Implementing Push Notifications in Flutter: Engaging Users
In the fast-paced digital landscape of today, user engagement is a key factor in the success of any mobile application. Push notifications have proven to be a powerful tool for enhancing user engagement by providing timely and relevant information, updates, and reminders. If you’re a Flutter developer looking to captivate your users and keep them hooked to your app, implementing push notifications is a crucial step. In this guide, we will walk you through the process of integrating push notifications into your Flutter app and share some best practices to ensure your notifications hit the mark.
Table of Contents
1. Introduction to Push Notifications
1.1. What are Push Notifications?
Push notifications are short messages that are sent from a server to a user’s device with the aim of delivering relevant information, updates, or alerts. These notifications appear on the user’s device even if they are not actively using the app. They are a direct line of communication to the user, providing valuable interactions and encouraging them to return to the app.
1.2. Importance of Push Notifications in User Engagement
User engagement plays a pivotal role in the success of mobile applications. Push notifications serve as a bridge to maintain this engagement by sending users updates about new features, personalized recommendations, time-sensitive alerts, and more. Well-crafted notifications can re-engage dormant users and encourage active users to explore the app further.
2. Setting Up Push Notifications
2.1. Creating a Firebase Project
To get started with implementing push notifications in your Flutter app, you’ll need a Firebase project. Firebase provides a robust cloud-based platform that includes a variety of features, one of which is Firebase Cloud Messaging (FCM) for handling push notifications.
2.2. Head to the Firebase Console.
- Create a new project or use an existing one.
- Follow the on-screen instructions to set up your project.
- Adding Your App to the Firebase Project
- Once you have a Firebase project, you need to add your Flutter app to it.
- In the Firebase Console, select your project.
- Click on “Add app” and choose the platform (Android or iOS) for your app.
- Follow the steps to register your app, providing necessary details like the app’s package name (Android) or bundle ID (iOS).
2.3. Obtaining Server API Key and Sender ID
To enable communication between your server (or the Firebase server) and your Flutter app, you’ll need to obtain the Server API Key and Sender ID.
- In the Firebase Console, navigate to your project and then to Project Settings.
- In the Cloud Messaging tab, you’ll find the Server Key under the “Project credentials” section. This key is essential for authenticating your requests to FCM.
- The Sender ID is also available in the Cloud Messaging tab. It’s a unique numerical value associated with your Firebase project.
3. Integrating Dependencies
Flutter provides several packages that simplify the process of adding push notifications to your app. The two main packages are firebase_messaging for handling FCM and flutter_local_notifications for displaying local notifications.
3.1. Adding Required Dependencies in pubspec.yaml
Open your pubspec.yaml file and add the following dependencies:
yaml dependencies: flutter: sdk: flutter firebase_core: ^1.10.0 firebase_messaging: ^10.0.0 flutter_local_notifications: ^10.4.0
3.2. Syncing Dependencies
After adding the dependencies, run flutter pub get in your terminal to sync them with your project.
4. Requesting Notification Permissions
Before sending push notifications, you need to request permission from the user to display notifications on their device.
4.1. Handling Permission Requests
In your app’s entry point (usually the main.dart file), add the following code to request notification permissions:
dart import 'package:firebase_core/firebase_core.dart'; import 'package:flutter/material.dart'; import 'package:firebase_messaging/firebase_messaging.dart'; Future<void> main() async { WidgetsFlutterBinding.ensureInitialized(); await Firebase.initializeApp(); FirebaseMessaging.instance.requestPermission(); runApp(MyApp()); }
4.2. Handling Permission Responses
To handle the user’s response to the permission request, you can set up a callback in your main widget:
dart FirebaseMessaging.onMessagePermissionDenied.listen((event) { // Handle when the user denies permission }); FirebaseMessaging.onMessage.listen((RemoteMessage message) { // Handle incoming messages }); FirebaseMessaging.onMessageOpenedApp.listen((RemoteMessage message) { // Handle when the app is in the background and the user taps on a notification });
5. Handling Push Notifications
5.1. Handling Notifications in Foreground
When the app is in the foreground, you can handle incoming notifications using the onMessage callback:
dart FirebaseMessaging.onMessage.listen((RemoteMessage message) { // Handle the notification print("Notification received in foreground: ${message.notification!.body}"); });
5.2. Handling Notifications in Background
When the app is in the background, you can handle notification taps using the onMessageOpenedApp callback:
dart FirebaseMessaging.onMessageOpenedApp.listen((RemoteMessage message) { // Handle the notification tap print("Notification tapped in background: ${message.notification!.body}"); // Navigate to a specific screen if needed // Navigator.of(context).pushNamed('/details'); });
5.3. Navigating to a Specific Screen on Notification Click
If you want to navigate to a specific screen when the user taps on a notification, you can achieve this by modifying the callback in the onMessageOpenedApp listener:
dart FirebaseMessaging.onMessageOpenedApp.listen((RemoteMessage message) { // Handle the notification tap print("Notification tapped in background: ${message.notification!.body}"); // Navigate to a specific screen Navigator.of(context).pushNamed('/details'); });
6. Displaying Custom Push Notifications
While the default notification layout provided by the operating system is functional, customizing the notification layout can enhance the user experience and align with your app’s branding.
