How do you handle form validation in Flutter?
Handling form validation is a crucial aspect of Flutter development to ensure data integrity and provide a seamless user experience. Flutter offers a robust set of tools for form validation, primarily through the use of the `Form` widget, `TextFormField`, and the `GlobalKey<FormState>`.
- Form Widget:
– The `Form` widget is the foundation for form validation in Flutter. It maintains the form state and provides methods to interact with and validate form fields.
- TextFormField:
– Use the `TextFormField` widget to create input fields within the form. It automatically integrates with the `Form` widget to manage the form’s state.
- GlobalKey<FormState>:
– Declare a global key of type `FormState` to uniquely identify and manage the state of the form. This key allows access to form-related functionalities.
- Validation Logic:
– Implement the `validator` property within `TextFormField` to define custom validation logic. Return `null` for valid input and an error message for invalid input.
Example:
```dart final _formKey = GlobalKey<FormState>(); String _email; ... Form( key: _formKey, child: TextFormField( decoration: InputDecoration(labelText: 'Email'), validator: (value) { if (value.isEmpty) { return 'Please enter your email.'; } else if (!isValidEmail(value)) { return 'Invalid email format.'; } return null; // Validation passed. }, onSaved: (value) { _email = value; }, ), ), ... ElevatedButton( onPressed: () { if (_formKey.currentState.validate()) { // Form is valid, proceed with data processing. _formKey.currentState.save(); // Additional logic such as API calls or data storage. } }, child: Text('Submit'), ), ```
In this example, the form includes a `TextFormField` for email input. The `validator` checks for non-empty and valid email format. The form is submitted through an `ElevatedButton`, executing further logic if the form is validated successfully.
By understanding and implementing these key points, developers can create robust and user-friendly form validation in Flutter applications.
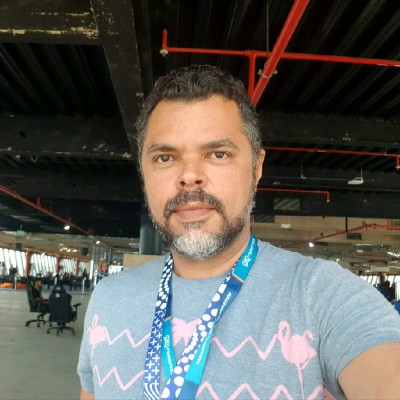
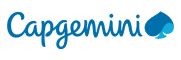