Handling User Input in Flutter: Forms and Validation
User input is a fundamental aspect of app development, enabling interaction and customization that cater to individual user needs. When it comes to building mobile applications with Flutter, a powerful and flexible UI toolkit developed by Google, handling user input efficiently is essential for creating a seamless and engaging user experience. In this guide, we’ll delve into the world of user input handling in Flutter, focusing on forms and validation techniques that ensure data integrity and user satisfaction.
Table of Contents
1. Introduction to User Input Handling
1.1. The Significance of Forms and Validation
User input comes in various forms: text, numbers, selections, and more. To effectively manage and utilize this input, Flutter provides the means to create interactive forms and implement validation processes. Forms enable structured data collection and submission, while validation ensures that the collected data is accurate, consistent, and meets specific criteria.
2. Building and Handling Forms
2.1. Creating a Basic Form
In Flutter, creating a basic form involves using the Form widget as the foundation. The Form widget holds a collection of form fields that allow users to input data. Let’s take a look at a simple example:
dart import 'package:flutter/material.dart'; class BasicFormExample extends StatefulWidget { @override _BasicFormExampleState createState() => _BasicFormExampleState(); } class _BasicFormExampleState extends State<BasicFormExample> { final _formKey = GlobalKey<FormState>(); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Basic Form Example')), body: Form( key: _formKey, child: Column( children: [ TextFormField( decoration: InputDecoration(labelText: 'Username'), validator: (value) { if (value == null || value.isEmpty) { return 'Please enter your username'; } return null; }, ), ElevatedButton( onPressed: () { if (_formKey.currentState!.validate()) { // Perform form submission } }, child: Text('Submit'), ), ], ), ), ); } }
In this example, we define a Form widget that wraps a TextFormField for the user to input their username. The validator property of the TextFormField is used to perform validation on the user’s input. If the input is empty, an error message is returned. The form submission is controlled by the ElevatedButton, and the _formKey is used to validate the form before submission.
2.2. Retrieving User Input
Once the form is submitted and validated, you can retrieve the user’s input using the TextFormField’s controller or by directly accessing the form’s data. Here’s how you can retrieve input using controllers:
dart class _BasicFormExampleState extends State<BasicFormExample> { final _formKey = GlobalKey<FormState>(); final _usernameController = TextEditingController(); @override void dispose() { _usernameController.dispose(); super.dispose(); } // Widget build method and other code... void _submitForm() { if (_formKey.currentState!.validate()) { final username = _usernameController.text; // Perform form submission with username } } @override Widget build(BuildContext context) { return Scaffold( // Scaffold code... body: Form( key: _formKey, child: Column( children: [ TextFormField( controller: _usernameController, decoration: InputDecoration(labelText: 'Username'), validator: (value) { // Validation logic... }, ), ElevatedButton( onPressed: _submitForm, child: Text('Submit'), ), ], ), ), ); } }
In this example, we’ve added a _usernameController to the state and linked it to the TextFormField. When the form is submitted, the _submitForm function retrieves the text from the controller.
2.3. Submitting and Resetting the Form
Submitting the form involves calling the validate() method on the form’s key, as demonstrated in the examples above. If the validation passes, you can proceed with the form submission logic. Additionally, you might want to reset the form after submission. To achieve this, call the reset() method on the form’s key.
dart void _submitForm() { if (_formKey.currentState!.validate()) { final username = _usernameController.text; // Perform form submission with username _formKey.currentState!.reset(); // Reset the form } }
3. Data Validation and Error Feedback
3.1. Implementing Validation Logic
Data validation ensures that the input data adheres to certain rules or constraints. Flutter provides the validator property in form fields, allowing you to define custom validation logic for each field. The validator function takes the input value as a parameter and returns an error message or null if the input is valid.
dart TextFormField( // Other properties... validator: (value) { if (value == null || value.isEmpty) { return 'This field is required'; } return null; // Input is valid }, ),
In this example, the validation function checks if the value is empty and returns an error message if it is.
3.2. Displaying Error Messages
To display validation error messages to users, Flutter provides the Text widget or other UI elements adjacent to the form field. You can conditionally render error messages based on the validation result:
dart Column( children: [ TextFormField( // Other properties... validator: (value) { // Validation logic... }, ), SizedBox(height: 8), Text( 'Error message goes here', style: TextStyle(color: Colors.red), ), ], ),
Remember to toggle the visibility of the error message based on the validation result.
3.3. Visual Feedback for Users
While error messages provide textual feedback, visual cues can enhance the user experience by highlighting validation status. For instance, changing the border color of the input field to red when there’s an error or to green when the input is valid:
dart TextFormField( // Other properties... validator: (value) { // Validation logic... }, decoration: InputDecoration( labelText: 'Username', errorBorder: OutlineInputBorder( borderSide: BorderSide(color: Colors.red), ), focusedErrorBorder: OutlineInputBorder( borderSide: BorderSide(color: Colors.red), ), enabledBorder: OutlineInputBorder( borderSide: BorderSide(color: Colors.grey), ), focusedBorder: OutlineInputBorder( borderSide: BorderSide(color: Colors.blue), ), ), ),
In this example, we customize the border colors for different validation states, providing users with instant feedback.
