How do you handle HTTP requests in a Flutter app?
Handling HTTP requests in a Flutter app is crucial for interacting with web services and fetching data from APIs. Flutter provides the `http` package to simplify this process. Here’s a comprehensive answer with key points and an example:
Importing the Package: Begin by adding the `http` package to your `pubspec.yaml` file and running `flutter pub get` to install it.
```yaml dependencies: http: ^0.14.0 ```
Making HTTP Requests:
– Use the `http` package’s `get()` method to perform a simple GET request.
– The package supports other HTTP methods like POST, PUT, DELETE, etc.
Handling Responses:
– HTTP responses are asynchronous, so utilize the `async/await` syntax or the `then()` method to handle responses.
– Extract relevant data from the response, considering the data format (JSON, XML, etc.).
Error Handling:
– Implement error handling to manage network issues or server errors.
– Utilize `try-catch` blocks for synchronous errors and the `catchError` method for asynchronous errors.
Example:
```dart import 'package:http/http.dart' as http; void fetchData() async { final url = Uri.parse('https://api.example.com/data'); try { final response = await http.get(url); if (response.statusCode == 200) { // Successful response print('Data: ${response.body}'); } else { // Handle errors print('Error: ${response.statusCode}'); } } catch (e) { // Handle network errors print('Error: $e'); } } ```
Explanation:
- Import the `http` package.
- Define the URL you want to request.
- Use `http.get()` to initiate the GET request.
- Check the response’s status code to determine success or failure.
- Handle the response data or errors accordingly.
This example showcases the basics of making an HTTP request in Flutter, including error handling and response processing.
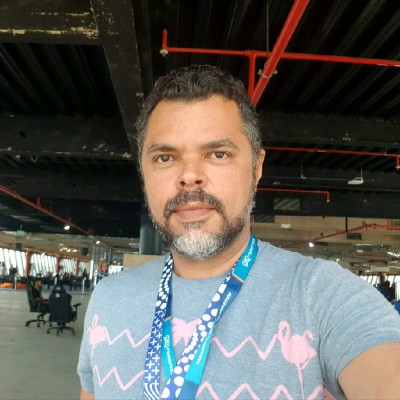
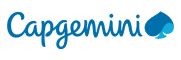