6.1. Designing Custom Notification Layouts
Flutter’s flutter_local_notifications package allows you to create custom notification layouts:
dart import 'package:flutter_local_notifications/flutter_local_notifications.dart'; Future<void> _showCustomNotification() async { var androidDetails = AndroidNotificationDetails( 'channelId', 'Custom Notification', 'A custom notification layout', styleInformation: BigTextStyleInformation(''), ); var platformChannelSpecifics = NotificationDetails(android: androidDetails); await flutterLocalNotificationsPlugin.show( 0, 'Custom Notification Title', 'Custom Notification Body', platformChannelSpecifics, ); }
6.2. Adding Custom Data to Notifications
You can include custom data in your notifications to provide additional context to your users:
dart var notificationData = RemoteNotification( title: 'Custom Data Notification', body: 'Notification with custom data', data: {'screen': 'details', 'id': '123'}, ); var androidPlatformChannelSpecifics = AndroidNotificationDetails( 'channelId', 'Custom Data Notification', 'Notification with custom data', ); var platformChannelSpecifics = NotificationDetails(android: androidPlatformChannelSpecifics); await flutterLocalNotificationsPlugin.show( 0, notificationData.title, notificationData.body, platformChannelSpecifics, payload: jsonEncode(notificationData.data), );
7. Sending Push Notifications
7.1. Using Firebase Cloud Messaging (FCM) Console
The Firebase Cloud Messaging (FCM) console provides an intuitive interface to send notifications manually. Follow these steps to send a notification:
- In the Firebase Console, select your project.
- Go to the “Cloud Messaging” tab.
- Click on “New notification” and fill in the notification details.
- Choose the target audience and click “Next.”
- Review the notification and click “Publish.”
7.2. Sending Notifications Programmatically
To send notifications programmatically, you’ll need to use the FCM API. Here’s a basic example using the http package:
dart import 'package:http/http.dart' as http; Future<void> sendPushNotification() async { final serverKey = 'YOUR_SERVER_KEY'; final url = Uri.parse('https://fcm.googleapis.com/fcm/send'); final headers = { 'Content-Type': 'application/json', 'Authorization': 'key=$serverKey', }; final payload = { 'notification': { 'title': 'Programmatic Notification', 'body': 'This is a programmatically sent notification.', }, 'to': 'DEVICE_TOKEN', }; final response = await http.post(url, headers: headers, body: jsonEncode(payload)); if (response.statusCode == 200) { print('Notification sent successfully'); } else { print('Failed to send notification'); } }
8. Best Practices for Effective Push Notifications
8.1. Personalization and Relevance
Craft personalized notifications based on user behavior and preferences. Users are more likely to engage with notifications that offer value to them.
8.2. Opt-out and Frequency Options
Provide users with the option to opt out of notifications or customize their notification preferences. Respect their choices to maintain a positive user experience.
8.2. A/B Testing of Notification Content
Experiment with different notification content to understand what resonates best with your users. A/B testing can help you refine your notification strategy over time.
Conclusion
In conclusion, push notifications are a vital tool for boosting user engagement in your Flutter app. By following these steps to integrate and effectively utilize push notifications, you can keep your users informed, engaged, and excited about your app’s offerings. Remember to strike a balance between timely updates and respecting user preferences to create a winning notification strategy. Happy coding and engaging your users!
Remember, user engagement is a continuous process, and push notifications can be a powerful addition to your arsenal. Utilize them wisely to keep your users hooked and your app thriving. Happy coding!
Table of Contents
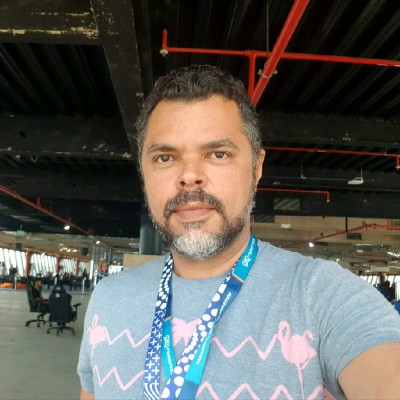
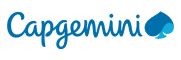