Using Flutter with GraphQL: Efficient Data Fetching in Apps
In the dynamic landscape of app development, the demand for faster and more efficient data fetching has led to the convergence of various technologies. Flutter, Google’s open-source UI software development toolkit, and GraphQL, a query language for APIs, have emerged as a powerful duo for building responsive and data-efficient applications. In this article, we’ll delve into the integration of Flutter with GraphQL and how this combination can revolutionize the way data is fetched and managed in your apps.
1. Understanding the Need for Efficient Data Fetching
In the world of app development, users’ expectations are soaring high, demanding applications that are not only visually appealing but also highly responsive. Traditional REST APIs, while effective, often lead to over-fetching or under-fetching of data, resulting in either sluggish performance or incomplete data sets. This is where GraphQL comes into play.
1.1 The Power of GraphQL
GraphQL is designed to provide a more flexible and efficient approach to data fetching compared to traditional REST APIs. With GraphQL, clients can request exactly the data they need, no more and no less, by crafting queries tailored to their requirements. This eliminates over-fetching and ensures that the application receives only the data necessary for its current context.
2. Integrating GraphQL into Flutter Apps
Flutter, with its reactive framework for building natively compiled applications, integrates seamlessly with GraphQL to create a harmonious development environment. Here’s how you can get started:
2.1 Setting Up Your Flutter Project
If you’re new to Flutter, you’ll need to set up a Flutter project first. Make sure you have the Flutter SDK installed. You can create a new Flutter project using the following command:
bash flutter create your_project_name cd your_project_name
2.2 Adding Dependencies
To begin using GraphQL in your Flutter project, you’ll need to add the necessary dependencies to your pubspec.yaml file. The most common package used is graphql_flutter, which provides widgets and classes to integrate GraphQL seamlessly into Flutter.
yaml dependencies: flutter: sdk: flutter graphql_flutter: ^4.0.0
After adding the dependencies, remember to run flutter pub get to fetch and install them.
2.3 Setting Up GraphQL Client
Next, you’ll need to set up a GraphQL client to communicate with your GraphQL server. Initialize the client in your app’s entry point (usually the main.dart file) as follows:
dart import 'package:flutter/material.dart'; import 'package:graphql_flutter/graphql_flutter.dart'; void main() { final HttpLink httpLink = HttpLink('https://your-graphql-endpoint.com'); final ValueNotifier<GraphQLClient> client = ValueNotifier( GraphQLClient( link: httpLink, cache: GraphQLCache(store: HiveStore()), ), ); runApp(MyApp(client)); } class MyApp extends StatelessWidget { final ValueNotifier<GraphQLClient> client; MyApp(this.client); @override Widget build(BuildContext context) { return GraphQLProvider( client: client, child: CacheProvider( child: MaterialApp( title: 'Flutter GraphQL Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ), ), ); } }
In this example, we’re using the HttpLink from graphql_flutter to connect to the GraphQL endpoint. You can replace ‘https://your-graphql-endpoint.com’ with the actual URL of your GraphQL server.
3. Efficient Data Fetching with GraphQL Queries
One of the core advantages of using GraphQL is its ability to fetch only the required data, minimizing unnecessary data transfers and enhancing performance. Let’s see how you can craft GraphQL queries within your Flutter app:
3.1 Defining GraphQL Queries
GraphQL queries are at the heart of efficient data fetching. Unlike REST APIs, where you often get a fixed set of data, GraphQL queries allow you to specify exactly what fields you need. Here’s an example of a GraphQL query to fetch a user’s profile:
dart const String getUserProfile = r''' query GetUserProfile($userId: ID!) { user(id: $userId) { id name email profileImage } } ''';
In this query, we’re fetching the user’s ID, name, email, and profile image. The $userId is a variable that can be provided when making the query.
3.2 Querying with the GraphQL Client
With the query defined, you can now use the GraphQL client to execute it and retrieve the desired data. Here’s how you can do it:
dart QueryOptions options = QueryOptions( document: gql(getUserProfile), variables: {'userId': '123'}, ); QueryResult result = await client.query(options); if (result.hasException) { print(result.exception.toString()); } else if (result.isLoading) { return CircularProgressIndicator(); } else { final userData = result.data['user']; // Now you can use userData for your UI components }
This code sends the defined query to the server, substitutes the $userId variable, and retrieves the user’s profile data from the response. Depending on the response state, you can handle exceptions, loading states, and utilize the fetched data.
3.3 Mutations and Fragments
GraphQL not only excels in querying data but also in performing mutations (create, update, delete operations) efficiently. Additionally, you can use fragments to define reusable sets of fields that can be included in multiple queries.
4. Leveraging GraphQL Subscriptions
Apart from queries and mutations, GraphQL offers a powerful feature called subscriptions. Subscriptions allow your app to receive real-time updates when specific data changes on the server. This is particularly useful for chat applications, live feeds, and other real-time features.
4.1 Creating a Subscription
Here’s an example of how you can create a subscription to receive real-time notifications about new messages in a chat application:
dart const String newMessageSubscription = r''' subscription NewMessage($chatId: ID!) { newMessage(chatId: $chatId) { id text sender timestamp } } '''; SubscriptionOptions options = SubscriptionOptions( document: gql(newMessageSubscription), variables: {'chatId': '456'}, ); Stream<QueryResult> stream = client.subscribe(options); stream.listen((result) { if (!result.hasException && result.data != null) { final newMessageData = result.data['newMessage']; // Handle the new message data in real-time } });
In this example, the subscription listens for new messages in a specific chat ($chatId variable). Whenever a new message is sent in that chat, the server sends the updated data, which is then processed in real-time.
Conclusion
The integration of Flutter with GraphQL brings forth a powerful synergy that greatly enhances data fetching efficiency and responsiveness in applications. By crafting precise queries, eliminating over-fetching, and utilizing real-time capabilities, developers can create apps that meet the high expectations of modern users. Whether it’s crafting tailored queries or receiving real-time updates, the combination of Flutter and GraphQL empowers developers to optimize their app’s performance and user experience.
In a world where user expectations are ever-evolving, embracing technologies like Flutter and GraphQL is not just a choice but a strategic move to deliver top-notch applications that stand out in a crowded market. As you embark on your journey to build efficient, flexible, and responsive apps, remember that the union of Flutter and GraphQL can be your most valuable asset. So why wait? Dive into the world of Flutter and GraphQL today and unlock the true potential of your app development journey. Happy coding!
Table of Contents
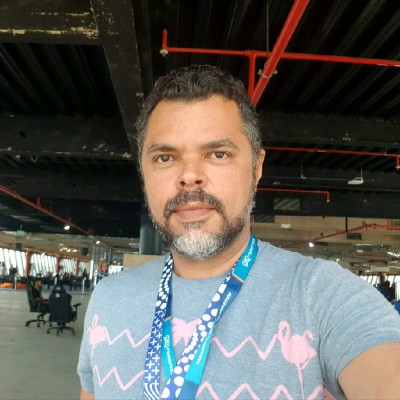
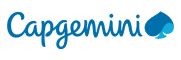