4. Advanced Input Fields
4.1. Working with Different Input Field Types
Flutter offers a variety of input field types tailored to specific data types and user interactions. Some common types include TextFormField, DatePicker, Slider, and Checkbox. These widgets come with their own set of properties for customization.
dart TextFormField( // Properties... keyboardType: TextInputType.emailAddress, // Other properties... ), DatePicker( // Properties... initialDate: DateTime.now(), firstDate: DateTime(2020), lastDate: DateTime(2025), // Other properties... ), Slider( // Properties... min: 0, max: 100, divisions: 5, // Other properties... ), Checkbox( // Properties... value: _isSelected, onChanged: (newValue) { setState(() { _isSelected = newValue; }); }, ),
4.2. Input Field Customization
Flutter allows extensive customization of input fields to match your app’s design. You can control various aspects such as borders, colors, fonts, and padding. Here’s an example of customizing the appearance of a TextFormField:
dart TextFormField( // Other properties... decoration: InputDecoration( labelText: 'Email', labelStyle: TextStyle(color: Colors.grey), focusedBorder: OutlineInputBorder( borderSide: BorderSide(color: Colors.blue), ), errorBorder: OutlineInputBorder( borderSide: BorderSide(color: Colors.red), ), // Other decoration properties... ), ),
4.3. Input Masks for Specialized Input
Input masks provide a structured format for user input, enforcing a specific pattern as the user types. This is useful for cases like phone numbers, credit card numbers, or dates. The flutter_masked_text package simplifies the implementation of input masks:
dart import 'package:flutter_masked_text/flutter_masked_text.dart'; // Inside your widget's class... var phoneNumberController = MaskedTextController(mask: '(000) 000-0000'); TextFormField( // Other properties... controller: phoneNumberController, keyboardType: TextInputType.phone, // Other properties... ),
5. Best Practices for Effective User Input
5.1. Keeping User Interface Intuitive
When designing forms, prioritize an intuitive user interface. Group related fields, use clear labels, and consider placeholder text to guide users. Additionally, minimize the number of required fields and avoid excessive validation requirements.
5.2. Real-time Validation
Providing real-time validation feedback enhances the user experience by catching errors early. Implement instant validation as users interact with the form fields, such as displaying error messages as soon as input becomes invalid.
5.3. Accessibility Considerations
Ensure your form is accessible to users with disabilities. Use appropriate labels and hints for screen readers, and provide contrasting colors for users with visual impairments. Test your app with accessibility tools to ensure a smooth experience for all users.
6. Putting It All Together: A Complete Example
6.1. Building a Login Screen
Let’s bring together the concepts we’ve covered to create a complete example of a login screen with user input handling and validation:
dart import 'package:flutter/material.dart'; class LoginScreen extends StatefulWidget { @override _LoginScreenState createState() => _LoginScreenState(); } class _LoginScreenState extends State<LoginScreen> { final _formKey = GlobalKey<FormState>(); final _emailController = TextEditingController(); final _passwordController = TextEditingController(); void _submitForm() { if (_formKey.currentState!.validate()) { final email = _emailController.text; final password = _passwordController.text; // Perform login with email and password } } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Login')), body: Padding( padding: EdgeInsets.all(16.0), child: Form( key: _formKey, child: Column( children: [ TextFormField( controller: _emailController, decoration: InputDecoration(labelText: 'Email'), keyboardType: TextInputType.emailAddress, validator: (value) { if (value == null || value.isEmpty) { return 'Please enter your email'; } return null; }, ), SizedBox(height: 16), TextFormField( controller: _passwordController, decoration: InputDecoration(labelText: 'Password'), obscureText: true, validator: (value) { if (value == null || value.isEmpty) { return 'Please enter your password'; } return null; }, ), SizedBox(height: 24), ElevatedButton( onPressed: _submitForm, child: Text('Login'), ), ], ), ), ), ); } } void main() { runApp(MaterialApp(home: LoginScreen())); }
In this example, we’ve created a simple login screen with email and password input fields. The form performs validation on both fields, and the user’s input is retrieved when the form is submitted.
6.2. Enhancing the User Experience
To enhance the user experience further, you can implement additional features such as password visibility toggling, “Remember Me” functionality, and social media authentication buttons. These elements contribute to a more user-friendly and engaging login process.
Conclusion
Efficiently handling user input is a crucial aspect of building successful mobile applications, and Flutter provides a powerful toolkit for achieving this. By creating forms, implementing validation logic, and utilizing advanced input field types, you can create a seamless user experience that encourages engagement and data accuracy. Remember to follow best practices for intuitive UI design, real-time validation, and accessibility considerations to ensure your app caters to a diverse user base. With the knowledge gained from this guide, you’re well-equipped to tackle user input handling in Flutter and create remarkable applications that cater to user needs effectively.
Table of Contents
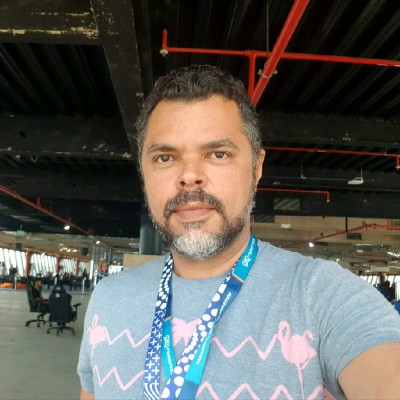
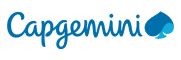