Flutter and Maps: Integrating Geolocation Services in Apps
In today’s fast-paced world, location-based services have become an integral part of mobile applications. Whether it’s finding the nearest restaurant, tracking a delivery, or simply exploring a new city, integrating geolocation services into your app can greatly enhance the user experience. Flutter, Google’s UI toolkit for building natively compiled applications for mobile, web, and desktop from a single codebase, provides a seamless way to integrate interactive maps and geolocation services into your app.
Table of Contents
1. Understanding Geolocation Services
Geolocation services allow your app to access the device’s location information. This opens up a world of possibilities, from displaying the user’s current location on a map to providing turn-by-turn directions. Flutter simplifies this process by providing plugins that streamline the integration of geolocation services.
2. Setting Up Your Flutter Project
Before diving into the integration process, make sure you have Flutter installed on your machine. You can set up a new Flutter project using the following commands:
bash flutter create geolocation_app cd geolocation_app
This will create a new Flutter project named “geolocation_app.”
3. Integrating Geolocation Services
Flutter offers several plugins that make it easy to integrate geolocation services into your app. One of the most popular plugins is the geolocator plugin, which provides a straightforward way to retrieve the device’s location.
3.1. Installing the Geolocator Plugin
To install the geolocator plugin, add the following dependency to your pubspec.yaml file:
yaml dependencies: flutter: sdk: flutter geolocator: ^7.7.0
After adding the dependency, run the following command to fetch the package:
bash flutter pub get
3.2. Retrieving the User’s Location
To retrieve the user’s location, import the geolocator package in your Dart code:
dart import 'package:geolocator/geolocator.dart';
You can then use the following code to fetch the user’s current location:
dart void getLocation() async { Position position = await Geolocator.getCurrentPosition( desiredAccuracy: LocationAccuracy.high, ); print('Latitude: ${position.latitude}, Longitude: ${position.longitude}'); }
In this code, the getCurrentPosition method is used to fetch the user’s current position with a desired accuracy level. You can adjust the LocationAccuracy enum to match your app’s requirements.
4. Integrating Interactive Maps
Displaying the user’s location on a map provides valuable context and enhances the overall user experience. Flutter offers the google_maps_flutter plugin, which allows you to embed Google Maps directly into your app.
4.1. Installing the Google Maps Plugin
To install the google_maps_flutter plugin, add the following dependency to your pubspec.yaml file:
yaml dependencies: flutter: sdk: flutter google_maps_flutter: ^2.2.0
Don’t forget to run the command flutter pub get to fetch the package.
4.2. Displaying a Map
To display a map in your Flutter app, you’ll need to obtain an API key from the Google Cloud Console. Follow the instructions provided by Google to obtain your API key.
Once you have the API key, you can use it to configure the GoogleMap widget:
dart import 'package:flutter/material.dart'; import 'package:google_maps_flutter/google_maps_flutter.dart'; class MapScreen extends StatefulWidget { @override _MapScreenState createState() => _MapScreenState(); } class _MapScreenState extends State<MapScreen> { GoogleMapController? mapController; final LatLng initialPosition = LatLng(37.7749, -122.4194); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Map Integration'), ), body: GoogleMap( onMapCreated: (controller) { mapController = controller; }, initialCameraPosition: CameraPosition( target: initialPosition, zoom: 14.0, ), ), ); } }
In this code, the GoogleMap widget is used to display the map. The initialCameraPosition parameter sets the initial position and zoom level of the map. Remember to replace the coordinates with your desired location.
4.3. Adding Markers to the Map
Markers provide visual cues on the map, indicating points of interest or specific locations. You can add markers to the map using the Marker class:
dart Marker( markerId: MarkerId('marker_1'), position: LatLng(37.7749, -122.4194), infoWindow: InfoWindow(title: 'San Francisco'), )
5. Real-world Applications
Integrating geolocation services and interactive maps opens the door to a wide range of real-world applications:
5.1. Navigation Apps
Navigation apps help users find the best route to their destination. By integrating geolocation services and interactive maps, you can provide turn-by-turn directions, real-time traffic updates, and alternative routes.
5.2. Location-based Social Networks
Location-based social networks allow users to connect with others in their vicinity. Geolocation services enable features such as finding nearby friends, checking in at locations, and discovering local events.
5.3. On-demand Services
Apps offering on-demand services, such as food delivery or ride-sharing, rely heavily on geolocation services to track orders, drivers, and delivery locations in real time.
5.4. Travel and Exploration Apps
Travel apps can enhance the user experience by providing interactive maps that highlight points of interest, recommend nearby attractions, and offer immersive city guides.
Conclusion
Integrating geolocation services and interactive maps into your Flutter app can significantly improve user engagement and functionality. Whether you’re building a navigation app, a social network, or an on-demand service, harnessing the power of geolocation opens up a world of possibilities. With the geolocator and google_maps_flutter plugins, Flutter simplifies the integration process, allowing you to create feature-rich location-aware apps with ease. So go ahead and enhance your app’s user experience by incorporating geolocation services and interactive maps today. Happy coding!
Table of Contents
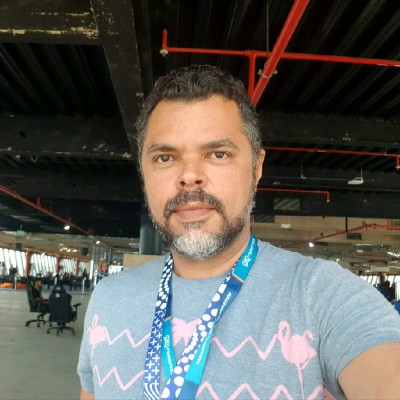
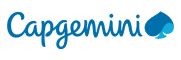