Firebase and Flutter Integration: Enhancing App Functionality with Backend Services
When it comes to building apps that are versatile, reliable, and efficient, the combination of Flutter and Firebase is a go-to choice for many developers, including those looking to hire Flutter developers. Flutter is a UI toolkit developed by Google for creating natively compiled applications for mobile, web, and desktop from a single codebase. Firebase, on the other hand, is a cloud service provider that offers a host of backend services like database, storage, authentication, analytics, and much more.
This article walks you through how you can leverage the power of Firebase services in your Flutter app, enhancing its functionality and overall user experience. This information is especially valuable if you’re looking to hire Flutter developers with expertise in Firebase. We will cover four key aspects of Firebase – Authentication, Cloud Firestore, Cloud Storage, and Cloud Messaging.
Flutter and Firebase: A Perfect Synergy
Before we delve into the tutorial, it’s essential to understand why Flutter and Firebase are such a powerful combination.
Flutter’s advantage lies in its rich set of widgets and the ability to create beautiful, high-performance apps across multiple platforms with a single codebase. Its integration with Firebase means you can easily utilize reliable backend services without the need to manage your own server.
Firebase, a product of Google, provides an array of backend services, making it a perfect fit for startups and businesses that require fast go-to-market times. It’s a serverless solution, meaning you can focus on building the frontend and leave the backend to Firebase.
Let’s now look at integrating Firebase services into a Flutter application.
1. Firebase Authentication
Firebase Authentication provides a secure authentication system that supports login with email, Google, Facebook, Twitter, and more. It ensures your users can log in seamlessly and securely into your Flutter app.
Implementation Steps:
– First, create a new Firebase project and register your app.
– Go to the Authentication section in Firebase and enable the desired sign-in method.
– Add Firebase dependencies to your Flutter project: firebase_core and firebase_auth.
```dart dependencies: firebase_core: "^1.2.1" firebase_auth: "^1.1.3" ```
– Now, you can initialize Firebase in your main.dart file:
```dart void main() async { WidgetsFlutterBinding.ensureInitialized(); await Firebase.initializeApp(); runApp(MyApp()); } ```
– To sign in with email and password, you can use the following code snippet:
```dart Future<UserCredential> signIn(String email, String password) { return FirebaseAuth.instance.signInWithEmailAndPassword( email: email, password: password, ); } ```
2. Cloud Firestore
Cloud Firestore is a flexible, scalable NoSQL cloud database for storing and syncing data. It keeps your data in sync across client apps and provides offline support.
Implementation Steps:
– Enable Firestore for your project in the Firebase console.
– Add the Firestore dependency in your Flutter project: cloud_firestore.
```dart dependencies: cloud_firestore: "^2.2.1" ```
– Use the following code to add data to Firestore:
```dart CollectionReference users = FirebaseFirestore.instance.collection('users'); Future<void> addUser() { return users .add({ 'name': 'John Doe', 'email': 'john.doe@example.com' }) .then((value) => print('User Added')) .catchError((error) => print('Failed to add user: $error')); } ```
– You can retrieve data with this code:
```dart FirebaseFirestore.instance .collection('users') .get() .then((QuerySnapshot querySnapshot) { querySnapshot.docs.forEach((doc) { print(doc["name"]); }); }); ```
3. Cloud Storage
Cloud Storage is a powerful, simple, and cost-effective object storage service, which can be used to store user-generated content like photos and videos.
Implementation Steps:
– Enable Cloud Storage for your project in the Firebase console.
– Add the Cloud Storage dependency to your Flutter project: firebase_storage.
```dart dependencies: firebase_storage: "^8.0.1" ```
– Upload a file to Firebase Storage:
```dart FirebaseStorage storage = FirebaseStorage.instance; Future<void> uploadFile(File file) async { try { await storage.ref('uploads/file-to-upload.png').putFile(file); } on FirebaseException catch (e) { // Handle any errors } } ```
– Download a file from Firebase Storage:
```dart Future<void> downloadFile() async { final String downloadURL = await storage.ref('uploads/file-to-upload.png').getDownloadURL(); // Use the downloadURL } ```
4. Firebase Cloud Messaging
Firebase Cloud Messaging (FCM) is a free, cross-platform messaging solution that lets you reliably deliver messages and notifications to iOS, Android, and the web at no cost.
Implementation Steps:
– Enable FCM for your project in the Firebase console.
– Add the FCM dependency to your Flutter project: firebase_messaging.
```dart dependencies: firebase_messaging: "^9.1.2" ```
– Request permission for notifications:
```dart FirebaseMessaging messaging = FirebaseMessaging.instance; void requestPermission() async { NotificationSettings settings = await messaging.requestPermission( alert: true, announcement: false, badge: true, carPlay: false, criticalAlert: false, provisional: false, sound: true, ); } ```
– To receive messages, use the following:
```dart FirebaseMessaging.onMessage.listen((RemoteMessage message) { print('Got a message whilst in the foreground!'); print('Message data: ${message.data}'); }); ```
Conclusion
The integration of Firebase with Flutter not only simplifies the process of app development but also gives you a robust, secure, and efficient backend infrastructure. By choosing to hire Flutter developers well-versed in Firebase, you can tap into the full potential of these powerful tools. The examples provided above offer just a glimpse into the possibilities when expertly leveraging Flutter and Firebase together. Happy coding!
Table of Contents
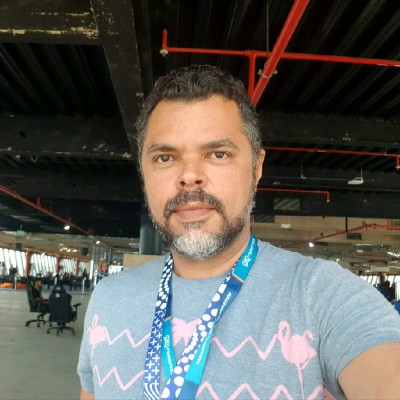
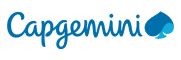