificate pinning is an extra layer of security that ensures your app communicates only with trusted servers. In Flutter, you can implement certificate pinning using the http package. Here’s a code snippet demonstrating certificate pinning:
dart import 'package:http/http.dart' as http; import 'package:pinning_headers/pinning_headers.dart'; Future<void> fetchData() async { final client = http.Client(); final request = http.Request('GET', Uri.parse('https://example.com/data')); // Add the pinned certificate's fingerprint request.headers.addAll(pinningHeaders( 'example.com', sha256Fingerprints: ['YOUR_CERTIFICATE_FINGERPRINT'], )); final response = await client.send(request); if (response.statusCode == 200) { // Handle the data } else { // Handle errors } client.close(); }
By pinning the server’s certificate, you can ensure that your app only communicates with servers possessing the correct certificate, guarding against man-in-the-middle attacks.
2.2. Authentication and Authorization
2.2.1. Implementing User Authentication
User authentication is crucial for financial apps. Ensure that only authorized users can access sensitive features and data. Firebase Authentication is a popular choice for user authentication in Flutter apps. Here’s a code snippet to get you started:
dart import 'package:firebase_auth/firebase_auth.dart'; Future<void> signIn() async { try { UserCredential userCredential = await FirebaseAuth.instance.signInWithEmailAndPassword( email: 'user@example.com', password: 'password', ); // User signed in } catch (e) { // Handle authentication errors } }
Firebase Authentication simplifies the process of integrating authentication features, including email/password, social media logins, and more.
2.2.2. Role-Based Access Control
Implement role-based access control (RBAC) to restrict users’ access based on their roles and permissions. Firebase and Flutter can be used together to implement RBAC effectively.
2.3. Data Encryption
2.3.1. Storing Sensitive Data
Financial apps often store sensitive user data, such as account numbers and transaction histories. Ensure that this data is encrypted when stored on the device. The flutter_secure_storage package provides a secure way to store sensitive data locally. Here’s how to use it:
dart import 'package:flutter_secure_storage/flutter_secure_storage.dart'; Future<void> storeData() async { final storage = FlutterSecureStorage(); await storage.write(key: 'account_number', value: '1234567890'); }
The flutter_secure_storage package stores data in an encrypted format, protecting it from unauthorized access.
2.3.2. Data Transmission Encryption
Encrypt data before sending it to the server to prevent data interception during transmission. You can use encryption libraries like crypto in Flutter to achieve this.
dart import 'package:crypto/crypto.dart'; String encryptData(String data, String key) { final keyBytes = utf8.encode(key); final plainText = utf8.encode(data); final cipher = AesGcm.with256bits(macLength: 16); final nonce = Nonce.randomBytes(12); final encryptedData = cipher.encrypt( plainText, secretKey: SecretKey(keyBytes), nonce: nonce, ); return base64Encode(nonce + encryptedData); }
This code demonstrates how to encrypt data using the AES-GCM encryption algorithm.
2.4. Regular Security Audits
2.4.1. Penetration Testing
Perform regular penetration testing to identify and address security vulnerabilities in your financial app. This involves simulating cyberattacks to uncover weaknesses that could be exploited by malicious actors.
2.4.2. Code Review
Review your codebase periodically to ensure that security best practices are being followed consistently. Consider using static analysis tools like flutter analyze to catch potential security issues.
3. Data Handling
Apart from secure transactions, financial apps must handle user data with care to protect privacy and ensure regulatory compliance. Here are some data handling best practices for Flutter-based financial apps.
3.1. Data Minimization
Collect and store the minimum amount of user data required for your app’s functionality. The less data you collect, the less risk there is of data breaches.
3.2. Data Retention
Clearly define data retention policies and inform users about them. Regularly purge data that is no longer needed, and ensure that users have control over their data.
3.3. Secure Backend
The security of your financial app isn’t limited to the client-side. Ensure that your server and backend infrastructure are also secure. Use robust authentication mechanisms and regularly update server software.
3.4. Compliance with Regulations
Depending on your app’s target audience and region, you may need to comply with data protection regulations like GDPR (General Data Protection Regulation) or CCPA (California Consumer Privacy Act). Familiarize yourself with these regulations and ensure your app adheres to their requirements.
Conclusion
Developing secure financial apps with Flutter is not only possible but also highly recommended. By following best practices for secure transactions and data handling, you can protect sensitive user information and build trust with your users. Remember that security is an ongoing process, and it’s essential to stay updated on the latest security threats and solutions.
Flutter’s cross-platform capabilities, coupled with its strong community support, make it an excellent choice for building robust financial applications. As you embark on your financial app development journey, keep security at the forefront of your priorities, and your users will thank you for it.
Table of Contents
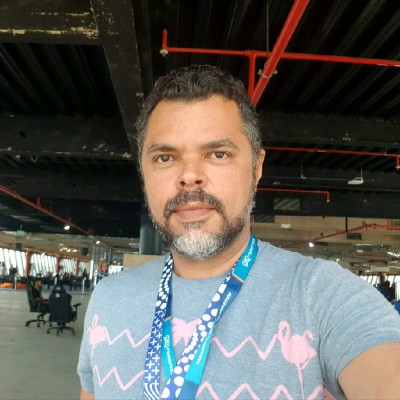
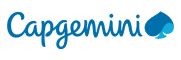